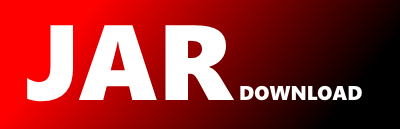
com.mailslurp.models.ConnectorSyncRequestResultExceptionCauseStackTrace Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mailslurp-client-java Show documentation
Show all versions of mailslurp-client-java Show documentation
Official MailSlurp email API - create real inboxes then send and receive emails and attachments from tests and code.
The newest version!
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* ConnectorSyncRequestResultExceptionCauseStackTrace
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-03T06:03:24.997Z[GMT]")
public class ConnectorSyncRequestResultExceptionCauseStackTrace {
public static final String SERIALIZED_NAME_CLASS_LOADER_NAME = "classLoaderName";
@SerializedName(SERIALIZED_NAME_CLASS_LOADER_NAME)
private String classLoaderName;
public static final String SERIALIZED_NAME_MODULE_NAME = "moduleName";
@SerializedName(SERIALIZED_NAME_MODULE_NAME)
private String moduleName;
public static final String SERIALIZED_NAME_MODULE_VERSION = "moduleVersion";
@SerializedName(SERIALIZED_NAME_MODULE_VERSION)
private String moduleVersion;
public static final String SERIALIZED_NAME_METHOD_NAME = "methodName";
@SerializedName(SERIALIZED_NAME_METHOD_NAME)
private String methodName;
public static final String SERIALIZED_NAME_FILE_NAME = "fileName";
@SerializedName(SERIALIZED_NAME_FILE_NAME)
private String fileName;
public static final String SERIALIZED_NAME_LINE_NUMBER = "lineNumber";
@SerializedName(SERIALIZED_NAME_LINE_NUMBER)
private Integer lineNumber;
public static final String SERIALIZED_NAME_CLASS_NAME = "className";
@SerializedName(SERIALIZED_NAME_CLASS_NAME)
private String className;
public static final String SERIALIZED_NAME_NATIVE_METHOD = "nativeMethod";
@SerializedName(SERIALIZED_NAME_NATIVE_METHOD)
private Boolean nativeMethod;
public ConnectorSyncRequestResultExceptionCauseStackTrace classLoaderName(String classLoaderName) {
this.classLoaderName = classLoaderName;
return this;
}
/**
* Get classLoaderName
* @return classLoaderName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getClassLoaderName() {
return classLoaderName;
}
public void setClassLoaderName(String classLoaderName) {
this.classLoaderName = classLoaderName;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace moduleName(String moduleName) {
this.moduleName = moduleName;
return this;
}
/**
* Get moduleName
* @return moduleName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getModuleName() {
return moduleName;
}
public void setModuleName(String moduleName) {
this.moduleName = moduleName;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace moduleVersion(String moduleVersion) {
this.moduleVersion = moduleVersion;
return this;
}
/**
* Get moduleVersion
* @return moduleVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getModuleVersion() {
return moduleVersion;
}
public void setModuleVersion(String moduleVersion) {
this.moduleVersion = moduleVersion;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace methodName(String methodName) {
this.methodName = methodName;
return this;
}
/**
* Get methodName
* @return methodName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getMethodName() {
return methodName;
}
public void setMethodName(String methodName) {
this.methodName = methodName;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace fileName(String fileName) {
this.fileName = fileName;
return this;
}
/**
* Get fileName
* @return fileName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace lineNumber(Integer lineNumber) {
this.lineNumber = lineNumber;
return this;
}
/**
* Get lineNumber
* @return lineNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getLineNumber() {
return lineNumber;
}
public void setLineNumber(Integer lineNumber) {
this.lineNumber = lineNumber;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace className(String className) {
this.className = className;
return this;
}
/**
* Get className
* @return className
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
public ConnectorSyncRequestResultExceptionCauseStackTrace nativeMethod(Boolean nativeMethod) {
this.nativeMethod = nativeMethod;
return this;
}
/**
* Get nativeMethod
* @return nativeMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getNativeMethod() {
return nativeMethod;
}
public void setNativeMethod(Boolean nativeMethod) {
this.nativeMethod = nativeMethod;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConnectorSyncRequestResultExceptionCauseStackTrace connectorSyncRequestResultExceptionCauseStackTrace = (ConnectorSyncRequestResultExceptionCauseStackTrace) o;
return Objects.equals(this.classLoaderName, connectorSyncRequestResultExceptionCauseStackTrace.classLoaderName) &&
Objects.equals(this.moduleName, connectorSyncRequestResultExceptionCauseStackTrace.moduleName) &&
Objects.equals(this.moduleVersion, connectorSyncRequestResultExceptionCauseStackTrace.moduleVersion) &&
Objects.equals(this.methodName, connectorSyncRequestResultExceptionCauseStackTrace.methodName) &&
Objects.equals(this.fileName, connectorSyncRequestResultExceptionCauseStackTrace.fileName) &&
Objects.equals(this.lineNumber, connectorSyncRequestResultExceptionCauseStackTrace.lineNumber) &&
Objects.equals(this.className, connectorSyncRequestResultExceptionCauseStackTrace.className) &&
Objects.equals(this.nativeMethod, connectorSyncRequestResultExceptionCauseStackTrace.nativeMethod);
}
@Override
public int hashCode() {
return Objects.hash(classLoaderName, moduleName, moduleVersion, methodName, fileName, lineNumber, className, nativeMethod);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConnectorSyncRequestResultExceptionCauseStackTrace {\n");
sb.append(" classLoaderName: ").append(toIndentedString(classLoaderName)).append("\n");
sb.append(" moduleName: ").append(toIndentedString(moduleName)).append("\n");
sb.append(" moduleVersion: ").append(toIndentedString(moduleVersion)).append("\n");
sb.append(" methodName: ").append(toIndentedString(methodName)).append("\n");
sb.append(" fileName: ").append(toIndentedString(fileName)).append("\n");
sb.append(" lineNumber: ").append(toIndentedString(lineNumber)).append("\n");
sb.append(" className: ").append(toIndentedString(className)).append("\n");
sb.append(" nativeMethod: ").append(toIndentedString(nativeMethod)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy