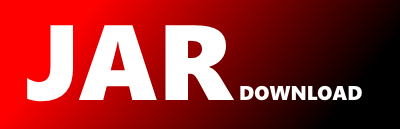
com.lukaspradel.steamapi.data.json.appnews.Newsitem Maven / Gradle / Ivy
package com.lukaspradel.steamapi.data.json.appnews;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.Generated;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"author",
"contents",
"date",
"feedlabel",
"feedname",
"gid",
"is_external_url",
"title",
"url"
})
public class Newsitem {
/**
*
*/
@JsonProperty("author")
private String author;
/**
*
*/
@JsonProperty("contents")
private String contents;
/**
*
*/
@JsonProperty("date")
private Integer date;
/**
*
*/
@JsonProperty("feedlabel")
private String feedlabel;
/**
*
*/
@JsonProperty("feedname")
private String feedname;
/**
*
*/
@JsonProperty("gid")
private String gid;
/**
*
*/
@JsonProperty("is_external_url")
private Boolean isExternalUrl;
/**
*
*/
@JsonProperty("title")
private String title;
/**
*
*/
@JsonProperty("url")
private String url;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
*
* @return
* The author
*/
@JsonProperty("author")
public String getAuthor() {
return author;
}
/**
*
* @param author
* The author
*/
@JsonProperty("author")
public void setAuthor(String author) {
this.author = author;
}
public Newsitem withAuthor(String author) {
this.author = author;
return this;
}
/**
*
* @return
* The contents
*/
@JsonProperty("contents")
public String getContents() {
return contents;
}
/**
*
* @param contents
* The contents
*/
@JsonProperty("contents")
public void setContents(String contents) {
this.contents = contents;
}
public Newsitem withContents(String contents) {
this.contents = contents;
return this;
}
/**
*
* @return
* The date
*/
@JsonProperty("date")
public Integer getDate() {
return date;
}
/**
*
* @param date
* The date
*/
@JsonProperty("date")
public void setDate(Integer date) {
this.date = date;
}
public Newsitem withDate(Integer date) {
this.date = date;
return this;
}
/**
*
* @return
* The feedlabel
*/
@JsonProperty("feedlabel")
public String getFeedlabel() {
return feedlabel;
}
/**
*
* @param feedlabel
* The feedlabel
*/
@JsonProperty("feedlabel")
public void setFeedlabel(String feedlabel) {
this.feedlabel = feedlabel;
}
public Newsitem withFeedlabel(String feedlabel) {
this.feedlabel = feedlabel;
return this;
}
/**
*
* @return
* The feedname
*/
@JsonProperty("feedname")
public String getFeedname() {
return feedname;
}
/**
*
* @param feedname
* The feedname
*/
@JsonProperty("feedname")
public void setFeedname(String feedname) {
this.feedname = feedname;
}
public Newsitem withFeedname(String feedname) {
this.feedname = feedname;
return this;
}
/**
*
* @return
* The gid
*/
@JsonProperty("gid")
public String getGid() {
return gid;
}
/**
*
* @param gid
* The gid
*/
@JsonProperty("gid")
public void setGid(String gid) {
this.gid = gid;
}
public Newsitem withGid(String gid) {
this.gid = gid;
return this;
}
/**
*
* @return
* The isExternalUrl
*/
@JsonProperty("is_external_url")
public Boolean getIsExternalUrl() {
return isExternalUrl;
}
/**
*
* @param isExternalUrl
* The is_external_url
*/
@JsonProperty("is_external_url")
public void setIsExternalUrl(Boolean isExternalUrl) {
this.isExternalUrl = isExternalUrl;
}
public Newsitem withIsExternalUrl(Boolean isExternalUrl) {
this.isExternalUrl = isExternalUrl;
return this;
}
/**
*
* @return
* The title
*/
@JsonProperty("title")
public String getTitle() {
return title;
}
/**
*
* @param title
* The title
*/
@JsonProperty("title")
public void setTitle(String title) {
this.title = title;
}
public Newsitem withTitle(String title) {
this.title = title;
return this;
}
/**
*
* @return
* The url
*/
@JsonProperty("url")
public String getUrl() {
return url;
}
/**
*
* @param url
* The url
*/
@JsonProperty("url")
public void setUrl(String url) {
this.url = url;
}
public Newsitem withUrl(String url) {
this.url = url;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Newsitem withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(author).append(contents).append(date).append(feedlabel).append(feedname).append(gid).append(isExternalUrl).append(title).append(url).append(additionalProperties).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof Newsitem) == false) {
return false;
}
Newsitem rhs = ((Newsitem) other);
return new EqualsBuilder().append(author, rhs.author).append(contents, rhs.contents).append(date, rhs.date).append(feedlabel, rhs.feedlabel).append(feedname, rhs.feedname).append(gid, rhs.gid).append(isExternalUrl, rhs.isExternalUrl).append(title, rhs.title).append(url, rhs.url).append(additionalProperties, rhs.additionalProperties).isEquals();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy