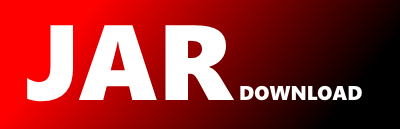
com.mailslurp.models.MissedEmailDto Maven / Gradle / Ivy
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
/**
* Missed email
*/
@ApiModel(description = "Missed email")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-05-03T02:14:29.400Z[GMT]")
public class MissedEmailDto {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private UUID id;
public static final String SERIALIZED_NAME_USER_ID = "userId";
@SerializedName(SERIALIZED_NAME_USER_ID)
private UUID userId;
public static final String SERIALIZED_NAME_SUBJECT = "subject";
@SerializedName(SERIALIZED_NAME_SUBJECT)
private String subject;
public static final String SERIALIZED_NAME_BODY_EXCERPT = "bodyExcerpt";
@SerializedName(SERIALIZED_NAME_BODY_EXCERPT)
private String bodyExcerpt;
public static final String SERIALIZED_NAME_ATTACHMENT_COUNT = "attachmentCount";
@SerializedName(SERIALIZED_NAME_ATTACHMENT_COUNT)
private Integer attachmentCount;
public static final String SERIALIZED_NAME_FROM = "from";
@SerializedName(SERIALIZED_NAME_FROM)
private String from;
public static final String SERIALIZED_NAME_RAW_URL = "rawUrl";
@SerializedName(SERIALIZED_NAME_RAW_URL)
private String rawUrl;
public static final String SERIALIZED_NAME_RAW_KEY = "rawKey";
@SerializedName(SERIALIZED_NAME_RAW_KEY)
private String rawKey;
public static final String SERIALIZED_NAME_RAW_BUCKET = "rawBucket";
@SerializedName(SERIALIZED_NAME_RAW_BUCKET)
private String rawBucket;
public static final String SERIALIZED_NAME_CAN_RESTORE = "canRestore";
@SerializedName(SERIALIZED_NAME_CAN_RESTORE)
private Boolean canRestore;
public static final String SERIALIZED_NAME_TO = "to";
@SerializedName(SERIALIZED_NAME_TO)
private List to = new ArrayList<>();
public static final String SERIALIZED_NAME_CC = "cc";
@SerializedName(SERIALIZED_NAME_CC)
private List cc = new ArrayList<>();
public static final String SERIALIZED_NAME_BCC = "bcc";
@SerializedName(SERIALIZED_NAME_BCC)
private List bcc = new ArrayList<>();
public static final String SERIALIZED_NAME_INBOX_IDS = "inboxIds";
@SerializedName(SERIALIZED_NAME_INBOX_IDS)
private List inboxIds = new ArrayList<>();
public static final String SERIALIZED_NAME_CREATED_AT = "createdAt";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private OffsetDateTime createdAt;
public static final String SERIALIZED_NAME_UPDATED_AT = "updatedAt";
@SerializedName(SERIALIZED_NAME_UPDATED_AT)
private OffsetDateTime updatedAt;
public MissedEmailDto id(UUID id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(required = true, value = "")
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public MissedEmailDto userId(UUID userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public UUID getUserId() {
return userId;
}
public void setUserId(UUID userId) {
this.userId = userId;
}
public MissedEmailDto subject(String subject) {
this.subject = subject;
return this;
}
/**
* Get subject
* @return subject
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public MissedEmailDto bodyExcerpt(String bodyExcerpt) {
this.bodyExcerpt = bodyExcerpt;
return this;
}
/**
* Get bodyExcerpt
* @return bodyExcerpt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getBodyExcerpt() {
return bodyExcerpt;
}
public void setBodyExcerpt(String bodyExcerpt) {
this.bodyExcerpt = bodyExcerpt;
}
public MissedEmailDto attachmentCount(Integer attachmentCount) {
this.attachmentCount = attachmentCount;
return this;
}
/**
* Get attachmentCount
* @return attachmentCount
**/
@ApiModelProperty(required = true, value = "")
public Integer getAttachmentCount() {
return attachmentCount;
}
public void setAttachmentCount(Integer attachmentCount) {
this.attachmentCount = attachmentCount;
}
public MissedEmailDto from(String from) {
this.from = from;
return this;
}
/**
* Get from
* @return from
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public MissedEmailDto rawUrl(String rawUrl) {
this.rawUrl = rawUrl;
return this;
}
/**
* Get rawUrl
* @return rawUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getRawUrl() {
return rawUrl;
}
public void setRawUrl(String rawUrl) {
this.rawUrl = rawUrl;
}
public MissedEmailDto rawKey(String rawKey) {
this.rawKey = rawKey;
return this;
}
/**
* Get rawKey
* @return rawKey
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getRawKey() {
return rawKey;
}
public void setRawKey(String rawKey) {
this.rawKey = rawKey;
}
public MissedEmailDto rawBucket(String rawBucket) {
this.rawBucket = rawBucket;
return this;
}
/**
* Get rawBucket
* @return rawBucket
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getRawBucket() {
return rawBucket;
}
public void setRawBucket(String rawBucket) {
this.rawBucket = rawBucket;
}
public MissedEmailDto canRestore(Boolean canRestore) {
this.canRestore = canRestore;
return this;
}
/**
* Get canRestore
* @return canRestore
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getCanRestore() {
return canRestore;
}
public void setCanRestore(Boolean canRestore) {
this.canRestore = canRestore;
}
public MissedEmailDto to(List to) {
this.to = to;
return this;
}
public MissedEmailDto addToItem(String toItem) {
this.to.add(toItem);
return this;
}
/**
* Get to
* @return to
**/
@ApiModelProperty(required = true, value = "")
public List getTo() {
return to;
}
public void setTo(List to) {
this.to = to;
}
public MissedEmailDto cc(List cc) {
this.cc = cc;
return this;
}
public MissedEmailDto addCcItem(String ccItem) {
this.cc.add(ccItem);
return this;
}
/**
* Get cc
* @return cc
**/
@ApiModelProperty(required = true, value = "")
public List getCc() {
return cc;
}
public void setCc(List cc) {
this.cc = cc;
}
public MissedEmailDto bcc(List bcc) {
this.bcc = bcc;
return this;
}
public MissedEmailDto addBccItem(String bccItem) {
this.bcc.add(bccItem);
return this;
}
/**
* Get bcc
* @return bcc
**/
@ApiModelProperty(required = true, value = "")
public List getBcc() {
return bcc;
}
public void setBcc(List bcc) {
this.bcc = bcc;
}
public MissedEmailDto inboxIds(List inboxIds) {
this.inboxIds = inboxIds;
return this;
}
public MissedEmailDto addInboxIdsItem(UUID inboxIdsItem) {
this.inboxIds.add(inboxIdsItem);
return this;
}
/**
* Get inboxIds
* @return inboxIds
**/
@ApiModelProperty(required = true, value = "")
public List getInboxIds() {
return inboxIds;
}
public void setInboxIds(List inboxIds) {
this.inboxIds = inboxIds;
}
public MissedEmailDto createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Get createdAt
* @return createdAt
**/
@ApiModelProperty(required = true, value = "")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public MissedEmailDto updatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
**/
@ApiModelProperty(required = true, value = "")
public OffsetDateTime getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MissedEmailDto missedEmailDto = (MissedEmailDto) o;
return Objects.equals(this.id, missedEmailDto.id) &&
Objects.equals(this.userId, missedEmailDto.userId) &&
Objects.equals(this.subject, missedEmailDto.subject) &&
Objects.equals(this.bodyExcerpt, missedEmailDto.bodyExcerpt) &&
Objects.equals(this.attachmentCount, missedEmailDto.attachmentCount) &&
Objects.equals(this.from, missedEmailDto.from) &&
Objects.equals(this.rawUrl, missedEmailDto.rawUrl) &&
Objects.equals(this.rawKey, missedEmailDto.rawKey) &&
Objects.equals(this.rawBucket, missedEmailDto.rawBucket) &&
Objects.equals(this.canRestore, missedEmailDto.canRestore) &&
Objects.equals(this.to, missedEmailDto.to) &&
Objects.equals(this.cc, missedEmailDto.cc) &&
Objects.equals(this.bcc, missedEmailDto.bcc) &&
Objects.equals(this.inboxIds, missedEmailDto.inboxIds) &&
Objects.equals(this.createdAt, missedEmailDto.createdAt) &&
Objects.equals(this.updatedAt, missedEmailDto.updatedAt);
}
@Override
public int hashCode() {
return Objects.hash(id, userId, subject, bodyExcerpt, attachmentCount, from, rawUrl, rawKey, rawBucket, canRestore, to, cc, bcc, inboxIds, createdAt, updatedAt);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MissedEmailDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" bodyExcerpt: ").append(toIndentedString(bodyExcerpt)).append("\n");
sb.append(" attachmentCount: ").append(toIndentedString(attachmentCount)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" rawUrl: ").append(toIndentedString(rawUrl)).append("\n");
sb.append(" rawKey: ").append(toIndentedString(rawKey)).append("\n");
sb.append(" rawBucket: ").append(toIndentedString(rawBucket)).append("\n");
sb.append(" canRestore: ").append(toIndentedString(canRestore)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append(" cc: ").append(toIndentedString(cc)).append("\n");
sb.append(" bcc: ").append(toIndentedString(bcc)).append("\n");
sb.append(" inboxIds: ").append(toIndentedString(inboxIds)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy