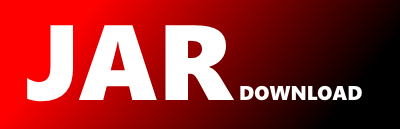
com.lzhpo.chatgpt.entity.chat.ChatCompletionRequest Maven / Gradle / Ivy
/*
* Copyright 2023 lzhpo
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lzhpo.chatgpt.entity.chat;
import cn.hutool.core.collection.ListUtil;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.lzhpo.chatgpt.entity.CommonConfig;
import java.util.List;
import java.util.Map;
import javax.validation.Valid;
import javax.validation.constraints.Max;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotEmpty;
import lombok.*;
import lombok.experimental.SuperBuilder;
/**
* @author lzhpo
*/
@Data
@SuperBuilder
@NoArgsConstructor
@AllArgsConstructor
@EqualsAndHashCode(callSuper = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class ChatCompletionRequest extends CommonConfig {
/**
* ID of the model to use.
*
* You can use the List models API to see all of your available models,
* or see our Model overview for descriptions of them.
* Model endpoint compatibility
*/
@NotBlank
@Builder.Default
private String model = "gpt-3.5-turbo";
/**
* The messages to generate chat completions for, in the chat format.
*/
@Valid
@NotEmpty
private List messages;
/**
* If set, partial message deltas will be sent, like in ChatGPT.
* Tokens will be sent as data-only server-sent events as they become available, with the stream terminated by a {@code data: [DONE]} message.
*
* See the OpenAI Cookbook for example code.
*/
private Boolean stream;
/**
* Up to 4 sequences where the API will stop generating further tokens.
*/
private String stop;
/**
* The maximum number of tokens to generate in the chat completion. Defaults to inf.
*
*
The total length of input tokens and generated tokens is limited by the model's context length.
*/
@JsonProperty("max_tokens")
private Integer maxTokens;
/**
* Number between -2.0 and 2.0. Positive values penalize new tokens based on whether they appear in the text so far,
* increasing the model's likelihood to talk about new topics.
*
*
See more information about frequency and presence penalties.
*/
@Min(-2)
@Max(2)
@JsonProperty("presence_penalty")
private Number presencePenalty;
/**
* Number between -2.0 and 2.0. Positive values penalize new tokens based on their existing frequency in the text so far,
* decreasing the model's likelihood to repeat the same line verbatim.
*
*
See more information about frequency and presence penalties.
*/
@Min(-2)
@Max(2)
@JsonProperty("frequency_penalty")
private Number frequencyPenalty;
/**
* Modify the likelihood of specified tokens appearing in the completion.
*
*
Accepts a json object that maps tokens (specified by their token ID in the tokenizer) to an associated bias value from -100 to 100.
* Mathematically, the bias is added to the logits generated by the model prior to sampling.
* The exact effect will vary per model, but values between -1 and 1 should decrease or increase likelihood of selection; values like -100 or 100 should result in a ban or exclusive selection of the relevant token.
*/
@JsonProperty("logit_bias")
private Map