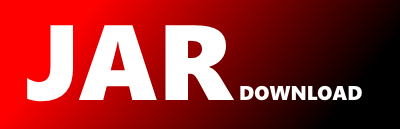
com.mailgun.model.events.EventItem Maven / Gradle / Ivy
Show all versions of mailgun-java Show documentation
package com.mailgun.model.events;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.mailgun.enums.Severity;
import lombok.Builder;
import lombok.Value;
import lombok.extern.jackson.Jacksonized;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Map;
/**
*
* Event.
*
*
* @see Events API
*/
@Value
@Jacksonized
@Builder
@JsonIgnoreProperties(ignoreUnknown = true)
public class EventItem {
/**
*
* Event id.
*
*/
String id;
/**
*
* Event Type.
*
*/
String event;
/**
*
* The time when the event was generated in the system.
*
*/
ZonedDateTime timestamp;
/**
*
* Event log level.
*
*/
@JsonProperty("log-level")
String logLevel;
/**
*
* {@link EventMember}
*
*/
EventMember member;
/**
*
* M
* Event method.
*
* E.g.:HTTP
, smtp
.
*/
String method;
/**
*
* {@link Severity}
*
*/
Severity severity;
/**
*
* Event reason.
*
*/
String reason;
/**
*
* List of {@link EventRoute}.
*
*/
List routes;
/**
*
* {@link EventEnvelope}
*
*/
EventEnvelope envelope;
/**
*
* {@link EventFlags}
*
*/
EventFlags flags;
/**
*
* {@link EventDeliveryStatus}
*
*/
@JsonProperty("delivery-status")
EventDeliveryStatus deliveryStatus;
/**
*
* {@link RejectedEventInfo}
*
*/
RejectedEventInfo reject;
/**
*
* {@link EventMessage}
*
*/
EventMessage message;
/**
*
* {@link Storage}
*
*/
Storage storage;
/**
*
* An email address of a particular recipient.
*
*/
String recipient;
/**
*
* Recipient domain.
*
*/
@JsonProperty("recipient-domain")
String recipientDomain;
/**
*
* List of campaigns.
*
*/
List campaigns;
/**
*
* {@link EventGeolocation}
*
*/
EventGeolocation geolocation;
/**
*
* List of tags.
*
*/
List tags;
/**
*
* Map of user variables.
*
*/
@JsonProperty("user-variables")
Map userVariables;
/**
*
* Event url.
*
*/
String url;
/**
*
* Event ip.
*
*/
String ip;
/**
*
* {@link EventClientInfo}
*
*/
@JsonProperty("client-info")
EventClientInfo clientInfo;
/**
*
* Event task id.
*
*/
@JsonProperty("task-id")
String taskId;
/**
*
* Event format.
*
*/
String format;
/**
*
* {@link EventMailingList}
*
*/
@JsonProperty("mailing-list")
EventMailingList mailingList;
@JsonProperty("failed-count")
Integer failedCount;
@JsonProperty("upserted-count")
Integer upsertedCount;
@JsonProperty("is-upsert")
Boolean isUpsert;
}