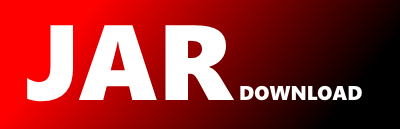
com.lukaspradel.steamapi.webapi.client.SteamWebApiClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of steam-web-api Show documentation
Show all versions of steam-web-api Show documentation
A library to provide access to the data available from Valve's Steam API
package com.lukaspradel.steamapi.webapi.client;
import java.io.IOException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.lukaspradel.steamapi.core.exception.SteamApiException;
import com.lukaspradel.steamapi.webapi.request.SteamWebApiRequest;
import com.lukaspradel.steamapi.webapi.request.SteamWebApiRequestHandler;
/**
* Create instances of this using {@link SteamWebApiClientBuilder}. This class
* should be used to pass instances of {@link SteamWebApiRequest} to the Steam
* servers and get the response-POJO.
*
* @author lpradel
*
*/
public class SteamWebApiClient {
private static final ObjectMapper MAPPER = new ObjectMapper();
private final String key;
private final boolean useHttps;
private final SteamWebApiRequestHandler requestHandler;
private SteamWebApiClient(SteamWebApiClientBuilder builder) {
this.key = builder.key;
this.useHttps = builder.useHttps;
this.requestHandler = new SteamWebApiRequestHandler(useHttps, key);
}
@SuppressWarnings({ "unchecked" })
public T processRequest(SteamWebApiRequest request)
throws SteamApiException {
T result = null;
String response = requestHandler.getWebApiResponse(request);
try {
result = (T) MAPPER.readValue(response, request.getResponseType());
} catch (IOException e) {
throw new SteamApiException(SteamApiException.Cause.MAPPING, e);
}
return result;
}
public String getKey() {
return key;
}
public boolean isUseHttps() {
return useHttps;
}
/**
* Builder class to create instances of {@link SteamWebApiClient}. Required
* parameters are
*
* - key : your Steam Web API Key
*
* Optional parameters are
*
* - useHttps : whether to communicate with Steam via HTTPS or plain HTTP.
* Default is false.
*
*
* @author lpradel
*
*/
public static class SteamWebApiClientBuilder {
private final String key;
private boolean useHttps = true;
/**
* Creates an instance of this class using your Steam Web API Key. Usage
* of HTTPS will be disabled by default, i.e. HTTP will
* be used to communicate with Steam.
*
* @param key
* Your Steam Web API Key
* @see http://steamcommunity.com/dev
*/
public SteamWebApiClientBuilder(String key) {
this.key = key;
}
public SteamWebApiClientBuilder useHttps(boolean useHttps) {
this.useHttps = useHttps;
return this;
}
public SteamWebApiClient build() {
return new SteamWebApiClient(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy