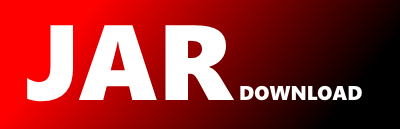
com.lukaspradel.steamapi.data.json.playersummaries.Player Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of steam-web-api Show documentation
Show all versions of steam-web-api Show documentation
A library to provide access to the data available from Valve's Steam API
package com.lukaspradel.steamapi.data.json.playersummaries;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.Generated;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"avatar",
"avatarfull",
"avatarmedium",
"communityvisibilitystate",
"lastlogoff",
"loccityid",
"loccountrycode",
"locstatecode",
"personaname",
"personastate",
"personastateflags",
"primaryclanid",
"profilestate",
"profileurl",
"realname",
"steamid",
"timecreated"
})
public class Player {
/**
*
*/
@JsonProperty("avatar")
private String avatar;
/**
*
*/
@JsonProperty("avatarfull")
private String avatarfull;
/**
*
*/
@JsonProperty("avatarmedium")
private String avatarmedium;
/**
*
*/
@JsonProperty("communityvisibilitystate")
private Integer communityvisibilitystate;
/**
*
*/
@JsonProperty("lastlogoff")
private Integer lastlogoff;
/**
*
*/
@JsonProperty("loccityid")
private Integer loccityid;
/**
*
*/
@JsonProperty("loccountrycode")
private String loccountrycode;
/**
*
*/
@JsonProperty("locstatecode")
private String locstatecode;
/**
*
*/
@JsonProperty("personaname")
private String personaname;
/**
*
*/
@JsonProperty("personastate")
private Integer personastate;
/**
*
*/
@JsonProperty("personastateflags")
private Integer personastateflags;
/**
*
*/
@JsonProperty("primaryclanid")
private String primaryclanid;
/**
*
*/
@JsonProperty("profilestate")
private Integer profilestate;
/**
*
*/
@JsonProperty("profileurl")
private String profileurl;
/**
*
*/
@JsonProperty("realname")
private String realname;
/**
*
*/
@JsonProperty("steamid")
private String steamid;
/**
*
*/
@JsonProperty("timecreated")
private Integer timecreated;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
*
* @return
* The avatar
*/
@JsonProperty("avatar")
public String getAvatar() {
return avatar;
}
/**
*
* @param avatar
* The avatar
*/
@JsonProperty("avatar")
public void setAvatar(String avatar) {
this.avatar = avatar;
}
public Player withAvatar(String avatar) {
this.avatar = avatar;
return this;
}
/**
*
* @return
* The avatarfull
*/
@JsonProperty("avatarfull")
public String getAvatarfull() {
return avatarfull;
}
/**
*
* @param avatarfull
* The avatarfull
*/
@JsonProperty("avatarfull")
public void setAvatarfull(String avatarfull) {
this.avatarfull = avatarfull;
}
public Player withAvatarfull(String avatarfull) {
this.avatarfull = avatarfull;
return this;
}
/**
*
* @return
* The avatarmedium
*/
@JsonProperty("avatarmedium")
public String getAvatarmedium() {
return avatarmedium;
}
/**
*
* @param avatarmedium
* The avatarmedium
*/
@JsonProperty("avatarmedium")
public void setAvatarmedium(String avatarmedium) {
this.avatarmedium = avatarmedium;
}
public Player withAvatarmedium(String avatarmedium) {
this.avatarmedium = avatarmedium;
return this;
}
/**
*
* @return
* The communityvisibilitystate
*/
@JsonProperty("communityvisibilitystate")
public Integer getCommunityvisibilitystate() {
return communityvisibilitystate;
}
/**
*
* @param communityvisibilitystate
* The communityvisibilitystate
*/
@JsonProperty("communityvisibilitystate")
public void setCommunityvisibilitystate(Integer communityvisibilitystate) {
this.communityvisibilitystate = communityvisibilitystate;
}
public Player withCommunityvisibilitystate(Integer communityvisibilitystate) {
this.communityvisibilitystate = communityvisibilitystate;
return this;
}
/**
*
* @return
* The lastlogoff
*/
@JsonProperty("lastlogoff")
public Integer getLastlogoff() {
return lastlogoff;
}
/**
*
* @param lastlogoff
* The lastlogoff
*/
@JsonProperty("lastlogoff")
public void setLastlogoff(Integer lastlogoff) {
this.lastlogoff = lastlogoff;
}
public Player withLastlogoff(Integer lastlogoff) {
this.lastlogoff = lastlogoff;
return this;
}
/**
*
* @return
* The loccityid
*/
@JsonProperty("loccityid")
public Integer getLoccityid() {
return loccityid;
}
/**
*
* @param loccityid
* The loccityid
*/
@JsonProperty("loccityid")
public void setLoccityid(Integer loccityid) {
this.loccityid = loccityid;
}
public Player withLoccityid(Integer loccityid) {
this.loccityid = loccityid;
return this;
}
/**
*
* @return
* The loccountrycode
*/
@JsonProperty("loccountrycode")
public String getLoccountrycode() {
return loccountrycode;
}
/**
*
* @param loccountrycode
* The loccountrycode
*/
@JsonProperty("loccountrycode")
public void setLoccountrycode(String loccountrycode) {
this.loccountrycode = loccountrycode;
}
public Player withLoccountrycode(String loccountrycode) {
this.loccountrycode = loccountrycode;
return this;
}
/**
*
* @return
* The locstatecode
*/
@JsonProperty("locstatecode")
public String getLocstatecode() {
return locstatecode;
}
/**
*
* @param locstatecode
* The locstatecode
*/
@JsonProperty("locstatecode")
public void setLocstatecode(String locstatecode) {
this.locstatecode = locstatecode;
}
public Player withLocstatecode(String locstatecode) {
this.locstatecode = locstatecode;
return this;
}
/**
*
* @return
* The personaname
*/
@JsonProperty("personaname")
public String getPersonaname() {
return personaname;
}
/**
*
* @param personaname
* The personaname
*/
@JsonProperty("personaname")
public void setPersonaname(String personaname) {
this.personaname = personaname;
}
public Player withPersonaname(String personaname) {
this.personaname = personaname;
return this;
}
/**
*
* @return
* The personastate
*/
@JsonProperty("personastate")
public Integer getPersonastate() {
return personastate;
}
/**
*
* @param personastate
* The personastate
*/
@JsonProperty("personastate")
public void setPersonastate(Integer personastate) {
this.personastate = personastate;
}
public Player withPersonastate(Integer personastate) {
this.personastate = personastate;
return this;
}
/**
*
* @return
* The personastateflags
*/
@JsonProperty("personastateflags")
public Integer getPersonastateflags() {
return personastateflags;
}
/**
*
* @param personastateflags
* The personastateflags
*/
@JsonProperty("personastateflags")
public void setPersonastateflags(Integer personastateflags) {
this.personastateflags = personastateflags;
}
public Player withPersonastateflags(Integer personastateflags) {
this.personastateflags = personastateflags;
return this;
}
/**
*
* @return
* The primaryclanid
*/
@JsonProperty("primaryclanid")
public String getPrimaryclanid() {
return primaryclanid;
}
/**
*
* @param primaryclanid
* The primaryclanid
*/
@JsonProperty("primaryclanid")
public void setPrimaryclanid(String primaryclanid) {
this.primaryclanid = primaryclanid;
}
public Player withPrimaryclanid(String primaryclanid) {
this.primaryclanid = primaryclanid;
return this;
}
/**
*
* @return
* The profilestate
*/
@JsonProperty("profilestate")
public Integer getProfilestate() {
return profilestate;
}
/**
*
* @param profilestate
* The profilestate
*/
@JsonProperty("profilestate")
public void setProfilestate(Integer profilestate) {
this.profilestate = profilestate;
}
public Player withProfilestate(Integer profilestate) {
this.profilestate = profilestate;
return this;
}
/**
*
* @return
* The profileurl
*/
@JsonProperty("profileurl")
public String getProfileurl() {
return profileurl;
}
/**
*
* @param profileurl
* The profileurl
*/
@JsonProperty("profileurl")
public void setProfileurl(String profileurl) {
this.profileurl = profileurl;
}
public Player withProfileurl(String profileurl) {
this.profileurl = profileurl;
return this;
}
/**
*
* @return
* The realname
*/
@JsonProperty("realname")
public String getRealname() {
return realname;
}
/**
*
* @param realname
* The realname
*/
@JsonProperty("realname")
public void setRealname(String realname) {
this.realname = realname;
}
public Player withRealname(String realname) {
this.realname = realname;
return this;
}
/**
*
* @return
* The steamid
*/
@JsonProperty("steamid")
public String getSteamid() {
return steamid;
}
/**
*
* @param steamid
* The steamid
*/
@JsonProperty("steamid")
public void setSteamid(String steamid) {
this.steamid = steamid;
}
public Player withSteamid(String steamid) {
this.steamid = steamid;
return this;
}
/**
*
* @return
* The timecreated
*/
@JsonProperty("timecreated")
public Integer getTimecreated() {
return timecreated;
}
/**
*
* @param timecreated
* The timecreated
*/
@JsonProperty("timecreated")
public void setTimecreated(Integer timecreated) {
this.timecreated = timecreated;
}
public Player withTimecreated(Integer timecreated) {
this.timecreated = timecreated;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Player withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(avatar).append(avatarfull).append(avatarmedium).append(communityvisibilitystate).append(lastlogoff).append(loccityid).append(loccountrycode).append(locstatecode).append(personaname).append(personastate).append(personastateflags).append(primaryclanid).append(profilestate).append(profileurl).append(realname).append(steamid).append(timecreated).append(additionalProperties).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof Player) == false) {
return false;
}
Player rhs = ((Player) other);
return new EqualsBuilder().append(avatar, rhs.avatar).append(avatarfull, rhs.avatarfull).append(avatarmedium, rhs.avatarmedium).append(communityvisibilitystate, rhs.communityvisibilitystate).append(lastlogoff, rhs.lastlogoff).append(loccityid, rhs.loccityid).append(loccountrycode, rhs.loccountrycode).append(locstatecode, rhs.locstatecode).append(personaname, rhs.personaname).append(personastate, rhs.personastate).append(personastateflags, rhs.personastateflags).append(primaryclanid, rhs.primaryclanid).append(profilestate, rhs.profilestate).append(profileurl, rhs.profileurl).append(realname, rhs.realname).append(steamid, rhs.steamid).append(timecreated, rhs.timecreated).append(additionalProperties, rhs.additionalProperties).isEquals();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy