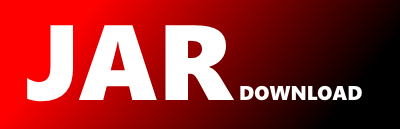
com.loocme.sys.util.XmlUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.List;
import lombok.extern.slf4j.Slf4j;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.OutputFormat;
import org.dom4j.io.SAXReader;
import org.dom4j.io.XMLWriter;
/**
* @author liuchi
*/
@Slf4j
public class XmlUtil
{
public static Document getXmlDocument(File file)
{
Document document = null;
try
{
SAXReader saxReader = new SAXReader();
document = saxReader.read(file);
}
catch (DocumentException e)
{
log.error("", e);
}
return document;
}
public static Element getElementById(Element ele, String id)
{
return ele.elementByID(id);
}
@SuppressWarnings("unchecked")
public static List getElementsByTagName(Element ele, String tagName)
{
return ele.elements(tagName);
}
public static String getString(Element ele, String attrName)
{
return ele.attributeValue(attrName);
}
public static boolean getBoolean(Element ele, String attrName)
{
return StringUtil.getBoolean(ele.attributeValue(attrName));
}
public static int getInteger(Element ele, String attrName)
{
return StringUtil.getInteger(ele.attributeValue(attrName));
}
public static void writeXml(Document document, File xmlFile)
{
writeXml(document, xmlFile, "UTF-8");
}
public static void writeXml(Document document, File xmlFile, String xmlEncoding)
{
if (null == document)
{
return;
}
OutputFormat format = OutputFormat.createPrettyPrint();
format.setEncoding(xmlEncoding);
try
{
XMLWriter xmlWriter = new XMLWriter(new FileOutputStream(xmlFile), format);
xmlWriter.write(document);
xmlWriter.close();
}
catch (IOException e)
{
log.error("", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy