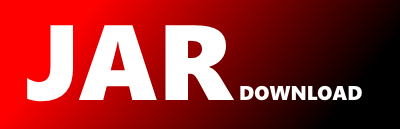
com.lumiomedical.flow.stream.StreamGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lumio-flow Show documentation
Show all versions of lumio-flow Show documentation
A library providing an opinionated way of structuring data processing programs such as ETLs
The newest version!
package com.lumiomedical.flow.stream;
import com.lumiomedical.flow.FlowIn;
import com.lumiomedical.flow.FlowOut;
import com.lumiomedical.flow.actor.accumulator.Accumulator;
import com.lumiomedical.flow.actor.generator.Generator;
import com.lumiomedical.flow.actor.loader.Loader;
import com.lumiomedical.flow.actor.transformer.BiTransformer;
import com.lumiomedical.flow.actor.transformer.Transformer;
import com.lumiomedical.flow.interruption.Interruption;
import com.lumiomedical.flow.node.SimpleNode;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* @author Pierre Lecerf ([email protected])
* Created on 2020/12/03
*/
public class StreamGenerator extends SimpleNode>> implements FlowIn, StreamOut
{
private int maxParallelism = 1;
/**
* @param generatorSupplier
*/
public StreamGenerator(Function> generatorSupplier)
{
super(generatorSupplier);
}
/**
*
* @param input
* @return
*/
public Generator produceGenerator(I input)
{
return this.getActor().apply(input);
}
@Override
public StreamPipe into(Transformer transformer)
{
var pipe = new StreamPipe<>(transformer);
this.bind(pipe);
return pipe;
}
@Override
public StreamSink into(Loader loader)
{
var sink = new StreamSink<>(loader);
this.bind(sink);
return sink;
}
@Override
public StreamJoin join(FlowOut input, BiTransformer transformer)
{
return new StreamJoin<>(this, input, transformer);
}
@Override
public StreamAccumulator accumulate(Accumulator accumulator)
{
var acc = new StreamAccumulator<>(accumulator);
this.bind(acc);
return acc;
}
/**
*
* @param loader
* @return
*/
public StreamGenerator driftSink(Loader loader)
{
this.into(loader);
return this;
}
/**
*
* @return
*/
public StreamPipe interrupt()
{
return this.into(new Interruption<>());
}
/**
*
* @param predicate
* @return
*/
public StreamPipe interruptIf(Predicate predicate)
{
return this.into(new Interruption<>(predicate));
}
public int getMaxParallelism()
{
return this.maxParallelism;
}
/**
*
* @param factor
* @return
*/
public StreamGenerator setMaxParallelism(int factor)
{
if (factor < 1)
throw new RuntimeException("StreamGenerator maximum parallelism factor is expected to be larger or equal to 1");
this.maxParallelism = factor;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy