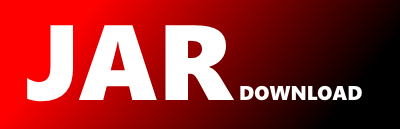
lowentry.ue4.classes.ThreadSleeper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
A Java library for the Low Entry UE4 plugins.
package lowentry.ue4.classes;
/**
* Makes it easier to sleep and wakeup threads.
*
* PS: Everything in this class is thread-safe.
*/
public class ThreadSleeper
{
public final Object object = new Object();
/**
* Sleeps until wakeup has been called.
*/
public void sleep()
{
try
{
synchronized(object)
{
object.wait();
}
}
catch(Throwable e)
{
}
}
/**
* Sleeps for the given ms, or until wakeup has been called.
*/
public void sleep(long ms)
{
try
{
synchronized(object)
{
object.wait(ms);
}
}
catch(Throwable e)
{
}
}
/**
* Sleeps for the given ms and nanos, or until wakeup has been called.
*/
public void sleep(long ms, int nanos)
{
try
{
synchronized(object)
{
object.wait(ms, nanos);
}
}
catch(Throwable e)
{
}
}
/**
* Wakes up every sleep.
*/
public void wakeup()
{
try
{
synchronized(object)
{
object.notifyAll();
}
}
catch(Throwable e)
{
}
}
/**
* Wakes up a single sleep.
*/
public void wakeupOnlyOne()
{
try
{
synchronized(object)
{
object.notify();
}
}
catch(Throwable e)
{
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy