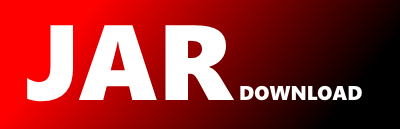
com.loocme.sys.util.SystemUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import com.loocme.sys.entities.LinuxCommandResult;
import lombok.extern.slf4j.Slf4j;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.lang.management.ManagementFactory;
import java.net.*;
import java.util.Arrays;
import java.util.regex.Pattern;
/**
* 操作系统相关使用帮助类
*
* @author loocme
*
*/
@Slf4j
public class SystemUtil
{
public static final boolean IS_WINDOWS;
public static final boolean IS_LINUX;
public static final boolean IS_OTHER_SYSTEM;
private static final String CURRENT_PID;
private static final String HOST_IP;
private static final String REG_OS_IS_WINDOWS = "^.*windows.*$";
private static final String REG_OS_IS_LINUX = "^.*(linux|unix).*$";
private static String realPath = SystemUtil.class.getResource("/")
.toString();
static
{
String osName = System.getProperty("os.name");
if (PatternUtil.isMatch(osName, REG_OS_IS_WINDOWS,
Pattern.CASE_INSENSITIVE))
{
IS_WINDOWS = true;
IS_LINUX = false;
IS_OTHER_SYSTEM = false;
}
else if (PatternUtil.isMatch(osName, REG_OS_IS_LINUX,
Pattern.CASE_INSENSITIVE))
{
IS_WINDOWS = false;
IS_LINUX = true;
IS_OTHER_SYSTEM = false;
}
else
{
IS_WINDOWS = false;
IS_LINUX = false;
IS_OTHER_SYSTEM = true;
}
// 获取当前pid
String name = ManagementFactory.getRuntimeMXBean().getName();
CURRENT_PID = name.split("@")[0];
HOST_IP = loadHostIp();
}
/**
* 获取src编译后的绝对路径
*
* @return 路径
*/
public static String getClassesPath()
{
if (IS_WINDOWS)
{
return realPath.substring(6);
}
else if (IS_LINUX)
{
return realPath.substring(5);
}
else
{
return realPath.substring(5);
}
}
public static String getRealFilePath(String file)
{
URL fileURL = SystemUtil.class.getResource(file);
if (null == fileURL)
return null;
String filePath = fileURL.getFile();
String jarPath = PatternUtil.getMatch(filePath, "^file:(.*/)[^/]+\\.jar!.*$", Pattern.CASE_INSENSITIVE, 1);
if (null != jarPath && !"".equals(jarPath))
{
// 在jar包中
return jarPath + file;
}
else
{
// 不在jar包中
return filePath;
}
}
/**
* 获取c静态库的后缀
*
* @return 后缀
*/
public static String getCLibSuffix()
{
if (IS_WINDOWS)
{
return ".lib";
}
else if (IS_LINUX)
{
return ".so";
}
else
{
return ".so";
}
}
/**
* 获取c动态库的后缀
*
* @return 后缀
*/
public static String getCDllSuffix()
{
if (IS_WINDOWS)
{
return ".dll";
}
else if (IS_LINUX)
{
return ".so";
}
else
{
return ".so";
}
}
public static String getCurrentPid()
{
return CURRENT_PID;
}
public static String getHostIp()
{
return HOST_IP;
}
private static String loadHostIp()
{
try
{
InetAddress ia = InetAddress.getLocalHost();
return ia.getHostAddress();
}
catch (UnknownHostException e)
{
log.error("", e);
}
return null;
}
public static String simpleExecuteCommand(String command)
{
return simpleExecuteCommand(new String[] {"sh", "-c", command});
}
public static String simpleExecuteCommand(String[] command)
{
LinuxCommandResult result = executeCommand(command);
return result.getResults().size() >= 1?result.getResults().get(0):"";
}
public static LinuxCommandResult executeCommand(String command)
{
return executeCommand(new String[] {"sh", "-c", command});
}
public static LinuxCommandResult executeCommand(String[] command)
{
LinuxCommandResult commandResult = new LinuxCommandResult();
Runtime rt = Runtime.getRuntime();
try
{
log.debug("[execute command] " + Arrays.toString(command));
Process p = rt.exec(command);
BufferedReader br = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
int index = 0;
while ((line = br.readLine()) != null)
{
commandResult.getResults().add(line);
}
index = 0;
BufferedReader errorBuffer = new BufferedReader(new InputStreamReader(p.getErrorStream()));
while ((line = errorBuffer.readLine()) != null)
{
commandResult.getError().append(line).append("\n");
}
p.waitFor();
commandResult.setExitValue(p.exitValue());
}
catch (InterruptedException e)
{
commandResult.setExitValue(-1);
commandResult.getError().append(e.getMessage());
}
catch (IOException e)
{
commandResult.setExitValue(-1);
commandResult.getError().append(e.getMessage());
}
return commandResult;
}
public static boolean isHostConnectable(String host, int port)
{
Socket socket = new Socket();
try
{
socket.setSoTimeout(3000);
socket.connect(new InetSocketAddress(host, port));
}
catch (IOException e)
{
return false;
}
finally
{
try
{
socket.close();
}
catch (IOException e)
{
}
}
return true;
}
public static String getBoardId()
{
if (!SystemUtil.IS_LINUX)
{
return "";
}
LinuxCommandResult result = SystemUtil.executeCommand("dmidecode -t baseboard | grep 'Serial Number'");
if (0 != result.getExitValue() || 0 != result.getError().length() || result.getResults().size() < 1)
{
return "";
}
String id = PatternUtil.getMatch(result.getResults().get(0), "^.*Serial Number: (.+)\\s*$", Pattern.CASE_INSENSITIVE, 1);
return null == id? "":id;
}
public static String getCpuId()
{
if (!SystemUtil.IS_LINUX)
{
return "";
}
LinuxCommandResult result = SystemUtil.executeCommand("dmidecode -t processor | grep 'ID'");
if (0 != result.getExitValue() || 0 != result.getError().length() || result.getResults().size() < 1)
{
return "";
}
String id = PatternUtil.getMatch(result.getResults().get(0), "^.*ID: (.+)\\s*$", Pattern.CASE_INSENSITIVE, 1);
return null == id? "":id;
}
public static String getSystemId()
{
if (!SystemUtil.IS_LINUX)
{
return "";
}
LinuxCommandResult result = SystemUtil.executeCommand("dmidecode -t system | grep 'UUID'");
if (0 != result.getExitValue() || 0 != result.getError().length() || result.getResults().size() < 1)
{
return "";
}
String id = PatternUtil.getMatch(result.getResults().get(0), "^.*UUID: (.+)\\s*$", Pattern.CASE_INSENSITIVE, 1);
return null == id? "":id;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy