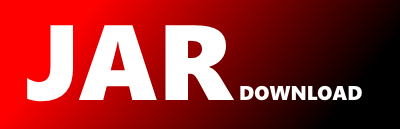
com.lucadev.coinmarketcap.api.CoinListingsFetcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of coinmarketcap-api Show documentation
Show all versions of coinmarketcap-api Show documentation
A REST API client specifically developed to connect to the CoinMarketCap API.
The newest version!
package com.lucadev.coinmarketcap.api;
import com.lucadev.coinmarketcap.model.CoinListingList;
import com.lucadev.coinmarketcap.model.CoinListingsApiResponse;
import java.util.concurrent.TimeUnit;
/**
* Fetches coin listings
*
* @author Luca Camphuisen
* @since 6-5-18
*/
public class CoinListingsFetcher implements Ticker {
/**
* Expiry of cache in seconds
*/
public static final long CACHE_EXPIRY_TIME_DEFAULT = 600;
/**
* Always fetch new
*/
public static final long CACHE_EXPIRY_TIME_NO_CACHE = -1;
private final ApiConnector apiConnector;
private CoinListingsApiResponse cachedResponse;
private long cacheExpiryTimeout = CACHE_EXPIRY_TIME_DEFAULT;
public CoinListingsFetcher() {
apiConnector = new ApiConnector().path("/listings");
}
@Override
public CoinListingsApiResponse getApiResponse() {
if (isCacheExpired()) {
cachedResponse = apiConnector.getApiResponse(CoinListingsApiResponse.class);
}
return cachedResponse;
}
/**
* Check if cache is expired.
*
* @return true when cache is expired.
*/
private boolean isCacheExpired() {
//No cache available so return true to fetch
if (cachedResponse == null) {
return true;
}
//If cache is disabled
if (cacheExpiryTimeout <= CACHE_EXPIRY_TIME_NO_CACHE) {
return true;
}
long currentUnix = System.currentTimeMillis();
//Timestamp when cache should expire
long expiryUnix = currentUnix
+ TimeUnit.SECONDS.convert(cacheExpiryTimeout, TimeUnit.MILLISECONDS);
if (currentUnix < expiryUnix) {
return true;
}
return false;
}
@Override
public CoinListingList get() {
return getApiResponse().getData();
}
/**
* After how many time units should we refresh our cache
*
* @param cacheExpiryTimeout
* @param timeUnit
*/
public CoinListingsFetcher cacheExpiryTimeout(long cacheExpiryTimeout, TimeUnit timeUnit) {
this.cacheExpiryTimeout = timeUnit.convert(cacheExpiryTimeout, TimeUnit.SECONDS);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy