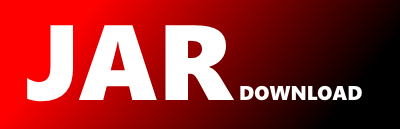
com.mailslurp.models.WebhookDto Maven / Gradle / Ivy
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.mailslurp.models.WebhookHeaders;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.UUID;
/**
* Representation of a webhook for an inbox. The URL specified will be using by MailSlurp whenever an email is received by the attached inbox. A webhook entity should have a URL that points to your server. Your server should accept HTTP/S POST requests and return a success 200. MailSlurp will retry your webhooks if they fail. See https://java.api.mailslurp.com/schemas/webhook-payload for the payload schema.
*/
@ApiModel(description = "Representation of a webhook for an inbox. The URL specified will be using by MailSlurp whenever an email is received by the attached inbox. A webhook entity should have a URL that points to your server. Your server should accept HTTP/S POST requests and return a success 200. MailSlurp will retry your webhooks if they fail. See https://java.api.mailslurp.com/schemas/webhook-payload for the payload schema.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-05-03T02:14:29.400Z[GMT]")
public class WebhookDto {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private UUID id;
public static final String SERIALIZED_NAME_USER_ID = "userId";
@SerializedName(SERIALIZED_NAME_USER_ID)
private UUID userId;
public static final String SERIALIZED_NAME_BASIC_AUTH = "basicAuth";
@SerializedName(SERIALIZED_NAME_BASIC_AUTH)
private Boolean basicAuth;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PHONE_ID = "phoneId";
@SerializedName(SERIALIZED_NAME_PHONE_ID)
private UUID phoneId;
public static final String SERIALIZED_NAME_INBOX_ID = "inboxId";
@SerializedName(SERIALIZED_NAME_INBOX_ID)
private UUID inboxId;
public static final String SERIALIZED_NAME_REQUEST_BODY_TEMPLATE = "requestBodyTemplate";
@SerializedName(SERIALIZED_NAME_REQUEST_BODY_TEMPLATE)
private String requestBodyTemplate;
public static final String SERIALIZED_NAME_URL = "url";
@SerializedName(SERIALIZED_NAME_URL)
private String url;
/**
* HTTP method that your server endpoint must listen for
*/
@JsonAdapter(MethodEnum.Adapter.class)
public enum MethodEnum {
POST("POST"),
DELETE("DELETE"),
GET("GET"),
PUT("PUT"),
PATCH("PATCH"),
HEAD("HEAD"),
OPTIONS("OPTIONS"),
TRACE("TRACE");
private String value;
MethodEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static MethodEnum fromValue(String value) {
for (MethodEnum b : MethodEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final MethodEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public MethodEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return MethodEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_METHOD = "method";
@SerializedName(SERIALIZED_NAME_METHOD)
private MethodEnum method;
public static final String SERIALIZED_NAME_PAYLOAD_JSON_SCHEMA = "payloadJsonSchema";
@SerializedName(SERIALIZED_NAME_PAYLOAD_JSON_SCHEMA)
private String payloadJsonSchema;
public static final String SERIALIZED_NAME_CREATED_AT = "createdAt";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private OffsetDateTime createdAt;
public static final String SERIALIZED_NAME_UPDATED_AT = "updatedAt";
@SerializedName(SERIALIZED_NAME_UPDATED_AT)
private OffsetDateTime updatedAt;
/**
* Webhook trigger event name
*/
@JsonAdapter(EventNameEnum.Adapter.class)
public enum EventNameEnum {
EMAIL_RECEIVED("EMAIL_RECEIVED"),
NEW_EMAIL("NEW_EMAIL"),
NEW_CONTACT("NEW_CONTACT"),
NEW_ATTACHMENT("NEW_ATTACHMENT"),
EMAIL_OPENED("EMAIL_OPENED"),
EMAIL_READ("EMAIL_READ"),
DELIVERY_STATUS("DELIVERY_STATUS"),
BOUNCE("BOUNCE"),
BOUNCE_RECIPIENT("BOUNCE_RECIPIENT"),
NEW_SMS("NEW_SMS");
private String value;
EventNameEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static EventNameEnum fromValue(String value) {
for (EventNameEnum b : EventNameEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final EventNameEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public EventNameEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return EventNameEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_EVENT_NAME = "eventName";
@SerializedName(SERIALIZED_NAME_EVENT_NAME)
private EventNameEnum eventName;
public static final String SERIALIZED_NAME_REQUEST_HEADERS = "requestHeaders";
@SerializedName(SERIALIZED_NAME_REQUEST_HEADERS)
private WebhookHeaders requestHeaders;
public static final String SERIALIZED_NAME_IGNORE_INSECURE_SSL_CERTIFICATES = "ignoreInsecureSslCertificates";
@SerializedName(SERIALIZED_NAME_IGNORE_INSECURE_SSL_CERTIFICATES)
private Boolean ignoreInsecureSslCertificates;
public static final String SERIALIZED_NAME_USE_STATIC_IP_RANGE = "useStaticIpRange";
@SerializedName(SERIALIZED_NAME_USE_STATIC_IP_RANGE)
private Boolean useStaticIpRange;
public WebhookDto id(UUID id) {
this.id = id;
return this;
}
/**
* ID of the Webhook
* @return id
**/
@ApiModelProperty(required = true, value = "ID of the Webhook")
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public WebhookDto userId(UUID userId) {
this.userId = userId;
return this;
}
/**
* User ID of the Webhook
* @return userId
**/
@ApiModelProperty(required = true, value = "User ID of the Webhook")
public UUID getUserId() {
return userId;
}
public void setUserId(UUID userId) {
this.userId = userId;
}
public WebhookDto basicAuth(Boolean basicAuth) {
this.basicAuth = basicAuth;
return this;
}
/**
* Does webhook expect basic authentication? If true it means you created this webhook with a username and password. MailSlurp will use these in the URL to authenticate itself.
* @return basicAuth
**/
@ApiModelProperty(required = true, value = "Does webhook expect basic authentication? If true it means you created this webhook with a username and password. MailSlurp will use these in the URL to authenticate itself.")
public Boolean getBasicAuth() {
return basicAuth;
}
public void setBasicAuth(Boolean basicAuth) {
this.basicAuth = basicAuth;
}
public WebhookDto name(String name) {
this.name = name;
return this;
}
/**
* Name of the webhook
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the webhook")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public WebhookDto phoneId(UUID phoneId) {
this.phoneId = phoneId;
return this;
}
/**
* The phoneNumberId that the Webhook will be triggered by. If null then webhook triggered at account level or inbox level if inboxId set
* @return phoneId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The phoneNumberId that the Webhook will be triggered by. If null then webhook triggered at account level or inbox level if inboxId set")
public UUID getPhoneId() {
return phoneId;
}
public void setPhoneId(UUID phoneId) {
this.phoneId = phoneId;
}
public WebhookDto inboxId(UUID inboxId) {
this.inboxId = inboxId;
return this;
}
/**
* The inbox that the Webhook will be triggered by. If null then webhook triggered at account level or phone level if phoneId set
* @return inboxId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The inbox that the Webhook will be triggered by. If null then webhook triggered at account level or phone level if phoneId set")
public UUID getInboxId() {
return inboxId;
}
public void setInboxId(UUID inboxId) {
this.inboxId = inboxId;
}
public WebhookDto requestBodyTemplate(String requestBodyTemplate) {
this.requestBodyTemplate = requestBodyTemplate;
return this;
}
/**
* Request body template for HTTP request that will be sent for the webhook. Use Moustache style template variables to insert values from the original event payload.
* @return requestBodyTemplate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Request body template for HTTP request that will be sent for the webhook. Use Moustache style template variables to insert values from the original event payload.")
public String getRequestBodyTemplate() {
return requestBodyTemplate;
}
public void setRequestBodyTemplate(String requestBodyTemplate) {
this.requestBodyTemplate = requestBodyTemplate;
}
public WebhookDto url(String url) {
this.url = url;
return this;
}
/**
* URL of your server that the webhook will be sent to. The schema of the JSON that is sent is described by the payloadJsonSchema.
* @return url
**/
@ApiModelProperty(required = true, value = "URL of your server that the webhook will be sent to. The schema of the JSON that is sent is described by the payloadJsonSchema.")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public WebhookDto method(MethodEnum method) {
this.method = method;
return this;
}
/**
* HTTP method that your server endpoint must listen for
* @return method
**/
@ApiModelProperty(required = true, value = "HTTP method that your server endpoint must listen for")
public MethodEnum getMethod() {
return method;
}
public void setMethod(MethodEnum method) {
this.method = method;
}
public WebhookDto payloadJsonSchema(String payloadJsonSchema) {
this.payloadJsonSchema = payloadJsonSchema;
return this;
}
/**
* Deprecated. Fetch JSON Schema for webhook using the getJsonSchemaForWebhookPayload method
* @return payloadJsonSchema
**/
@ApiModelProperty(required = true, value = "Deprecated. Fetch JSON Schema for webhook using the getJsonSchemaForWebhookPayload method")
public String getPayloadJsonSchema() {
return payloadJsonSchema;
}
public void setPayloadJsonSchema(String payloadJsonSchema) {
this.payloadJsonSchema = payloadJsonSchema;
}
public WebhookDto createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* When the webhook was created
* @return createdAt
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "When the webhook was created")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public WebhookDto updatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
**/
@ApiModelProperty(required = true, value = "")
public OffsetDateTime getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
}
public WebhookDto eventName(EventNameEnum eventName) {
this.eventName = eventName;
return this;
}
/**
* Webhook trigger event name
* @return eventName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Webhook trigger event name")
public EventNameEnum getEventName() {
return eventName;
}
public void setEventName(EventNameEnum eventName) {
this.eventName = eventName;
}
public WebhookDto requestHeaders(WebhookHeaders requestHeaders) {
this.requestHeaders = requestHeaders;
return this;
}
/**
* Get requestHeaders
* @return requestHeaders
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public WebhookHeaders getRequestHeaders() {
return requestHeaders;
}
public void setRequestHeaders(WebhookHeaders requestHeaders) {
this.requestHeaders = requestHeaders;
}
public WebhookDto ignoreInsecureSslCertificates(Boolean ignoreInsecureSslCertificates) {
this.ignoreInsecureSslCertificates = ignoreInsecureSslCertificates;
return this;
}
/**
* Should notifier ignore insecure SSL certificates
* @return ignoreInsecureSslCertificates
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Should notifier ignore insecure SSL certificates")
public Boolean getIgnoreInsecureSslCertificates() {
return ignoreInsecureSslCertificates;
}
public void setIgnoreInsecureSslCertificates(Boolean ignoreInsecureSslCertificates) {
this.ignoreInsecureSslCertificates = ignoreInsecureSslCertificates;
}
public WebhookDto useStaticIpRange(Boolean useStaticIpRange) {
this.useStaticIpRange = useStaticIpRange;
return this;
}
/**
* Should notifier use static IP range when sending webhook payload
* @return useStaticIpRange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Should notifier use static IP range when sending webhook payload")
public Boolean getUseStaticIpRange() {
return useStaticIpRange;
}
public void setUseStaticIpRange(Boolean useStaticIpRange) {
this.useStaticIpRange = useStaticIpRange;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WebhookDto webhookDto = (WebhookDto) o;
return Objects.equals(this.id, webhookDto.id) &&
Objects.equals(this.userId, webhookDto.userId) &&
Objects.equals(this.basicAuth, webhookDto.basicAuth) &&
Objects.equals(this.name, webhookDto.name) &&
Objects.equals(this.phoneId, webhookDto.phoneId) &&
Objects.equals(this.inboxId, webhookDto.inboxId) &&
Objects.equals(this.requestBodyTemplate, webhookDto.requestBodyTemplate) &&
Objects.equals(this.url, webhookDto.url) &&
Objects.equals(this.method, webhookDto.method) &&
Objects.equals(this.payloadJsonSchema, webhookDto.payloadJsonSchema) &&
Objects.equals(this.createdAt, webhookDto.createdAt) &&
Objects.equals(this.updatedAt, webhookDto.updatedAt) &&
Objects.equals(this.eventName, webhookDto.eventName) &&
Objects.equals(this.requestHeaders, webhookDto.requestHeaders) &&
Objects.equals(this.ignoreInsecureSslCertificates, webhookDto.ignoreInsecureSslCertificates) &&
Objects.equals(this.useStaticIpRange, webhookDto.useStaticIpRange);
}
@Override
public int hashCode() {
return Objects.hash(id, userId, basicAuth, name, phoneId, inboxId, requestBodyTemplate, url, method, payloadJsonSchema, createdAt, updatedAt, eventName, requestHeaders, ignoreInsecureSslCertificates, useStaticIpRange);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WebhookDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" basicAuth: ").append(toIndentedString(basicAuth)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" phoneId: ").append(toIndentedString(phoneId)).append("\n");
sb.append(" inboxId: ").append(toIndentedString(inboxId)).append("\n");
sb.append(" requestBodyTemplate: ").append(toIndentedString(requestBodyTemplate)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" method: ").append(toIndentedString(method)).append("\n");
sb.append(" payloadJsonSchema: ").append(toIndentedString(payloadJsonSchema)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" eventName: ").append(toIndentedString(eventName)).append("\n");
sb.append(" requestHeaders: ").append(toIndentedString(requestHeaders)).append("\n");
sb.append(" ignoreInsecureSslCertificates: ").append(toIndentedString(ignoreInsecureSslCertificates)).append("\n");
sb.append(" useStaticIpRange: ").append(toIndentedString(useStaticIpRange)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy