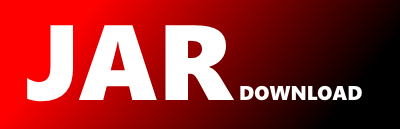
com.mailgun.model.routes.RoutesRequest Maven / Gradle / Ivy
Show all versions of mailgun-java Show documentation
package com.mailgun.model.routes;
import com.mailgun.util.CollectionUtil;
import lombok.Builder;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.ToString;
import java.util.List;
import java.util.Set;
/**
*
* Routes request.
*
*
* @see Routes
*/
@Getter
@ToString
@EqualsAndHashCode
@Builder
public class RoutesRequest {
/**
*
* Integer: smaller number indicates higher priority.
* Higher priority routes are handled first.
*
* Defaults to 0.
*/
Integer priority;
/**
*
* Route description (an arbitrary string).
*
*/
String description;
/**
*
* A filter expression like match_recipient('.*@gmail.com')
.
*
*/
String expression;
/**
*
* Route action.
*
*
* This action is executed when the expression evaluates to True
.
*
*
* Example: forward("[email protected]")
*
*
* You can pass multiple action parameters.
*
*/
Set action;
public static class RoutesRequestBuilder {
/**
*
* Route action.
*
*
* This action is executed when the expression evaluates to True
.
*
*
* Example: forward("[email protected]")
*
*
* You can pass multiple action parameters.
*
*
* @param action Route action
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RoutesRequest.RoutesRequestBuilder action(String action) {
this.action = CollectionUtil.addToSet(this.action, action);
return this;
}
/**
*
* Route actions.
*
*
* This action is executed when the expression evaluates to True
.
*
*
* Example: forward("[email protected]")
*
*
* @param actions list if route actions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RoutesRequest.RoutesRequestBuilder actions(List actions) {
this.action = CollectionUtil.addToSet(this.action, actions);
return this;
}
}
}