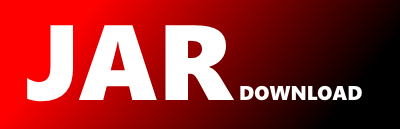
com.machinezoo.sourceafis.Block Maven / Gradle / Ivy
// Part of SourceAFIS: https://sourceafis.machinezoo.com
package com.machinezoo.sourceafis;
import java.util.*;
class Block implements Iterable {
final int x;
final int y;
final int width;
final int height;
Block(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
Block(Cell size) {
this(0, 0, size.x, size.y);
}
int left() {
return x;
}
int top() {
return y;
}
int right() {
return x + width;
}
int bottom() {
return y + height;
}
int area() {
return width * height;
}
static Block between(int startX, int startY, int endX, int endY) {
return new Block(startX, startY, endX - startX, endY - startY);
}
static Block between(Cell start, Cell end) {
return between(start.x, start.y, end.x, end.y);
}
static Block around(int x, int y, int radius) {
return between(x - radius, y - radius, x + radius + 1, y + radius + 1);
}
static Block around(Cell center, int radius) {
return around(center.x, center.y, radius);
}
Cell center() {
return new Cell((right() + left()) / 2, (top() + bottom()) / 2);
}
Block intersect(Block other) {
return between(
new Cell(Math.max(left(), other.left()), Math.max(top(), other.top())),
new Cell(Math.min(right(), other.right()), Math.min(bottom(), other.bottom())));
}
Block move(Cell delta) {
return new Block(x + delta.x, y + delta.y, width, height);
}
private List |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy