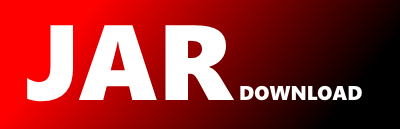
org.kohsuke.github.GHTeam Maven / Gradle / Ivy
package org.kohsuke.github;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
/**
* A team in GitHub organization.
*
* @author Kohsuke Kawaguchi
*/
public class GHTeam {
private String name,permission;
private int id;
private GHOrganization organization; // populated by GET /user/teams where Teams+Orgs are returned together
protected /*final*/ GHOrganization org;
/*package*/ GHTeam wrapUp(GHOrganization owner) {
this.org = owner;
return this;
}
/*package*/ GHTeam wrapUp(GitHub root) { // auto-wrapUp when organization is known from GET /user/teams
this.organization.wrapUp(root);
return wrapUp(organization);
}
/*package*/ static GHTeam[] wrapUp(GHTeam[] teams, GHOrganization owner) {
for (GHTeam t : teams) {
t.wrapUp(owner);
}
return teams;
}
public String getName() {
return name;
}
public String getPermission() {
return permission;
}
public int getId() {
return id;
}
/**
* Retrieves the current members.
*/
public Set getMembers() throws IOException {
return new HashSet(Arrays.asList(GHUser.wrap(org.root.retrieve().to(api("/members"), GHUser[].class), org.root)));
}
/**
* Checks if this team has the specified user as a member.
*/
public boolean hasMember(GHUser user) {
try {
org.root.retrieve().to("/teams/" + id + "/members/" + user.getLogin());
return true;
} catch (IOException ignore) {
return false;
}
}
public Map getRepositories() throws IOException {
Map m = new TreeMap();
for (GHRepository r : listRepositories()) {
m.put(r.getName(), r);
}
return m;
}
public PagedIterable listRepositories() {
return new PagedIterable() {
public PagedIterator iterator() {
return new PagedIterator(org.root.retrieve().asIterator(api("/repos"), GHRepository[].class)) {
@Override
protected void wrapUp(GHRepository[] page) {
for (GHRepository r : page)
r.wrap(org.root);
}
};
}
};
}
/**
* Adds a member to the team.
*
* The user will be invited to the organization if required.
*
* @since 1.59
*/
public void add(GHUser u) throws IOException {
org.root.retrieve().method("PUT").to(api("/memberships/" + u.getLogin()), null);
}
/**
* Removes a member to the team.
*/
public void remove(GHUser u) throws IOException {
org.root.retrieve().method("DELETE").to(api("/members/" + u.getLogin()), null);
}
public void add(GHRepository r) throws IOException {
org.root.retrieve().method("PUT").to(api("/repos/" + r.getOwnerName() + '/' + r.getName()), null);
}
public void remove(GHRepository r) throws IOException {
org.root.retrieve().method("DELETE").to(api("/repos/" + r.getOwnerName() + '/' + r.getName()), null);
}
private String api(String tail) {
return "/teams/"+id+tail;
}
public GHOrganization getOrganization() {
return org;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy