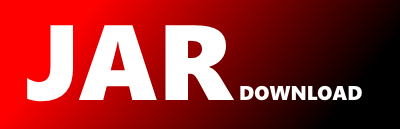
com.mailslurp.models.EmailFeatureOverview Maven / Gradle / Ivy
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.mailslurp.models.EmailFeatureFamilyStatistics;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* EmailFeatureOverview
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-05-03T02:14:29.400Z[GMT]")
public class EmailFeatureOverview {
/**
* Gets or Sets feature
*/
@JsonAdapter(FeatureEnum.Adapter.class)
public enum FeatureEnum {
AMP("amp"),
CSS_ACCENT_COLOR("css-accent-color"),
CSS_ALIGN_ITEMS("css-align-items"),
CSS_ANIMATION("css-animation"),
CSS_ASPECT_RATIO("css-aspect-ratio"),
CSS_AT_FONT_FACE("css-at-font-face"),
CSS_AT_IMPORT("css-at-import"),
CSS_AT_KEYFRAMES("css-at-keyframes"),
CSS_AT_MEDIA("css-at-media"),
CSS_AT_SUPPORTS("css-at-supports"),
CSS_BACKGROUND_BLEND_MODE("css-background-blend-mode"),
CSS_BACKGROUND_CLIP("css-background-clip"),
CSS_BACKGROUND_COLOR("css-background-color"),
CSS_BACKGROUND_IMAGE("css-background-image"),
CSS_BACKGROUND_ORIGIN("css-background-origin"),
CSS_BACKGROUND_POSITION("css-background-position"),
CSS_BACKGROUND_REPEAT("css-background-repeat"),
CSS_BACKGROUND_SIZE("css-background-size"),
CSS_BACKGROUND("css-background"),
CSS_BLOCK_INLINE_SIZE("css-block-inline-size"),
CSS_BORDER_IMAGE("css-border-image"),
CSS_BORDER_INLINE_BLOCK_INDIVIDUAL("css-border-inline-block-individual"),
CSS_BORDER_INLINE_BLOCK_LONGHAND("css-border-inline-block-longhand"),
CSS_BORDER_INLINE_BLOCK("css-border-inline-block"),
CSS_BORDER_RADIUS_LOGICAL("css-border-radius-logical"),
CSS_BORDER_RADIUS("css-border-radius"),
CSS_BORDER("css-border"),
CSS_BOX_SHADOW("css-box-shadow"),
CSS_BOX_SIZING("css-box-sizing"),
CSS_CAPTION_SIDE("css-caption-side"),
CSS_CLIP_PATH("css-clip-path"),
CSS_COLUMN_COUNT("css-column-count"),
CSS_COLUMN_LAYOUT_PROPERTIES("css-column-layout-properties"),
CSS_DIRECTION("css-direction"),
CSS_DISPLAY_FLEX("css-display-flex"),
CSS_DISPLAY_GRID("css-display-grid"),
CSS_DISPLAY_NONE("css-display-none"),
CSS_DISPLAY("css-display"),
CSS_FILTER("css-filter"),
CSS_FLEX_DIRECTION("css-flex-direction"),
CSS_FLEX_WRAP("css-flex-wrap"),
CSS_FLOAT("css-float"),
CSS_FONT_KERNING("css-font-kerning"),
CSS_FONT_WEIGHT("css-font-weight"),
CSS_FONT("css-font"),
CSS_GAP("css-gap"),
CSS_GRID_TEMPLATE("css-grid-template"),
CSS_HEIGHT("css-height"),
CSS_HYPHENS("css-hyphens"),
CSS_INLINE_SIZE("css-inline-size"),
CSS_JUSTIFY_CONTENT("css-justify-content"),
CSS_LEFT_RIGHT_TOP_BOTTOM("css-left-right-top-bottom"),
CSS_LETTER_SPACING("css-letter-spacing"),
CSS_LINE_HEIGHT("css-line-height"),
CSS_LIST_STYLE_IMAGE("css-list-style-image"),
CSS_LIST_STYLE_POSITION("css-list-style-position"),
CSS_LIST_STYLE_TYPE("css-list-style-type"),
CSS_LIST_STYLE("css-list-style"),
CSS_MARGIN_BLOCK_START_END("css-margin-block-start-end"),
CSS_MARGIN_INLINE_BLOCK("css-margin-inline-block"),
CSS_MARGIN_INLINE_START_END("css-margin-inline-start-end"),
CSS_MARGIN_INLINE("css-margin-inline"),
CSS_MARGIN("css-margin"),
CSS_MAX_BLOCK_SIZE("css-max-block-size"),
CSS_MAX_HEIGHT("css-max-height"),
CSS_MAX_WIDTH("css-max-width"),
CSS_MIN_HEIGHT("css-min-height"),
CSS_MIN_INLINE_SIZE("css-min-inline-size"),
CSS_MIN_WIDTH("css-min-width"),
CSS_MIX_BLEND_MODE("css-mix-blend-mode"),
CSS_OBJECT_FIT("css-object-fit"),
CSS_OBJECT_POSITION("css-object-position"),
CSS_OPACITY("css-opacity"),
CSS_OUTLINE_OFFSET("css-outline-offset"),
CSS_OUTLINE("css-outline"),
CSS_OVERFLOW_WRAP("css-overflow-wrap"),
CSS_OVERFLOW("css-overflow"),
CSS_PADDING_BLOCK_START_END("css-padding-block-start-end"),
CSS_PADDING_INLINE_BLOCK("css-padding-inline-block"),
CSS_PADDING_INLINE_START_END("css-padding-inline-start-end"),
CSS_PADDING("css-padding"),
CSS_POSITION("css-position"),
CSS_TAB_SIZE("css-tab-size"),
CSS_TABLE_LAYOUT("css-table-layout"),
CSS_TEXT_ALIGN_LAST("css-text-align-last"),
CSS_TEXT_ALIGN("css-text-align"),
CSS_TEXT_DECORATION_COLOR("css-text-decoration-color"),
CSS_TEXT_DECORATION_THICKNESS("css-text-decoration-thickness"),
CSS_TEXT_DECORATION("css-text-decoration"),
CSS_TEXT_EMPHASIS_POSITION("css-text-emphasis-position"),
CSS_TEXT_EMPHASIS("css-text-emphasis"),
CSS_TEXT_INDENT("css-text-indent"),
CSS_TEXT_OVERFLOW("css-text-overflow"),
CSS_TEXT_SHADOW("css-text-shadow"),
CSS_TEXT_TRANSFORM("css-text-transform"),
CSS_TEXT_UNDERLINE_OFFSET("css-text-underline-offset"),
CSS_TRANSFORM("css-transform"),
CSS_VERTICAL_ALIGN("css-vertical-align"),
CSS_VISIBILITY("css-visibility"),
CSS_WHITE_SPACE("css-white-space"),
CSS_WIDTH("css-width"),
CSS_WORD_BREAK("css-word-break"),
CSS_WRITING_MODE("css-writing-mode"),
CSS_Z_INDEX("css-z-index"),
HTML_ABBR("html-abbr"),
HTML_ADDRESS("html-address"),
HTML_ALIGN("html-align"),
HTML_ANCHOR_LINKS("html-anchor-links"),
HTML_ARIA_DESCRIBEDBY("html-aria-describedby"),
HTML_ARIA_HIDDEN("html-aria-hidden"),
HTML_ARIA_LABEL("html-aria-label"),
HTML_ARIA_LABELLEDBY("html-aria-labelledby"),
HTML_ARIA_LIVE("html-aria-live"),
HTML_AUDIO("html-audio"),
HTML_BACKGROUND("html-background"),
HTML_BASE("html-base"),
HTML_BLOCKQUOTE("html-blockquote"),
HTML_BODY("html-body"),
HTML_BUTTON_RESET("html-button-reset"),
HTML_BUTTON_SUBMIT("html-button-submit"),
HTML_CODE("html-code"),
HTML_DEL("html-del"),
HTML_DFN("html-dfn"),
HTML_DIALOG("html-dialog"),
HTML_DIR("html-dir"),
HTML_DIV("html-div"),
HTML_DOCTYPE("html-doctype"),
HTML_FORM("html-form"),
HTML_H1_H6("html-h1-h6"),
HTML_HEIGHT("html-height"),
HTML_IMAGE_MAPS("html-image-maps"),
HTML_INPUT_CHECKBOX("html-input-checkbox"),
HTML_INPUT_HIDDEN("html-input-hidden"),
HTML_INPUT_RADIO("html-input-radio"),
HTML_INPUT_RESET("html-input-reset"),
HTML_INPUT_SUBMIT("html-input-submit"),
HTML_INPUT_TEXT("html-input-text"),
HTML_LANG("html-lang"),
HTML_LINK("html-link"),
HTML_LISTS("html-lists"),
HTML_LOADING_ATTRIBUTE("html-loading-attribute"),
HTML_MAILTO_LINKS("html-mailto-links"),
HTML_MARQUEE("html-marquee"),
HTML_METER("html-meter"),
HTML_OBJECT("html-object"),
HTML_P("html-p"),
HTML_PICTURE("html-picture"),
HTML_PRE("html-pre"),
HTML_PROGRESS("html-progress"),
HTML_REQUIRED("html-required"),
HTML_ROLE("html-role"),
HTML_RP("html-rp"),
HTML_RT("html-rt"),
HTML_RUBY("html-ruby"),
HTML_SELECT("html-select"),
HTML_SEMANTICS("html-semantics"),
HTML_SMALL("html-small"),
HTML_SPAN("html-span"),
HTML_SRCSET("html-srcset"),
HTML_STRIKE("html-strike"),
HTML_STRONG("html-strong"),
HTML_STYLE("html-style"),
HTML_SVG("html-svg"),
HTML_TABLE("html-table"),
HTML_TARGET("html-target"),
HTML_TEXTAREA("html-textarea"),
HTML_VALIGN("html-valign"),
HTML_VIDEO("html-video"),
HTML_WBR("html-wbr"),
HTML_WIDTH("html-width"),
IMAGE_AVIF("image-avif"),
IMAGE_BASE64("image-base64"),
IMAGE_BMP("image-bmp"),
IMAGE_GIF("image-gif"),
IMAGE_ICO("image-ico"),
IMAGE_JPG("image-jpg"),
IMAGE_PNG("image-png"),
IMAGE_SVG("image-svg"),
IMAGE_WEBP("image-webp"),
UNSUPPORTED("unsupported");
private String value;
FeatureEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static FeatureEnum fromValue(String value) {
for (FeatureEnum b : FeatureEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final FeatureEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public FeatureEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return FeatureEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_FEATURE = "feature";
@SerializedName(SERIALIZED_NAME_FEATURE)
private FeatureEnum feature;
public static final String SERIALIZED_NAME_TITLE = "title";
@SerializedName(SERIALIZED_NAME_TITLE)
private String title;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
/**
* Gets or Sets category
*/
@JsonAdapter(CategoryEnum.Adapter.class)
public enum CategoryEnum {
CSS("css"),
HTML("html"),
IMAGE("image"),
OTHERS("others");
private String value;
CategoryEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CategoryEnum fromValue(String value) {
for (CategoryEnum b : CategoryEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CategoryEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CategoryEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CategoryEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private CategoryEnum category;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_NOTES_NUMBERS = "notesNumbers";
@SerializedName(SERIALIZED_NAME_NOTES_NUMBERS)
private Map notesNumbers = null;
public static final String SERIALIZED_NAME_FEATURE_STATISTICS = "featureStatistics";
@SerializedName(SERIALIZED_NAME_FEATURE_STATISTICS)
private List featureStatistics = null;
/**
* Gets or Sets statuses
*/
@JsonAdapter(StatusesEnum.Adapter.class)
public enum StatusesEnum {
SUPPORTED("SUPPORTED"),
PARTIAL("PARTIAL"),
NOT_SUPPORTED("NOT_SUPPORTED"),
UNKNOWN("UNKNOWN");
private String value;
StatusesEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusesEnum fromValue(String value) {
for (StatusesEnum b : StatusesEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusesEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusesEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusesEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATUSES = "statuses";
@SerializedName(SERIALIZED_NAME_STATUSES)
private List statuses = new ArrayList<>();
public EmailFeatureOverview feature(FeatureEnum feature) {
this.feature = feature;
return this;
}
/**
* Get feature
* @return feature
**/
@ApiModelProperty(required = true, value = "")
public FeatureEnum getFeature() {
return feature;
}
public void setFeature(FeatureEnum feature) {
this.feature = feature;
}
public EmailFeatureOverview title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public EmailFeatureOverview description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public EmailFeatureOverview category(CategoryEnum category) {
this.category = category;
return this;
}
/**
* Get category
* @return category
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CategoryEnum getCategory() {
return category;
}
public void setCategory(CategoryEnum category) {
this.category = category;
}
public EmailFeatureOverview notes(String notes) {
this.notes = notes;
return this;
}
/**
* Get notes
* @return notes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public EmailFeatureOverview notesNumbers(Map notesNumbers) {
this.notesNumbers = notesNumbers;
return this;
}
public EmailFeatureOverview putNotesNumbersItem(String key, String notesNumbersItem) {
if (this.notesNumbers == null) {
this.notesNumbers = new HashMap<>();
}
this.notesNumbers.put(key, notesNumbersItem);
return this;
}
/**
* Get notesNumbers
* @return notesNumbers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Map getNotesNumbers() {
return notesNumbers;
}
public void setNotesNumbers(Map notesNumbers) {
this.notesNumbers = notesNumbers;
}
public EmailFeatureOverview featureStatistics(List featureStatistics) {
this.featureStatistics = featureStatistics;
return this;
}
public EmailFeatureOverview addFeatureStatisticsItem(EmailFeatureFamilyStatistics featureStatisticsItem) {
if (this.featureStatistics == null) {
this.featureStatistics = new ArrayList<>();
}
this.featureStatistics.add(featureStatisticsItem);
return this;
}
/**
* Get featureStatistics
* @return featureStatistics
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getFeatureStatistics() {
return featureStatistics;
}
public void setFeatureStatistics(List featureStatistics) {
this.featureStatistics = featureStatistics;
}
public EmailFeatureOverview statuses(List statuses) {
this.statuses = statuses;
return this;
}
public EmailFeatureOverview addStatusesItem(StatusesEnum statusesItem) {
this.statuses.add(statusesItem);
return this;
}
/**
* Get statuses
* @return statuses
**/
@ApiModelProperty(required = true, value = "")
public List getStatuses() {
return statuses;
}
public void setStatuses(List statuses) {
this.statuses = statuses;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailFeatureOverview emailFeatureOverview = (EmailFeatureOverview) o;
return Objects.equals(this.feature, emailFeatureOverview.feature) &&
Objects.equals(this.title, emailFeatureOverview.title) &&
Objects.equals(this.description, emailFeatureOverview.description) &&
Objects.equals(this.category, emailFeatureOverview.category) &&
Objects.equals(this.notes, emailFeatureOverview.notes) &&
Objects.equals(this.notesNumbers, emailFeatureOverview.notesNumbers) &&
Objects.equals(this.featureStatistics, emailFeatureOverview.featureStatistics) &&
Objects.equals(this.statuses, emailFeatureOverview.statuses);
}
@Override
public int hashCode() {
return Objects.hash(feature, title, description, category, notes, notesNumbers, featureStatistics, statuses);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailFeatureOverview {\n");
sb.append(" feature: ").append(toIndentedString(feature)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" notesNumbers: ").append(toIndentedString(notesNumbers)).append("\n");
sb.append(" featureStatistics: ").append(toIndentedString(featureStatistics)).append("\n");
sb.append(" statuses: ").append(toIndentedString(statuses)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy