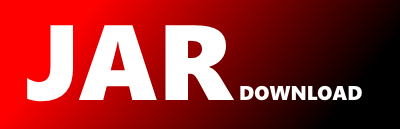
com.mailslurp.models.ConnectorSyncRequestResultException Maven / Gradle / Ivy
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.mailslurp.models.ConnectorSyncRequestResultExceptionCause;
import com.mailslurp.models.ConnectorSyncRequestResultExceptionCauseStackTrace;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* ConnectorSyncRequestResultException
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-05-03T02:14:29.400Z[GMT]")
public class ConnectorSyncRequestResultException {
public static final String SERIALIZED_NAME_CAUSE = "cause";
@SerializedName(SERIALIZED_NAME_CAUSE)
private ConnectorSyncRequestResultExceptionCause cause;
public static final String SERIALIZED_NAME_STACK_TRACE = "stackTrace";
@SerializedName(SERIALIZED_NAME_STACK_TRACE)
private List stackTrace = null;
public static final String SERIALIZED_NAME_MESSAGE = "message";
@SerializedName(SERIALIZED_NAME_MESSAGE)
private String message;
public static final String SERIALIZED_NAME_SUPPRESSED = "suppressed";
@SerializedName(SERIALIZED_NAME_SUPPRESSED)
private List suppressed = null;
public static final String SERIALIZED_NAME_LOCALIZED_MESSAGE = "localizedMessage";
@SerializedName(SERIALIZED_NAME_LOCALIZED_MESSAGE)
private String localizedMessage;
public ConnectorSyncRequestResultException cause(ConnectorSyncRequestResultExceptionCause cause) {
this.cause = cause;
return this;
}
/**
* Get cause
* @return cause
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ConnectorSyncRequestResultExceptionCause getCause() {
return cause;
}
public void setCause(ConnectorSyncRequestResultExceptionCause cause) {
this.cause = cause;
}
public ConnectorSyncRequestResultException stackTrace(List stackTrace) {
this.stackTrace = stackTrace;
return this;
}
public ConnectorSyncRequestResultException addStackTraceItem(ConnectorSyncRequestResultExceptionCauseStackTrace stackTraceItem) {
if (this.stackTrace == null) {
this.stackTrace = new ArrayList<>();
}
this.stackTrace.add(stackTraceItem);
return this;
}
/**
* Get stackTrace
* @return stackTrace
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getStackTrace() {
return stackTrace;
}
public void setStackTrace(List stackTrace) {
this.stackTrace = stackTrace;
}
public ConnectorSyncRequestResultException message(String message) {
this.message = message;
return this;
}
/**
* Get message
* @return message
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public ConnectorSyncRequestResultException suppressed(List suppressed) {
this.suppressed = suppressed;
return this;
}
public ConnectorSyncRequestResultException addSuppressedItem(ConnectorSyncRequestResultExceptionCause suppressedItem) {
if (this.suppressed == null) {
this.suppressed = new ArrayList<>();
}
this.suppressed.add(suppressedItem);
return this;
}
/**
* Get suppressed
* @return suppressed
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getSuppressed() {
return suppressed;
}
public void setSuppressed(List suppressed) {
this.suppressed = suppressed;
}
public ConnectorSyncRequestResultException localizedMessage(String localizedMessage) {
this.localizedMessage = localizedMessage;
return this;
}
/**
* Get localizedMessage
* @return localizedMessage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getLocalizedMessage() {
return localizedMessage;
}
public void setLocalizedMessage(String localizedMessage) {
this.localizedMessage = localizedMessage;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConnectorSyncRequestResultException connectorSyncRequestResultException = (ConnectorSyncRequestResultException) o;
return Objects.equals(this.cause, connectorSyncRequestResultException.cause) &&
Objects.equals(this.stackTrace, connectorSyncRequestResultException.stackTrace) &&
Objects.equals(this.message, connectorSyncRequestResultException.message) &&
Objects.equals(this.suppressed, connectorSyncRequestResultException.suppressed) &&
Objects.equals(this.localizedMessage, connectorSyncRequestResultException.localizedMessage);
}
@Override
public int hashCode() {
return Objects.hash(cause, stackTrace, message, suppressed, localizedMessage);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConnectorSyncRequestResultException {\n");
sb.append(" cause: ").append(toIndentedString(cause)).append("\n");
sb.append(" stackTrace: ").append(toIndentedString(stackTrace)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" suppressed: ").append(toIndentedString(suppressed)).append("\n");
sb.append(" localizedMessage: ").append(toIndentedString(localizedMessage)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy