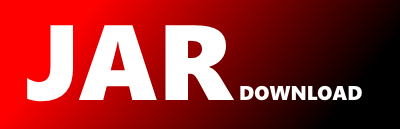
com.lordofthejars.nosqlunit.couchdb.CouchDbAssertion Maven / Gradle / Ivy
package com.lordofthejars.nosqlunit.couchdb;
import static com.lordofthejars.nosqlunit.util.DeepEquals.deepEquals;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.JsonProcessingException;
import org.codehaus.jackson.map.JsonMappingException;
import org.codehaus.jackson.map.ObjectMapper;
import org.ektorp.CouchDbConnector;
import com.lordofthejars.nosqlunit.core.FailureHandler;
public class CouchDbAssertion {
private static final String _REV = "_rev";
private static final String _ID = "_id";
private static final ObjectMapper OBJECT_MAPPER = new ObjectMapper();
private CouchDbAssertion() {
super();
}
public static final void strictAssertEquals(InputStream expectedData, CouchDbConnector couchDb) {
try {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy