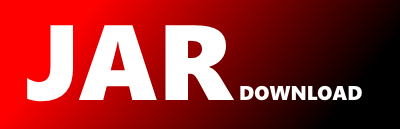
com.mailslurp.models.EmailAnalysis Maven / Gradle / Ivy
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Analysis result for email. Each verdict property is a string PASS|FAIL|GRAY or dynamic error message
*/
@ApiModel(description = "Analysis result for email. Each verdict property is a string PASS|FAIL|GRAY or dynamic error message")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-05-03T02:14:29.400Z[GMT]")
public class EmailAnalysis {
public static final String SERIALIZED_NAME_SPAM_VERDICT = "spamVerdict";
@SerializedName(SERIALIZED_NAME_SPAM_VERDICT)
private String spamVerdict;
public static final String SERIALIZED_NAME_VIRUS_VERDICT = "virusVerdict";
@SerializedName(SERIALIZED_NAME_VIRUS_VERDICT)
private String virusVerdict;
public static final String SERIALIZED_NAME_SPF_VERDICT = "spfVerdict";
@SerializedName(SERIALIZED_NAME_SPF_VERDICT)
private String spfVerdict;
public static final String SERIALIZED_NAME_DKIM_VERDICT = "dkimVerdict";
@SerializedName(SERIALIZED_NAME_DKIM_VERDICT)
private String dkimVerdict;
public static final String SERIALIZED_NAME_DMARC_VERDICT = "dmarcVerdict";
@SerializedName(SERIALIZED_NAME_DMARC_VERDICT)
private String dmarcVerdict;
public EmailAnalysis spamVerdict(String spamVerdict) {
this.spamVerdict = spamVerdict;
return this;
}
/**
* Verdict of spam ranking analysis
* @return spamVerdict
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Verdict of spam ranking analysis")
public String getSpamVerdict() {
return spamVerdict;
}
public void setSpamVerdict(String spamVerdict) {
this.spamVerdict = spamVerdict;
}
public EmailAnalysis virusVerdict(String virusVerdict) {
this.virusVerdict = virusVerdict;
return this;
}
/**
* Verdict of virus scan analysis
* @return virusVerdict
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Verdict of virus scan analysis")
public String getVirusVerdict() {
return virusVerdict;
}
public void setVirusVerdict(String virusVerdict) {
this.virusVerdict = virusVerdict;
}
public EmailAnalysis spfVerdict(String spfVerdict) {
this.spfVerdict = spfVerdict;
return this;
}
/**
* Verdict of Send Policy Framework record spoofing analysis
* @return spfVerdict
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Verdict of Send Policy Framework record spoofing analysis")
public String getSpfVerdict() {
return spfVerdict;
}
public void setSpfVerdict(String spfVerdict) {
this.spfVerdict = spfVerdict;
}
public EmailAnalysis dkimVerdict(String dkimVerdict) {
this.dkimVerdict = dkimVerdict;
return this;
}
/**
* Verdict of DomainKeys Identified Mail analysis
* @return dkimVerdict
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Verdict of DomainKeys Identified Mail analysis")
public String getDkimVerdict() {
return dkimVerdict;
}
public void setDkimVerdict(String dkimVerdict) {
this.dkimVerdict = dkimVerdict;
}
public EmailAnalysis dmarcVerdict(String dmarcVerdict) {
this.dmarcVerdict = dmarcVerdict;
return this;
}
/**
* Verdict of Domain-based Message Authentication Reporting and Conformance analysis
* @return dmarcVerdict
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Verdict of Domain-based Message Authentication Reporting and Conformance analysis")
public String getDmarcVerdict() {
return dmarcVerdict;
}
public void setDmarcVerdict(String dmarcVerdict) {
this.dmarcVerdict = dmarcVerdict;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailAnalysis emailAnalysis = (EmailAnalysis) o;
return Objects.equals(this.spamVerdict, emailAnalysis.spamVerdict) &&
Objects.equals(this.virusVerdict, emailAnalysis.virusVerdict) &&
Objects.equals(this.spfVerdict, emailAnalysis.spfVerdict) &&
Objects.equals(this.dkimVerdict, emailAnalysis.dkimVerdict) &&
Objects.equals(this.dmarcVerdict, emailAnalysis.dmarcVerdict);
}
@Override
public int hashCode() {
return Objects.hash(spamVerdict, virusVerdict, spfVerdict, dkimVerdict, dmarcVerdict);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailAnalysis {\n");
sb.append(" spamVerdict: ").append(toIndentedString(spamVerdict)).append("\n");
sb.append(" virusVerdict: ").append(toIndentedString(virusVerdict)).append("\n");
sb.append(" spfVerdict: ").append(toIndentedString(spfVerdict)).append("\n");
sb.append(" dkimVerdict: ").append(toIndentedString(dkimVerdict)).append("\n");
sb.append(" dmarcVerdict: ").append(toIndentedString(dmarcVerdict)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy