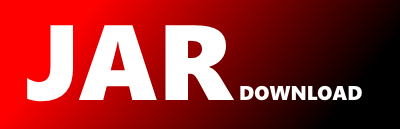
seleniumtestinglib.WebElement.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-testing-library Show documentation
Show all versions of selenium-testing-library Show documentation
Selenium locators that resemble the Testing Library (testing-library.com)
The newest version!
package seleniumtestinglib
import org.openqa.selenium.By
import org.openqa.selenium.By.id
import org.openqa.selenium.WebElement
import org.openqa.selenium.remote.RemoteWebDriver
import org.openqa.selenium.remote.RemoteWebElement
import org.openqa.selenium.support.ui.Select
val WebElement.value: Any?
get() {
if (tagName == "input" && getAttribute("type") == "number")
return getAttribute("value")?.toIntOrNull()
if (tagName == "select" && Select(this).isMultiple) {
return Select(this).allSelectedOptions.map { it.getAttribute("value") }
}
return getAttribute("value")
}
val WebElement.displayValue: Any?
get() = if (tagName == "select")
Select(this).let {
when {
it.isMultiple -> it.allSelectedOptions.map(WebElement::getText)
else -> it.firstSelectedOption.text
}
} else getAttribute("value")
val WebElement.formValues: Map
get() {
require(tagName == "form" || tagName == "fieldset") { "this should be a form or a fieldset but it's a $tagName" }
@Suppress("UNCHECKED_CAST")
return (wrappedDriver.executeScript("return arguments[0].elements", this) as List)
.groupBy { it.getAttribute("name") }
.filterKeys(String::isNotBlank)
.mapValues { (_, elements) ->
when (elements.first().tagName) {
"input" -> when (elements.first().getAttribute("type")) {
"checkbox" -> when {
elements.size > 1 -> elements.filter { it.isChecked }.map { it.getAttribute("value") }
else -> elements.first().isChecked
}
"radio" -> elements.firstOrNull { it.isChecked }?.value
else -> elements.first().value
}
else -> elements.first().value
}
}
}
fun WebElement.hasFormValues(vararg values: Pair): Boolean =
values.toMap().all { formValues[it.key] == it.value }
private fun WebElement.hasStyle(css: Map): Boolean {
val expectedCss = css.mapKeys { it.key.normalizeCssProp() }
val existingCss = expectedCss.keys
.associateWith(::getCssValue)
return expectedCss == existingCss
}
fun WebElement.hasStyle(css: String): Boolean =
hasStyle(css.split(";")
.filter(String::isNotBlank)
.associate {
it.split(":").map(String::trim)
.let { it.first() to it.last() }
})
fun WebElement.hasStyle(vararg css: Pair) = hasStyle(css.toMap())
private fun String.normalizeCssProp() =
"(?<=[a-zA-Z])[A-Z]".toRegex().replace(this) { "-${it.value}" }.lowercase()
val WebElement.isChecked: Boolean
get() {
if (tagName == "input" && getAttribute("type") in setOf("radio", "checkbox"))
return isSelected
if (ariaRole in setOf("checkbox", "radio", "switch"))
return getAttribute("aria-checked").toBoolean()
throw IllegalArgumentException("invalid aria role: $ariaRole")
}
val WebElement.isDisabled: Boolean
get() = isEnabled.not()
val WebElement.isFocused: Boolean
get() = equals(wrappedDriver.switchTo().activeElement())
val WebElement.isRequired
get() = ((tagName == "input") and (getAttribute("type") in setOf("file", "password", "date"))
or (ariaRole?.lowercase() in setOf(
"textbox",
"checkbox",
"radio",
"email",
"spinbutton",
"combobox",
"listbox",
))) and (getAttribute("required") in setOf(
"",
"true"
)) or (getAttribute("aria-required") in setOf("", "true"))
val WebElement.isValid
get() = when (tagName) {
"form" -> wrappedDriver.executeScript("return arguments[0].checkValidity()", this) as Boolean
else -> wrappedDriver.executeScript(
"return arguments[0].getAttribute('aria-invalid')",
this
) in setOf(null, "false")
}
val WebElement.isInvalid
get() = isValid.not()
val WebElement.isVisible
get() = isDisplayed
val WebElement.isPartiallyChecked: Boolean
get() {
require(ariaRole == "checkbox")
return wrappedDriver.executeScript(
"return arguments[0].indeterminate || arguments[0].ariaChecked == 'mixed'", this
) as Boolean
}
val WebElement.files: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy