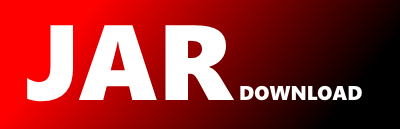
com.loocme.sys.util.PropUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import lombok.extern.slf4j.Slf4j;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.util.Properties;
/**
* Properties文件读写帮助类
*
* @author loocme
*/
@Slf4j
public class PropUtil
{
private static final String PREFIX_LOCAL_LINUX_FILEPATH = "/home/";
private static final String REG_LOCAL_WINDOWS_FILEPATH = "^[a-zA-z]:";
/**
* 通过文件路径获取properties文件
*
* @param filePath 文件路径
* @return properties文件
*/
public static Properties getPropFile(String filePath)
{
Properties prop = new Properties();
if (PatternUtil.isMatch(filePath, REG_LOCAL_WINDOWS_FILEPATH) || filePath.startsWith(PREFIX_LOCAL_LINUX_FILEPATH))
{
File file = new File(filePath);
try
{
prop.load(new FileInputStream(file));
}
catch (FileNotFoundException e)
{
log.error("", e);
}
catch (IOException e)
{
log.error("", e);
}
}
else
{
try
{
prop.load(new InputStreamReader(PropUtil.class.getResourceAsStream(filePath), "UTF-8"));
}
catch (IOException e)
{
log.error("", e);
}
}
return prop;
}
/**
* 通过文件路径获取properties文件
*
* @param file 文件句柄
* @return properties文件
*/
public static Properties getPropFile(File file)
{
Properties prop = new Properties();
try
{
prop.load(new InputStreamReader(new FileInputStream(file), "UTF-8"));
}
catch (FileNotFoundException e)
{
log.error("", e);
}
catch (IOException e)
{
log.error("", e);
}
return prop;
}
public static void savePropFile(String filePath, Properties prop)
{
try
{
OutputStream outputStream = new FileOutputStream(filePath);
prop.store(outputStream, "");
outputStream.close();
}
catch (FileNotFoundException e)
{
log.error("", e);
}
catch (IOException e)
{
log.error("", e);
}
}
/**
* 获取properties文件中的string属性
*
* @param prop properties文件
* @param key 获取的key
* @return 获取的value
*/
public static String getString(Properties prop, String key)
{
if (prop == null || StringUtil.isNull(key))
{
return null;
}
return prop.getProperty(key);
}
public static void setString(Properties prop, String key, String value)
{
if (null != prop && StringUtil.isNotNull(key))
{
prop.setProperty(key, value);
}
}
/**
* 获取properties文件中的string属性
*
* @param filePath properties文件路径
* @param key 获取的key
* @return 获取的value
*/
public static String getString(String filePath, String key)
{
return getString(getPropFile(filePath), key);
}
public static void setString(String filePath, String key, String value)
{
setString(getPropFile(filePath), key, value);
}
/**
* 获取properties文件中的int属性
*
* @param prop properties文件
* @param key 获取的key
* @return 获取的value
*/
public static int getInteger(Properties prop, String key)
{
return StringUtil.getInteger(getString(prop, key));
}
/**
* 获取properties文件中的int属性
*
* @param filePath properties文件路径
* @param key 获取的key
* @return 获取的value
*/
public static int getInteger(String filePath, String key)
{
return StringUtil.getInteger(getString(filePath, key));
}
/**
* 获取properties文件中的long属性
*
* @param prop properties文件
* @param key 获取的key
* @return 获取的value
*/
public static long getLong(Properties prop, String key)
{
return StringUtil.getLong(getString(prop, key));
}
/**
* 获取properties文件中的long属性
*
* @param filePath properties文件路径
* @param key 获取的key
* @return 获取的value
*/
public static long getLong(String filePath, String key)
{
return StringUtil.getLong(getString(filePath, key));
}
/**
* 获取properties文件中的double属性
*
* @param prop properties文件
* @param key 获取的key
* @return 获取的value
*/
public static double getDouble(Properties prop, String key)
{
return StringUtil.getDouble(getString(prop, key));
}
/**
* 获取properties文件中的double属性
*
* @param filePath properties文件路径
* @param key 获取的key
* @return 获取的value
*/
public static double getDouble(String filePath, String key)
{
return StringUtil.getDouble(getString(filePath, key));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy