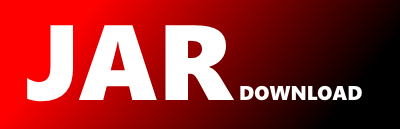
com.machinezoo.noexception.optional.OptionalDoubleToIntFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of noexception Show documentation
Show all versions of noexception Show documentation
Makes exception handling concise and beautiful, yet architecturally clean and flexible.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy