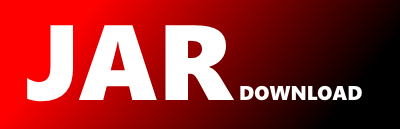
com.magic80.springBootCommon.helper.PagedHelper Maven / Gradle / Ivy
package com.magic80.springBootCommon.helper;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import com.magic80.springBootCommon.helper.page.PagedMutipleTableCountFunction;
import com.magic80.springBootCommon.helper.page.PagedMutipleTableFunction;
import com.magic80.springBootCommon.helper.page.PagedSingleTableFunction;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
public class PagedHelper {
public static Map pagedList(Map requestMap,
PagedSingleTableFunction singleTableFunction) {
PagedParameterParser parameterParser = new PagedParameterParser(requestMap);
Page page = PageHelper.startPage(parameterParser.getPageIndex(), parameterParser.getPageSize(), true);
List list = singleTableFunction.call(requestMap);
return formatPagedList(
page.getTotal(),
list
);
}
public static Map pagedList(Map requestMap,
PagedMutipleTableFunction pagedMutipleTableFunction,
PagedMutipleTableCountFunction pagedMutipleTableCountFunction) {
PagedParameterParser parameterParser = new PagedParameterParser(requestMap);
return formatPagedList(
pagedMutipleTableCountFunction.call(requestMap),
pagedMutipleTableFunction.call(
(parameterParser.getPageIndex() - 1) * parameterParser.getPageSize(),
parameterParser.getPageSize(),
requestMap
)
);
}
private static Map formatPagedList(Long total, List list) {
return new HashMap(){
{
put("total", total);
put("list", list);
}
};
}
private static class PagedParameterParser{
private Map requestMap;
public PagedParameterParser(Map requestMap) {
this.requestMap = requestMap;
}
/**
* 起始页码从1开始
* @return
*/
public Integer getPageIndex() {
return parse("pageIndex", 1);
}
public Integer getPageSize() {
return parse("pageSize", 10);
}
private Integer parse(String key, Integer defaultValue) {
return Optional
.ofNullable(requestMap.get(key))
.map(Integer::parseInt)
.orElse(defaultValue);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy