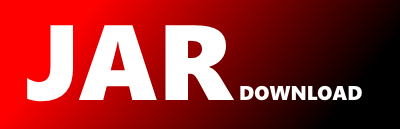
com.loocme.sys.util.PostUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import java.io.File;
import java.util.Map;
import java.util.regex.Pattern;
import com.loocme.sys.exception.HttpConnectionException;
/**
* post请求使用帮助类
*
* 会根据请求的url判断使用http还是https
*
* @author loocme
*/
public class PostUtil
{
private static final String REG_IS_HTTPS = "^https:.+$";
private static final String REG_IS_HTTP = "^http:.+$";
/**
* post请求数据
*
* @param url 请求地址
* @param content 请求参数
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, String content) throws HttpConnectionException
{
return request(url, null, null, content);
}
/**
* post请求数据
*
* @param url 请求地址
* @param headerMap 请求中需指定的头信息
* @param content 请求参数
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, Map headerMap, String content) throws HttpConnectionException
{
return request(url, null, headerMap, content);
}
/**
* post请求数据
*
* @param url 请求地址
* @param encoding 字符编码
* @param headerMap 请求中需指定的头信息
* @param content 请求参数
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, String encoding, Map headerMap, String content) throws HttpConnectionException
{
if (PatternUtil.isMatch(url, REG_IS_HTTPS, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.post(url, encoding, headerMap, content);
}
else if (PatternUtil.isMatch(url, REG_IS_HTTP, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.post(url, encoding, headerMap, content);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
public static String request(String url, Map requestParams) throws HttpConnectionException
{
return request(url, null, 0, null, requestParams);
}
public static String request(String url, String encoding, Map requestParams) throws HttpConnectionException
{
return request(url, encoding, 0, null, requestParams);
}
/**
* post请求数据
*
* @param url 请求地址
* @param encoding 字符编码
* @param headerMap 请求中需指定的头信息
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, String encoding, int timeout, Map headerMap, Map requestParams) throws HttpConnectionException
{
if (PatternUtil.isMatch(url, REG_IS_HTTPS, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.post(url, encoding, timeout, headerMap, requestParams);
}
else if (PatternUtil.isMatch(url, REG_IS_HTTP, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.post(url, encoding, timeout, headerMap, requestParams);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
public static String requestContent(String url, String encoding, String contentType, String content) throws HttpConnectionException
{
return requestContent(url, encoding, null, contentType, content);
}
public static String requestContent(String url, String contentType, String content) throws HttpConnectionException
{
return requestContent(url, "UTF-8", null, contentType, content);
}
public static String requestContent(String url, String contentType, int timeout,
String content) throws HttpConnectionException
{
return requestContent(url, "UTF-8", timeout, null, contentType, content);
}
public static String requestContent(String url, String encoding,
Map headerMap, String contentType, String content)
throws HttpConnectionException
{
return requestContent(url, encoding, 0, headerMap, contentType, content);
}
/**
* post请求数据
*
* @param url 请求地址
* @param encoding 字符编码
* @param headerMap 请求中需指定的头信息
* @param content 请求参数
* @return 响应信息
* @throws HttpConnectionException
*/
public static String requestContent(String url, String encoding, int timeout, Map headerMap, String contentType, String content) throws HttpConnectionException
{
if (PatternUtil.isMatch(url, REG_IS_HTTPS, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.postContent(url, encoding, timeout, headerMap, contentType, content);
}
else if (PatternUtil.isMatch(url, REG_IS_HTTP, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.postContent(url, encoding, timeout, headerMap, contentType, content);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
public static String requestBytes(String url, byte[] body)
throws HttpConnectionException
{
return requestBytes(url, null, null, null, body);
}
public static String requestBytes(String url, String contentType, byte[] body)
throws HttpConnectionException
{
return requestBytes(url, null, null, contentType, body);
}
public static String requestBytes(String url, Map headerMap, Map queryParams, String contentType, byte[] body)
throws HttpConnectionException
{
if (PatternUtil.isMatch(url, "^https:.+$", Pattern.CASE_INSENSITIVE))
{
throw new HttpConnectionException(1001, "不是http请求");
}
else if (PatternUtil
.isMatch(url, "^http:.+$", Pattern.CASE_INSENSITIVE))
{
return HttpUtil.postBytes(url, headerMap, queryParams, contentType, body);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
public static byte[] requestProtobuf(String url, byte[] body) throws HttpConnectionException
{
return requestProtobuf(url, null, null, 0, null, body);
}
public static byte[] requestProtobuf(String url, Map headerMap, Map queryParams, int timeout, String contentType, byte[] body) throws HttpConnectionException
{
if (PatternUtil.isMatch(url, "^https:.+$", Pattern.CASE_INSENSITIVE))
{
throw new HttpConnectionException(1001, "不是http请求");
}
else if (PatternUtil.isMatch(url, "^http:.+$", Pattern.CASE_INSENSITIVE))
{
return HttpUtil.postProtobuf(url, timeout, headerMap, queryParams, contentType, body);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
public static void download(String url, File file) throws HttpConnectionException
{
download(url, null, "", file);
}
public static void download(String url, String encoding, String content, File file) throws HttpConnectionException
{
if (PatternUtil.isMatch(url, REG_IS_HTTPS, Pattern.CASE_INSENSITIVE))
{
HttpUtil.download(url, encoding, content, file);
}
else if (PatternUtil.isMatch(url, REG_IS_HTTP, Pattern.CASE_INSENSITIVE))
{
HttpUtil.download(url, encoding, content, file);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
}