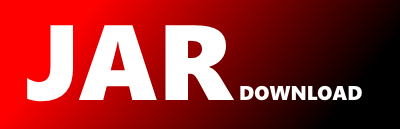
com.github.threadcontext.httpasyncclient.ContextAsyncResponseConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of httpasyncclient-thread-context Show documentation
Show all versions of httpasyncclient-thread-context Show documentation
httpasyncclient-thread-context
package com.github.threadcontext.httpasyncclient;
import com.github.threadcontext.Context;
import java.io.IOException;
import org.apache.http.HttpException;
import org.apache.http.HttpResponse;
import org.apache.http.nio.ContentDecoder;
import org.apache.http.nio.IOControl;
import org.apache.http.nio.protocol.HttpAsyncResponseConsumer;
import org.apache.http.protocol.HttpContext;
public class ContextAsyncResponseConsumer implements HttpAsyncResponseConsumer {
private final HttpAsyncResponseConsumer delegate;
private final Context context;
public ContextAsyncResponseConsumer(HttpAsyncResponseConsumer delegate, Context context) {
this.delegate = delegate;
this.context = context;
}
public void responseReceived(HttpResponse response) throws IOException, HttpException {
context.supplyException2(() -> {
delegate.responseReceived(response);
return null;
});
}
public void consumeContent(ContentDecoder decoder, IOControl ioctrl) throws IOException {
context.supplyException1(() -> {
delegate.consumeContent(decoder, ioctrl);
return null;
});
}
public void responseCompleted(HttpContext context) {
this.context.run(() -> delegate.responseCompleted(context));
}
public void failed(Exception ex) {
context.run(() -> delegate.failed(ex));
}
public Exception getException() {
return context.supply(delegate::getException);
}
public T getResult() {
return context.supply(delegate::getResult);
}
public boolean isDone() {
return context.supply(delegate::isDone);
}
public void close() throws IOException {
context.supplyException1(() -> {
delegate.close();
return null;
});
}
public boolean cancel() {
return context.supply(delegate::cancel);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy