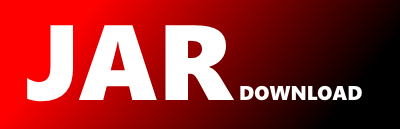
com.lumiomedical.flow.impl.pipeline.runtime.node.OffsetNode Maven / Gradle / Ivy
package com.lumiomedical.flow.impl.pipeline.runtime.node;
import com.lumiomedical.flow.node.Node;
import com.lumiomedical.flow.node.NodeDecorator;
import com.lumiomedical.flow.stream.StreamNode;
import java.util.List;
import java.util.stream.Collectors;
/**
* @author Pierre Lecerf ([email protected])
* Created on 2020/12/12
*/
public final class OffsetNode extends NodeDecorator
{
private final String uid;
private final int offset;
private List downstream;
private List requirements;
//private List requiredBy;
/**
*
* @param node
* @param offset
*/
public OffsetNode(Node node, int offset)
{
super(node);
this.offset = offset;
this.uid = node.getUid() + "#"+this.offset;
}
/**
*
* @return
*/
public String getUid()
{
return this.uid;
}
public int getOffset()
{
return this.offset;
}
@Override
public List getRequirements()
{
return this.getOffsetRequirements();
}
@Override
public List getDownstream()
{
return this.getOffsetDownstream();
}
/**
*
* @return
*/
private List getOffsetDownstream()
{
if (this.downstream != null)
return this.downstream;
synchronized (this) {
if (this.downstream != null)
return this.downstream;
this.downstream = this.getNode().getDownstream().stream()
.map(n -> {
if (n instanceof StreamNode)
return new OffsetNode(n, this.offset);
return n;
})
.collect(Collectors.toList())
;
}
return this.downstream;
}
/**
*
* @return
*/
private List getOffsetRequirements()
{
if (this.requirements != null)
return this.requirements;
synchronized (this) {
if (this.requirements != null)
return this.requirements;
this.requirements = this.getNode().getRequirements().stream()
.map(n -> {
if (n instanceof StreamNode)
return new OffsetNode(n, this.offset);
return n;
})
.collect(Collectors.toList())
;
}
return this.requirements;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy