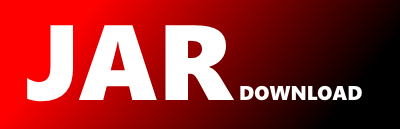
com.mailgun.model.StatisticsOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mailgun-java Show documentation
Show all versions of mailgun-java Show documentation
The Mailgun SDK for Java enables Java developers to work with Mailgun API
efficiently.
package com.mailgun.model;
import com.mailgun.enums.Duration;
import com.mailgun.enums.ResolutionPeriod;
import com.mailgun.enums.StatsEventType;
import com.mailgun.util.CollectionUtil;
import com.mailgun.util.DateTimeUtil;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.NonNull;
import lombok.Value;
import lombok.extern.jackson.Jacksonized;
import org.apache.commons.lang3.Validate;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
import static com.mailgun.util.Constants.DURATION_MUST_BE_GREATER_THAN_ZERO;
/**
*
* Mailgun tracks all of the events that occur throughout the system.
*
*
* @see Stats
*/
@Value
@Jacksonized
@Builder
@AllArgsConstructor
public class StatisticsOptions {
/**
*
* (Required) Event type(s).
*
* {@link StatsEventType}
*
* @see Event Types
*/
@NonNull
Set event;
/**
*
* The starting time.
*
* Default: 7 days from the current time.
*/
String start;
/**
*
* The ending date.
*
* Default: current time.
*/
String end;
/**
*
* Resolution
*
* {@link ResolutionPeriod}
*
* Can be either HOUR
, DAY
or MONTH
*
* Default: DAY
*/
String resolution;
/**
*
* Period of time with resolution encoded. If provided, overwrites the start date.
*
*
* @see Duration
*/
String duration;
public static class StatisticsOptionsBuilder {
/**
*
* Event type.
*
*
* @param event {@link StatsEventType}
* @return Returns a reference to this object so that method calls can be chained together.
* @see Event Types
*/
public StatisticsOptions.StatisticsOptionsBuilder event(StatsEventType event) {
this.event = CollectionUtil.addToSet(this.event, event.getValue());
return this;
}
/**
*
* Event types.
*
*
* @param events list of {@link StatsEventType}
* @return Returns a reference to this object so that method calls can be chained together.
* @see Event Types
*/
public StatisticsOptions.StatisticsOptionsBuilder event(List events) {
this.event = CollectionUtil.addToSet(this.event, events.stream()
.map(StatsEventType::getValue)
.collect(Collectors.toList()));
return this;
}
/**
*
* The starting time.
*
* Default: 7 days from the current time.
*
* @param zonedDateTime {@link ZonedDateTime}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatisticsOptions.StatisticsOptionsBuilder start(ZonedDateTime zonedDateTime) {
this.start = DateTimeUtil.toStringNumericTimeZone(zonedDateTime);
return this;
}
/**
*
* The ending date.
*
* Default: current time.
*
* @param zonedDateTime {@link ZonedDateTime}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatisticsOptions.StatisticsOptionsBuilder end(ZonedDateTime zonedDateTime) {
this.end = DateTimeUtil.toStringNumericTimeZone(zonedDateTime);
return this;
}
/**
*
* Resolution period.
*
*
* Can be either HOUR
, DAY
or MONTH
*
* Default: DAY
*
* @param resolutionPeriod {@link ResolutionPeriod}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatisticsOptions.StatisticsOptionsBuilder resolution(ResolutionPeriod resolutionPeriod) {
this.resolution = resolutionPeriod.getValue();
return this;
}
/**
*
* Period of time with resolution encoded. If provided, overwrites the start date.
*
*
* @param timeValue time value
* @param duration {@link Duration}
* @return Returns a reference to this object so that method calls can be chained together.
* @see Duration
*/
public StatisticsOptions.StatisticsOptionsBuilder duration(int timeValue, Duration duration) {
Validate.isTrue(timeValue > 0, DURATION_MUST_BE_GREATER_THAN_ZERO, timeValue);
String durationValue = duration.getValue();
this.duration = timeValue + durationValue;
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy