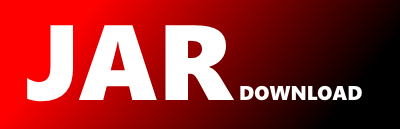
com.loocme.sys.util.ReflectUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.loocme.sys.classloader.AppClassLoader;
import lombok.extern.slf4j.Slf4j;
/**
* java 反射帮助类
*
* @author loocme
*/
@Slf4j
public class ReflectUtil
{
/**
* 通过Class创建一个实例对象
*
* @param 泛型
* @param clz 需要被创建对象的class
* @return 创建的实例对象
*/
public static T newInstance(Class clz)
{
if (null == clz)
{
return null;
}
try
{
return clz.getDeclaredConstructor().newInstance();
}
catch (InstantiationException | IllegalAccessException | NoSuchMethodException | InvocationTargetException e)
{
log.error("", e);
}
return null;
}
/**
* 通过Class路径创建一个实例对象
*
* @param clzName 需要被创建对象的class路径
* @return 创建的实例对象
*/
public static Object newInstance(String clzName)
{
Class> clz = getClass(clzName);
if (null == clz)
{
return null;
}
try
{
return clz.getDeclaredConstructor().newInstance();
}
catch (InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchMethodException e)
{
log.error("", e);
}
return null;
}
public static Class> getClass(String className)
{
Class> clz = null;
try
{
clz = AppClassLoader.getInstance().loadClass(className);
}
catch (ClassNotFoundException e)
{
log.error("", e);
}
return clz;
}
public static Object invokeMethod(Object obj, String methodName, Object... args)
{
Class> clz = obj.getClass();
try
{
Class>[] paramClzs = new Class>[args.length];
if (null != args && 0 < args.length)
{
for (int i = 0; i < args.length; i++)
{
if (null == args[i])
{
paramClzs[i] = Object.class;
}
else
{
paramClzs[i] = args[i].getClass();
}
}
}
Method method = clz.getDeclaredMethod(methodName, paramClzs);
return invokeMethod(obj, method, args);
}
catch (SecurityException | NoSuchMethodException | IllegalArgumentException e)
{
log.error("", e);
}
return null;
}
public static Object invokeMethod(Object obj, Method method, Object... args)
{
try
{
return method.invoke(obj, args);
}
catch (SecurityException | InvocationTargetException | IllegalAccessException |
IllegalArgumentException e)
{
log.error("", e);
}
return null;
}
/**
* 通过反射使用对象的set方法给属性设值
*
* @param 泛型
* @param obj 需要被设值的对象
* @param attrName 需要被设值的属性
* @param attrValue 需要被设置的值
*/
public static void setValue(T obj, String attrName, Class> valueClz, Object attrValue)
{
String methodName = "set" + upperFirstLetter(attrName);
Class> searchType = obj.getClass();
Method method = null;
while (!Object.class.equals(searchType) && null != searchType)
{
try
{
method = searchType.getDeclaredMethod(methodName, valueClz);
}
catch (NoSuchMethodException e)
{
log.debug("search upper class method. ", e);
searchType = searchType.getSuperclass();
continue;
}
catch (SecurityException e)
{
log.error("", e);
}
break;
}
try
{
if (null != method)
{
method.invoke(obj, attrValue);
}
}
catch (SecurityException | IllegalArgumentException | IllegalAccessException | InvocationTargetException e)
{
log.error("", e);
}
}
public static void setValue(T obj, String attrName, Object attrValue)
{
Class> clz = obj.getClass();
try
{
Field field = clz.getDeclaredField(attrName);
field.setAccessible(true);
field.set(obj, attrValue);
}
catch (SecurityException | IllegalArgumentException | IllegalAccessException | NoSuchFieldException e)
{
log.error("", e);
}
}
public static Method getObjSetMethod(Class> clz, String attrName, Class> valueClz)
{
String methodName = "set" + upperFirstLetter(attrName);
Method method = null;
while (!Object.class.equals(clz) && null != clz)
{
try
{
method = clz.getDeclaredMethod(methodName, valueClz);
}
catch (NoSuchMethodException e)
{
log.debug("search upper class method. ", e);
clz = clz.getSuperclass();
continue;
}
catch (SecurityException e)
{
log.error("", e);
}
break;
}
return method;
}
public static void setValueByMethod(T obj, Method method, Object attrValue)
{
try
{
if (null != method)
{
method.invoke(obj, attrValue);
}
}
catch (SecurityException | IllegalArgumentException | IllegalAccessException | InvocationTargetException e)
{
log.error("", e);
}
}
/**
* 通过反射获取对象实例中的特定属性的属性值(需设定返回值类型,且与obj中属性类型一致)
*
* @param 泛型
* @param obj 对象实例
* @param attrName 获取值得属性名
* @param attrValueClz 获取值的类型
* @return 获取的值
*/
@SuppressWarnings("unchecked")
public static T getValue(Object obj, String attrName, Class attrValueClz)
{
Object attrValue = getValue(obj, attrName);
if (null == attrValue)
{
return null;
}
if (attrValue.getClass() == attrValueClz)
{
return (T) attrValue;
}
else
{
log.error(String.format("反射获取属性值类型(%s)与需获取的类型(%s)不一致", attrValue.getClass(), attrValueClz));
return null;
}
}
/**
* 通过反射获取对象实例中特定属性的值
*
* @param obj 对象实例
* @param attrName 需要获取的属性的属性名
* @return 属性的值
*/
public static Object getValue(Object obj, String attrName)
{
Object attrValue = null;
String methodName = null;
Class> searchType = obj.getClass();
methodName = "get" + upperFirstLetter(attrName);
Method method = null;
while (!Object.class.equals(searchType) && null != searchType)
{
try
{
method = searchType.getDeclaredMethod(methodName);
}
catch (NoSuchMethodException e)
{
log.debug("search upper class method. ", e);
searchType = searchType.getSuperclass();
continue;
}
catch (SecurityException e)
{
log.error("", e);
}
break;
}
try
{
if (null != method)
{
attrValue = method.invoke(obj);
}
}
catch (SecurityException | IllegalArgumentException | IllegalAccessException | InvocationTargetException e)
{
log.error("", e);
}
return attrValue;
}
/**
* 获取对象实例中特定修饰符的属性(不包含继承的属性)
*
* @param clz 类对象
* @param modifier 修饰符
* @return field集合
*/
public static List getDeclaredFields(Class> clz, int modifier)
{
List fieldList = new ArrayList();
Class> searchType = clz;
while (!Object.class.equals(searchType) && searchType != null)
{
Field[] fields = searchType.getDeclaredFields();
if (ArrayUtil.isNotNull(fields))
{
for (int i = 0; i < fields.length; i++)
{
Field field = fields[i];
if ((field.getModifiers() | modifier) > 0)
{
fieldList.add(field);
}
}
}
// 获取所有父类,接着遍历父类中的元素。
searchType = searchType.getSuperclass();
}
return fieldList;
}
/**
* 获取对象实例中特定修饰符的属性
*
* @param clz 类class
* @param modifier 修饰符
* @return field集合
*/
public static List getFields(Class> clz, int modifier)
{
List fieldList = new ArrayList();
Class> searchType = clz;
while (!Object.class.equals(searchType) && searchType != null)
{
Field[] fields = searchType.getFields();
if (ArrayUtil.isNotNull(fields))
{
for (int i = 0; i < fields.length; i++)
{
Field field = fields[i];
if ((field.getModifiers() | modifier) > 0)
{
fieldList.add(field);
}
}
}
// 获取所有父类,接着遍历父类中的元素。
searchType = searchType.getSuperclass();
}
return fieldList;
}
/**
* 获取对象实例中特定修饰符的属性
*
* @param clz 类class
* @param modifier 修饰符
* @return field集合
*/
public static List getMethods(Class> clz, int modifier)
{
List methodList = new ArrayList();
Method[] methods = clz.getMethods();
if (ArrayUtil.isNotNull(methods))
{
for (int i = 0; i < methods.length; i++)
{
Method method = methods[i];
if ((method.getModifiers() | modifier) > 0)
{
methodList.add(method);
}
}
}
return methodList;
}
/**
* 通过反射获取对象实例中所有的private属性的属性名集合
*
* @param clz 类class
* @return private属性名集合
*/
public static List getAttributeNames(Class> clz)
{
List nameList = new ArrayList();
Class> searchType = clz;
while (!Object.class.equals(searchType) && searchType != null)
{
Field[] fields = searchType.getDeclaredFields();
if (ArrayUtil.isNotNull(fields))
{
for (int i = 0; i < fields.length; i++)
{
Field field = fields[i];
if (field.getModifiers() == Modifier.PRIVATE || field.getModifiers() == Modifier.PROTECTED)
{
nameList.add(field.getName());
}
}
}
// 获取所有父类,接着遍历父类中的元素。
searchType = searchType.getSuperclass();
}
return nameList;
}
/**
* 将obj对象实例转换为键值对的 map集合
*
* @param obj 对象实例
* @return 键值对集合
*/
public static Map objToMap(Object obj)
{
Map map = new HashMap(16);
List attrNameList = getAttributeNames(obj.getClass());
if (ListUtil.isNotNull(attrNameList))
{
for (int i = 0; i < attrNameList.size(); i++)
{
String attrName = attrNameList.get(i);
map.put(attrName, getValue(obj, attrName));
}
}
return map;
}
/**
* 将字符串的首字母转换为大写
*
* @param attrName 字符串
* @return 转换后的字符串
*/
private static String upperFirstLetter(String attrName)
{
if (Character.isUpperCase(attrName.charAt(0)))
{
return attrName;
}
else
{
StringBuilder sb = new StringBuilder(attrName);
sb.setCharAt(0, Character.toUpperCase(sb.charAt(0)));
return sb.toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy