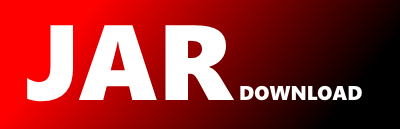
com.machinepublishers.jbrowserdriver.Robot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbrowserdriver Show documentation
Show all versions of jbrowserdriver Show documentation
A programmable, embedded web browser driver compatible with the Selenium WebDriver spec -- fast, headless, WebKit-based, 100% pure Java, and no browser dependencies
/*
* jBrowserDriver (TM)
* Copyright (C) 2014-2016 Machine Publishers, LLC and the jBrowserDriver contributors
* https://github.com/MachinePublishers/jBrowserDriver
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.machinepublishers.jbrowserdriver;
import java.awt.Transparency;
import java.awt.event.KeyEvent;
import java.awt.image.BufferedImage;
import java.awt.image.ColorModel;
import java.awt.image.ComponentColorModel;
import java.awt.image.DataBuffer;
import java.awt.image.DataBufferByte;
import java.awt.image.Raster;
import java.awt.image.WritableRaster;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicLong;
import java.util.concurrent.atomic.AtomicReference;
import javax.imageio.ImageIO;
import org.openqa.selenium.Keys;
import com.machinepublishers.jbrowserdriver.AppThread.Sync;
import com.sun.glass.ui.Application;
import com.sun.glass.ui.Pixels;
import javafx.embed.swing.SwingFXUtils;
import javafx.scene.Scene;
import javafx.scene.SnapshotParameters;
import javafx.scene.image.WritableImage;
import javafx.scene.input.KeyCode;
import javafx.stage.Stage;
class Robot {
private static final Map keysMap;
static {
Map keysMapTmp = new HashMap();
keysMapTmp.put(Keys.ADD, new int[] { KeyEvent.VK_ADD });
keysMapTmp.put(Keys.ALT, new int[] { KeyEvent.VK_ALT });
keysMapTmp.put(Keys.ARROW_DOWN, new int[] { KeyEvent.VK_DOWN });
keysMapTmp.put(Keys.ARROW_LEFT, new int[] { KeyEvent.VK_LEFT });
keysMapTmp.put(Keys.ARROW_RIGHT, new int[] { KeyEvent.VK_RIGHT });
keysMapTmp.put(Keys.ARROW_UP, new int[] { KeyEvent.VK_UP });
keysMapTmp.put(Keys.BACK_SPACE, new int[] { KeyEvent.VK_BACK_SPACE });
keysMapTmp.put(Keys.CANCEL, new int[] { KeyEvent.VK_CANCEL });
keysMapTmp.put(Keys.CLEAR, new int[] { KeyEvent.VK_CLEAR });
keysMapTmp.put(Keys.COMMAND, new int[] { KeyEvent.VK_META });
keysMapTmp.put(Keys.CONTROL, new int[] { KeyEvent.VK_CONTROL });
keysMapTmp.put(Keys.DECIMAL, new int[] { KeyEvent.VK_DECIMAL });
keysMapTmp.put(Keys.DELETE, new int[] { KeyEvent.VK_DELETE });
keysMapTmp.put(Keys.DIVIDE, new int[] { KeyEvent.VK_DIVIDE });
keysMapTmp.put(Keys.DOWN, new int[] { KeyEvent.VK_DOWN });
keysMapTmp.put(Keys.END, new int[] { KeyEvent.VK_END });
keysMapTmp.put(Keys.ENTER, new int[] { KeyEvent.VK_ENTER });
keysMapTmp.put(Keys.EQUALS, new int[] { KeyEvent.VK_EQUALS });
keysMapTmp.put(Keys.ESCAPE, new int[] { KeyEvent.VK_ESCAPE });
keysMapTmp.put(Keys.F1, new int[] { KeyEvent.VK_F1 });
keysMapTmp.put(Keys.F10, new int[] { KeyEvent.VK_F10 });
keysMapTmp.put(Keys.F11, new int[] { KeyEvent.VK_F11 });
keysMapTmp.put(Keys.F12, new int[] { KeyEvent.VK_F12 });
keysMapTmp.put(Keys.F2, new int[] { KeyEvent.VK_F2 });
keysMapTmp.put(Keys.F3, new int[] { KeyEvent.VK_F3 });
keysMapTmp.put(Keys.F4, new int[] { KeyEvent.VK_F4 });
keysMapTmp.put(Keys.F5, new int[] { KeyEvent.VK_F5 });
keysMapTmp.put(Keys.F6, new int[] { KeyEvent.VK_F6 });
keysMapTmp.put(Keys.F7, new int[] { KeyEvent.VK_F7 });
keysMapTmp.put(Keys.F8, new int[] { KeyEvent.VK_F8 });
keysMapTmp.put(Keys.F9, new int[] { KeyEvent.VK_F9 });
keysMapTmp.put(Keys.HELP, new int[] { KeyEvent.VK_HELP });
keysMapTmp.put(Keys.HOME, new int[] { KeyEvent.VK_HOME });
keysMapTmp.put(Keys.INSERT, new int[] { KeyEvent.VK_INSERT });
keysMapTmp.put(Keys.LEFT, new int[] { KeyEvent.VK_LEFT });
keysMapTmp.put(Keys.LEFT_ALT, new int[] { KeyEvent.VK_ALT });
keysMapTmp.put(Keys.LEFT_CONTROL, new int[] { KeyEvent.VK_CONTROL });
keysMapTmp.put(Keys.LEFT_SHIFT, new int[] { KeyEvent.VK_SHIFT });
keysMapTmp.put(Keys.META, new int[] { KeyEvent.VK_META });
keysMapTmp.put(Keys.MULTIPLY, new int[] { KeyEvent.VK_MULTIPLY });
keysMapTmp.put(Keys.NUMPAD0, new int[] { KeyEvent.VK_NUMPAD0 });
keysMapTmp.put(Keys.NUMPAD1, new int[] { KeyEvent.VK_NUMPAD1 });
keysMapTmp.put(Keys.NUMPAD2, new int[] { KeyEvent.VK_NUMPAD2 });
keysMapTmp.put(Keys.NUMPAD3, new int[] { KeyEvent.VK_NUMPAD3 });
keysMapTmp.put(Keys.NUMPAD4, new int[] { KeyEvent.VK_NUMPAD4 });
keysMapTmp.put(Keys.NUMPAD5, new int[] { KeyEvent.VK_NUMPAD5 });
keysMapTmp.put(Keys.NUMPAD6, new int[] { KeyEvent.VK_NUMPAD6 });
keysMapTmp.put(Keys.NUMPAD7, new int[] { KeyEvent.VK_NUMPAD7 });
keysMapTmp.put(Keys.NUMPAD8, new int[] { KeyEvent.VK_NUMPAD8 });
keysMapTmp.put(Keys.NUMPAD9, new int[] { KeyEvent.VK_NUMPAD9 });
keysMapTmp.put(Keys.PAGE_DOWN, new int[] { KeyEvent.VK_PAGE_DOWN });
keysMapTmp.put(Keys.PAGE_UP, new int[] { KeyEvent.VK_PAGE_UP });
keysMapTmp.put(Keys.PAUSE, new int[] { KeyEvent.VK_PAUSE });
keysMapTmp.put(Keys.RETURN, new int[] { KeyEvent.VK_ENTER });
keysMapTmp.put(Keys.RIGHT, new int[] { KeyEvent.VK_RIGHT });
keysMapTmp.put(Keys.SEMICOLON, new int[] { KeyEvent.VK_SEMICOLON });
keysMapTmp.put(Keys.SEPARATOR, new int[] { KeyEvent.VK_SEPARATOR });
keysMapTmp.put(Keys.SHIFT, new int[] { KeyEvent.VK_SHIFT });
keysMapTmp.put(Keys.SPACE, new int[] { KeyEvent.VK_SPACE });
keysMapTmp.put(Keys.SUBTRACT, new int[] { KeyEvent.VK_SUBTRACT });
keysMapTmp.put(Keys.TAB, new int[] { KeyEvent.VK_TAB });
keysMapTmp.put(Keys.UP, new int[] { KeyEvent.VK_UP });
keysMap = Collections.unmodifiableMap(keysMapTmp);
}
private static final Map textMap;
static {
Map textMapTmp = new HashMap();
textMapTmp.put("1", new int[] { KeyEvent.VK_1 });
textMapTmp.put("2", new int[] { KeyEvent.VK_2 });
textMapTmp.put("3", new int[] { KeyEvent.VK_3 });
textMapTmp.put("4", new int[] { KeyEvent.VK_4 });
textMapTmp.put("5", new int[] { KeyEvent.VK_5 });
textMapTmp.put("6", new int[] { KeyEvent.VK_6 });
textMapTmp.put("7", new int[] { KeyEvent.VK_7 });
textMapTmp.put("8", new int[] { KeyEvent.VK_8 });
textMapTmp.put("9", new int[] { KeyEvent.VK_9 });
textMapTmp.put("0", new int[] { KeyEvent.VK_0 });
textMapTmp.put("a", new int[] { KeyEvent.VK_A });
textMapTmp.put("b", new int[] { KeyEvent.VK_B });
textMapTmp.put("c", new int[] { KeyEvent.VK_C });
textMapTmp.put("d", new int[] { KeyEvent.VK_D });
textMapTmp.put("e", new int[] { KeyEvent.VK_E });
textMapTmp.put("f", new int[] { KeyEvent.VK_F });
textMapTmp.put("g", new int[] { KeyEvent.VK_G });
textMapTmp.put("h", new int[] { KeyEvent.VK_H });
textMapTmp.put("i", new int[] { KeyEvent.VK_I });
textMapTmp.put("j", new int[] { KeyEvent.VK_J });
textMapTmp.put("k", new int[] { KeyEvent.VK_K });
textMapTmp.put("l", new int[] { KeyEvent.VK_L });
textMapTmp.put("m", new int[] { KeyEvent.VK_M });
textMapTmp.put("n", new int[] { KeyEvent.VK_N });
textMapTmp.put("o", new int[] { KeyEvent.VK_O });
textMapTmp.put("p", new int[] { KeyEvent.VK_P });
textMapTmp.put("q", new int[] { KeyEvent.VK_Q });
textMapTmp.put("r", new int[] { KeyEvent.VK_R });
textMapTmp.put("s", new int[] { KeyEvent.VK_S });
textMapTmp.put("t", new int[] { KeyEvent.VK_T });
textMapTmp.put("u", new int[] { KeyEvent.VK_U });
textMapTmp.put("v", new int[] { KeyEvent.VK_V });
textMapTmp.put("w", new int[] { KeyEvent.VK_W });
textMapTmp.put("x", new int[] { KeyEvent.VK_X });
textMapTmp.put("y", new int[] { KeyEvent.VK_Y });
textMapTmp.put("z", new int[] { KeyEvent.VK_Z });
textMapTmp.put("A", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_A });
textMapTmp.put("B", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_B });
textMapTmp.put("C", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_C });
textMapTmp.put("D", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_D });
textMapTmp.put("E", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_E });
textMapTmp.put("F", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_F });
textMapTmp.put("G", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_G });
textMapTmp.put("H", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_H });
textMapTmp.put("I", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_I });
textMapTmp.put("J", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_J });
textMapTmp.put("K", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_K });
textMapTmp.put("L", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_L });
textMapTmp.put("M", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_M });
textMapTmp.put("N", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_N });
textMapTmp.put("O", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_O });
textMapTmp.put("P", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_P });
textMapTmp.put("Q", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_Q });
textMapTmp.put("R", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_R });
textMapTmp.put("S", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_S });
textMapTmp.put("T", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_T });
textMapTmp.put("U", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_U });
textMapTmp.put("V", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_V });
textMapTmp.put("W", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_W });
textMapTmp.put("X", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_X });
textMapTmp.put("Y", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_Y });
textMapTmp.put("Z", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_Z });
textMapTmp.put("`", new int[] { KeyEvent.VK_BACK_QUOTE });
textMapTmp.put("-", new int[] { KeyEvent.VK_MINUS });
textMapTmp.put("=", new int[] { KeyEvent.VK_EQUALS });
textMapTmp.put("[", new int[] { KeyEvent.VK_OPEN_BRACKET });
textMapTmp.put("]", new int[] { KeyEvent.VK_CLOSE_BRACKET });
textMapTmp.put("\\", new int[] { KeyEvent.VK_BACK_SLASH });
textMapTmp.put(";", new int[] { KeyEvent.VK_SEMICOLON });
textMapTmp.put("'", new int[] { KeyEvent.VK_QUOTE });
textMapTmp.put(",", new int[] { KeyEvent.VK_COMMA });
textMapTmp.put(".", new int[] { KeyEvent.VK_PERIOD });
textMapTmp.put("/", new int[] { KeyEvent.VK_SLASH });
textMapTmp.put("~", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_BACK_QUOTE });
textMapTmp.put("_", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_MINUS });
textMapTmp.put("+", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_EQUALS });
textMapTmp.put("{", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_OPEN_BRACKET });
textMapTmp.put("}", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_CLOSE_BRACKET });
textMapTmp.put("|", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_BACK_SLASH });
textMapTmp.put(":", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_SEMICOLON });
textMapTmp.put("\"", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_QUOTE });
textMapTmp.put("<", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_COMMA });
textMapTmp.put(">", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_PERIOD });
textMapTmp.put("?", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_SLASH });
textMapTmp.put("!", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_1 });
textMapTmp.put("@", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_2 });
textMapTmp.put("#", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_3 });
textMapTmp.put("$", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_4 });
textMapTmp.put("%", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_5 });
textMapTmp.put("^", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_6 });
textMapTmp.put("&", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_7 });
textMapTmp.put("*", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_8 });
textMapTmp.put("(", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_9 });
textMapTmp.put(")", new int[] { KeyEvent.VK_SHIFT, KeyEvent.VK_0 });
textMapTmp.put("\t", new int[] { KeyEvent.VK_TAB });
textMapTmp.put("\n", new int[] { KeyEvent.VK_ENTER });
textMapTmp.put(" ", new int[] { KeyEvent.VK_SPACE });
textMap = Collections.unmodifiableMap(textMapTmp);
}
static enum MouseButton {
LEFT(com.sun.glass.ui.Robot.MOUSE_LEFT_BTN), MIDDLE(com.sun.glass.ui.Robot.MOUSE_MIDDLE_BTN), RIGHT(com.sun.glass.ui.Robot.MOUSE_RIGHT_BTN);
private final int value;
MouseButton(int value) {
this.value = value;
}
int getValue() {
return value;
}
}
private static final int LINE_FEED = "\n".codePointAt(0);
private static final int CARRIAGE_RETURN = "\r".codePointAt(0);
private static final int ENTER = Keys.ENTER.toString().codePointAt(0);
private final AtomicReference robot = new AtomicReference();
private final AtomicLong latestThread = new AtomicLong();
private final AtomicLong curThread = new AtomicLong();
private final Context context;
Robot(final Context context) {
robot.set(AppThread.exec(context.item().statusCode, new Sync() {
public com.sun.glass.ui.Robot perform() {
return Application.GetApplication().createRobot();
}
}));
this.context = context;
}
private static int[] convertKey(int codePoint) {
char[] chars = Character.toChars(codePoint);
if (chars.length == 1) {
Keys key = Keys.getKeyFromUnicode(chars[0]);
if (key != null) {
return keysMap.get(key);
}
}
String str = new String(new int[] { codePoint }, 0, 1);
int[] mapping = textMap.get(str);
return mapping;
}
private static boolean isChord(CharSequence charSequence) {
int[] codePoints = charSequence.codePoints().toArray();
if (codePoints.length > 0) {
char[] chars = Character.toChars(codePoints[codePoints.length - 1]);
if (chars.length == 1) {
return Keys.NULL.equals(Keys.getKeyFromUnicode(chars[0]));
}
}
return false;
}
private void lock() {
long myThread = latestThread.incrementAndGet();
synchronized (curThread) {
while (myThread != curThread.get() + 1) {
try {
curThread.wait();
} catch (InterruptedException e) {}
}
}
AppThread.exec(context.item().statusCode, new Sync
© 2015 - 2024 Weber Informatics LLC | Privacy Policy