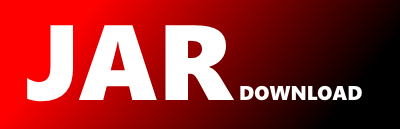
com.loocme.sys.util.PatternUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import lombok.extern.slf4j.Slf4j;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* 正则表达式匹配帮助类
*
* @author loocme
*/
@Slf4j
public class PatternUtil
{
/**
* 使用默认方式判断匹配字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @return 是否有匹配的字符串
*/
public static boolean isMatch(String str, String regex)
{
return isMatch(str, regex, 0);
}
/**
* 使用高级方式判断匹配字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @param flags Pattern类中的常量组合
* @return 是否有匹配的字符串
*/
public static boolean isMatch(String str, String regex, int flags)
{
if (null == str)
{
return false;
}
Pattern p = 0 == flags ? Pattern.compile(regex) : Pattern.compile(regex, flags);
Matcher m = p.matcher(str);
return m.find();
}
/**
* 使用默认方式判断不匹配字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @return 是否不匹配字符串
*/
public static boolean isNotMatch(String str, String regex)
{
return !isMatch(str, regex, 0);
}
/**
* 使用高级方式判断不匹配字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @param flags Pattern类中的常量组合
* @return 是否不匹配字符串
*/
public static boolean isNotMatch(String str, String regex, int flags)
{
return !isMatch(str, regex, flags);
}
/**
* 通过正则表达式获取所有匹配的字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @return 匹配成功的字符串集合
*/
public static List getMatches(String str, String regex)
{
return getMatches(str, regex, 0);
}
/**
* 通过正则表达式获取所有匹配的字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @param flags Pattern类中的常量组合
* @return 匹配成功的字符串集合
*/
public static List getMatches(String str, String regex, int flags)
{
if (null == str)
{
return null;
}
List list = new ArrayList();
String[] tempArr = null;
Pattern p = 0 == flags ? Pattern.compile(regex) : Pattern.compile(regex, flags);
Matcher m = p.matcher(str);
while (m.find())
{
int tempLength = m.groupCount() + 1;
tempArr = new String[tempLength];
for (int i = 0; i < tempLength; i++)
{
tempArr[i] = m.group(i);
}
list.add(tempArr);
}
return list;
}
/**
* 通过正则表达式获取所有匹配的字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @param flags Pattern类中的常量组合
* @param groupNum 设定需要获取的group
* @return 匹配成功的字符串集合
*/
public static List getMatches(String str, String regex, int flags, int groupNum)
{
if (null == str)
{
return null;
}
List list = new ArrayList();
Pattern p = 0 == flags ? Pattern.compile(regex) : Pattern.compile(regex, flags);
Matcher m = p.matcher(str);
while (m.find())
{
if (groupNum > m.groupCount())
{
log.error(String.format("获取的group数量(%s)超出了正则表达式中定义的group数量(%s)", groupNum, m.groupCount()));
}
list.add(m.group(groupNum));
}
return list;
}
/**
* 通过正则表达式获取所有匹配的字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @return 第一次匹配成功的字符串
*/
public static String[] getMatch(String str, String regex)
{
return getMatch(str, regex, 0);
}
/**
* 通过正则表达式获取所有匹配的字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @param flags Pattern类中的常量组合
* @return 第一次匹配成功的字符串
*/
public static String[] getMatch(String str, String regex, int flags)
{
if (null == str)
{
return null;
}
String[] tempArr = null;
Pattern p = 0 == flags ? Pattern.compile(regex) : Pattern.compile(regex, flags);
Matcher m = p.matcher(str);
if (m.find())
{
int tempLength = m.groupCount() + 1;
tempArr = new String[tempLength];
for (int i = 0; i < tempLength; i++)
{
tempArr[i] = m.group(i);
}
}
return tempArr;
}
/**
* 通过正则表达式获取所有匹配的字符串
*
* @param str 进行匹配的字符串
* @param regex 正则表达式
* @param flags Pattern类中的常量组合
* @param groupNum 设定需要获取的group
* @return 第一次匹配成功的字符串
*/
public static String getMatch(String str, String regex, int flags, int groupNum)
{
if (null == str)
{
return null;
}
Pattern p = 0 == flags ? Pattern.compile(regex) : Pattern.compile(regex, flags);
Matcher m = p.matcher(str);
if (m.find())
{
if (groupNum > m.groupCount())
{
log.error(String.format("获取的group数量(%s)超出了正则表达式中定义的group数量(%s)", groupNum, m.groupCount()));
}
return m.group(groupNum);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy