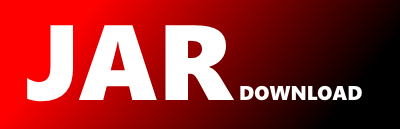
com.mailgun.api.v3.suppression.MailgunSuppressionComplaintsApi Maven / Gradle / Ivy
Show all versions of mailgun-java Show documentation
package com.mailgun.api.v3.suppression;
import com.mailgun.api.MailgunApi;
import com.mailgun.model.ResponseWithMessage;
import com.mailgun.model.suppression.SuppressionResponse;
import com.mailgun.model.suppression.bounces.ComplaintsListImportRequest;
import com.mailgun.model.suppression.complaints.ComplaintsItem;
import com.mailgun.model.suppression.complaints.ComplaintsItemResponse;
import com.mailgun.model.suppression.complaints.ComplaintsSingleItemRequest;
import feign.Headers;
import feign.Param;
import feign.RequestLine;
import feign.Response;
import java.util.List;
/**
*
* Mailgun Suppression Complaints Api
*
*
*
* Mailgun keeps three lists of addresses it blocks the delivery to: bounces, unsubscribes and complaints.
* These lists are populated automatically as Mailgun detects undeliverable addresses you try to send to
* and as recipients unsubscribe from your mailings or mark your emails as a spam (for ESPs that provide FBL).
* You can also add/remove addresses from any of these lists using the API.
*
*
* It’s important to note that these suppression lists are unique to a sending domain and are not an account level (global) suppression list.
* If you want to add/remove the same address(es) from multiple domains, you’ll need to do so for each domain.
*
*
*
* Complaint list stores email addresses of recipients who marked your messages as a spam (for ESPs that support FBL).
*
*
*
* Mailgun can notify your application every time a recipient flags your message as spam via a
* complained webhook.
*
*
* @see Suppressions/Complaints
*/
@Headers("Accept: application/json")
public interface MailgunSuppressionComplaintsApi extends MailgunApi {
/**
*
* Returns a list of complaints for a domain (limit to 100 entries).
*
*
* Note Via this API method complaints are returned in the alphabetical order.
* If you wish to poll for the recently occurred complaints, please consider using the {@link com.mailgun.api.v3.MailgunEventsApi}.
*
*
* @param domain Name of the domain
* @return {@link ComplaintsItemResponse}
*/
@RequestLine("GET /{domain}/complaints")
ComplaintsItemResponse getAllComplaints(@Param("domain") String domain);
/**
*
* Returns a list of complaints for a domain (limit to 100 entries).
*
*
* Note Via this API method complaints are returned in the alphabetical order.
* If you wish to poll for the recently occurred complaints, please consider using the {@link com.mailgun.api.v3.MailgunEventsApi}.
*
*
* @param domain Name of the domain
* @return {@link Response}
*/
@RequestLine("GET /{domain}/complaints")
Response getAllComplaintsFeignResponse(@Param("domain") String domain);
/**
*
* Returns a list of complaints for a domain.
*
*
* Note Via this API method complaints are returned in the alphabetical order.
* If you wish to poll for the recently occurred complaints, please consider using the {@link com.mailgun.api.v3.MailgunEventsApi}.
*
*
* @param domain Name of the domain
* @param limit Number of entries to return, max: 10000.
* @return {@link ComplaintsItemResponse}
*/
@RequestLine("GET /{domain}/complaints?limit={limit}")
ComplaintsItemResponse getAllComplaints(@Param("domain") String domain, @Param("limit") Integer limit);
/**
*
* Returns a list of complaints for a domain.
*
*
* Note Via this API method complaints are returned in the alphabetical order.
* If you wish to poll for the recently occurred complaints, please consider using the {@link com.mailgun.api.v3.MailgunEventsApi}.
*
*
* @param domain Name of the domain
* @param limit Number of entries to return, max: 10000.
* @return {@link Response}
*/
@RequestLine("GET /{domain}/complaints?limit={limit}")
Response getAllComplaintsFeignResponse(@Param("domain") String domain, @Param("limit") Integer limit);
/**
*
* Fetch a single spam complaint by a given email address. This is useful to check if a particular user has complained.
*
*
* @param domain Name of the domain
* @param address An email address
* @return {@link ComplaintsItem}
*/
@RequestLine("GET /{domain}/complaints/{address}")
ComplaintsItem getSingleComplaint(@Param("domain") String domain, @Param("address") String address);
/**
*
* Fetch a single spam complaint by a given email address. This is useful to check if a particular user has complained.
*
*
* @param domain Name of the domain
* @param address An email address
* @return {@link Response}
*/
@RequestLine("GET /{domain}/complaints/{address}")
Response getSingleComplaintFeignResponse(@Param("domain") String domain, @Param("address") String address);
/**
*
* Add an address to the complaints list.
*
*
* @param domain Name of the domain
* @param request {@link ComplaintsSingleItemRequest}
* @return {@link SuppressionResponse}
*/
@Headers("Content-Type: multipart/form-data")
@RequestLine("POST /{domain}/complaints")
SuppressionResponse addAddressToComplaintsList(@Param("domain") String domain, ComplaintsSingleItemRequest request);
/**
*
* Add an address to the complaints list.
*
*
* @param domain Name of the domain
* @param request {@link ComplaintsSingleItemRequest}
* @return {@link Response}
*/
@Headers("Content-Type: multipart/form-data")
@RequestLine("POST /{domain}/complaints")
Response addAddressToComplaintsListFeignResponse(@Param("domain") String domain, ComplaintsSingleItemRequest request);
/**
*
* Add multiple complaint records to the complaint list in a single API call(up to 1000 complaint records).
*
*
* @param domain Name of the domain
* @param request {@link ComplaintsItem}
* @return list of {@link ResponseWithMessage}
*/
@Headers("Content-Type: application/json")
@RequestLine("POST /{domain}/complaints")
ResponseWithMessage addAddressesToComplaintsList(@Param("domain") String domain, List request);
/**
*
* Add multiple complaint records to the complaint list in a single API call(up to 1000 complaint records).
*
*
* @param domain Name of the domain
* @param request {@link ComplaintsItem}
* @return list of {@link Response}
*/
@Headers("Content-Type: application/json")
@RequestLine("POST /{domain}/complaints")
Response addAddressesToComplaintsListFeignResponse(@Param("domain") String domain, List request);
/**
*
* Import a list of complaints.
*
*
* Import a CSV file containing a list of addresses to add to the complaint list.
*
*
* CSV file must be 25MB or under and must contain the following column headers:
*
*
* address
Valid email address
* created_at
Timestamp of a bounce event in RFC2822 format (optional, default: current time)
*
*
* @param domain Name of the domain
* @param request {@link ComplaintsItem}
* @return list of {@link ResponseWithMessage}
*/
@Headers("Content-Type: multipart/form-data")
@RequestLine("POST /{domain}/complaints/import")
ResponseWithMessage importComplaintsList(@Param("domain") String domain, ComplaintsListImportRequest request);
/**
*
* Add multiple complaints.
*
*
* Import a CSV file containing a list of addresses to add to the complaint list.
*
*
* CSV file must be 25MB or under and must contain the following column headers:
*
*
* address
Valid email address
* created_at
Timestamp of a bounce event in RFC2822 format (optional, default: current time)
*
*
* @param domain Name of the domain
* @param request {@link ComplaintsItem}
* @return list of {@link Response}
*/
@Headers("Content-Type: multipart/form-data")
@RequestLine("POST /{domain}/complaints/import")
Response importComplaintsListFeignResponse(@Param("domain") String domain, ComplaintsListImportRequest request);
/**
*
* Remove Address From Complaints.
*
*
* @param domain Name of the domain
* @param address An email address
* @return {@link SuppressionResponse}
*/
@RequestLine("DELETE /{domain}/complaints/{address}")
SuppressionResponse removeAddressFromComplaints(@Param("domain") String domain, @Param("address") String address);
/**
*
* Remove Address From Complaints.
*
*
* @param domain Name of the domain
* @param address An email address
* @return {@link Response}
*/
@RequestLine("DELETE /{domain}/complaints/{address}")
Response removeAddressFromComplaintsFeignResponse(@Param("domain") String domain, @Param("address") String address);
}