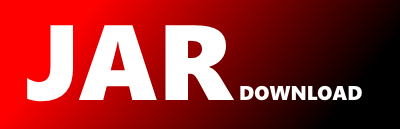
com.lordofthejars.nosqlunit.couchdb.DataLoader Maven / Gradle / Ivy
package com.lordofthejars.nosqlunit.couchdb;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import org.codehaus.jackson.JsonParseException;
import org.codehaus.jackson.JsonProcessingException;
import org.codehaus.jackson.map.JsonMappingException;
import org.codehaus.jackson.map.ObjectMapper;
import org.ektorp.CouchDbConnector;
public class DataLoader {
private static final ObjectMapper MAPPER = new ObjectMapper();
public static final String ROOT_ELEMENT = "data";
private CouchDbConnector connector;
public DataLoader(CouchDbConnector connector) {
this.connector = connector;
}
public void load(InputStream dataScript) {
try {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy