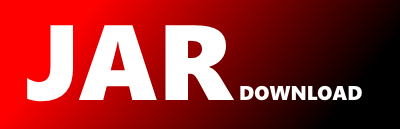
com.mailslurp.models.CreateDomainOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mailslurp-client-java Show documentation
Show all versions of mailslurp-client-java Show documentation
Official MailSlurp email API - create real inboxes then send and receive emails and attachments from tests and code.
The newest version!
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Options for creating a domain to use with MailSlurp. You must have ownership access to this domain in order to verify it. Domains will not function correctly until the domain has been verified. See https://www.mailslurp.com/guides/custom-domains for help. Domains can be either `HTTP` or `SMTP` type. The type of domain determines which inboxes can be used with it. `SMTP` inboxes use a mail server running `mxslurp.click` while `HTTP` inboxes are handled by AWS SES.
*/
@ApiModel(description = "Options for creating a domain to use with MailSlurp. You must have ownership access to this domain in order to verify it. Domains will not function correctly until the domain has been verified. See https://www.mailslurp.com/guides/custom-domains for help. Domains can be either `HTTP` or `SMTP` type. The type of domain determines which inboxes can be used with it. `SMTP` inboxes use a mail server running `mxslurp.click` while `HTTP` inboxes are handled by AWS SES.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-03T06:03:24.997Z[GMT]")
public class CreateDomainOptions {
public static final String SERIALIZED_NAME_DOMAIN = "domain";
@SerializedName(SERIALIZED_NAME_DOMAIN)
private String domain;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_CREATED_CATCH_ALL_INBOX = "createdCatchAllInbox";
@SerializedName(SERIALIZED_NAME_CREATED_CATCH_ALL_INBOX)
private Boolean createdCatchAllInbox;
/**
* Type of domain. Dictates type of inbox that can be created with domain. HTTP means inboxes are processed using SES while SMTP inboxes use a custom SMTP mail server. SMTP does not support sending so use HTTP for sending emails.
*/
@JsonAdapter(DomainTypeEnum.Adapter.class)
public enum DomainTypeEnum {
HTTP_INBOX("HTTP_INBOX"),
SMTP_DOMAIN("SMTP_DOMAIN");
private String value;
DomainTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DomainTypeEnum fromValue(String value) {
for (DomainTypeEnum b : DomainTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DomainTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DomainTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DomainTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DOMAIN_TYPE = "domainType";
@SerializedName(SERIALIZED_NAME_DOMAIN_TYPE)
private DomainTypeEnum domainType;
public CreateDomainOptions domain(String domain) {
this.domain = domain;
return this;
}
/**
* The top level domain you wish to use with MailSlurp. Do not specify subdomain just the top level. So `test.com` covers all subdomains such as `mail.test.com`. Don't include a protocol such as `http://`. Once added you must complete the verification steps by adding the returned records to your domain.
* @return domain
**/
@ApiModelProperty(required = true, value = "The top level domain you wish to use with MailSlurp. Do not specify subdomain just the top level. So `test.com` covers all subdomains such as `mail.test.com`. Don't include a protocol such as `http://`. Once added you must complete the verification steps by adding the returned records to your domain.")
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public CreateDomainOptions description(String description) {
this.description = description;
return this;
}
/**
* Optional description of the domain.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Optional description of the domain.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public CreateDomainOptions createdCatchAllInbox(Boolean createdCatchAllInbox) {
this.createdCatchAllInbox = createdCatchAllInbox;
return this;
}
/**
* Whether to create a catch all inbox for the domain. Any email sent to an address using your domain that cannot be matched to an existing inbox you created with the domain will be routed to the created catch all inbox. You can access emails using the regular methods on this inbox ID.
* @return createdCatchAllInbox
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether to create a catch all inbox for the domain. Any email sent to an address using your domain that cannot be matched to an existing inbox you created with the domain will be routed to the created catch all inbox. You can access emails using the regular methods on this inbox ID.")
public Boolean getCreatedCatchAllInbox() {
return createdCatchAllInbox;
}
public void setCreatedCatchAllInbox(Boolean createdCatchAllInbox) {
this.createdCatchAllInbox = createdCatchAllInbox;
}
public CreateDomainOptions domainType(DomainTypeEnum domainType) {
this.domainType = domainType;
return this;
}
/**
* Type of domain. Dictates type of inbox that can be created with domain. HTTP means inboxes are processed using SES while SMTP inboxes use a custom SMTP mail server. SMTP does not support sending so use HTTP for sending emails.
* @return domainType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of domain. Dictates type of inbox that can be created with domain. HTTP means inboxes are processed using SES while SMTP inboxes use a custom SMTP mail server. SMTP does not support sending so use HTTP for sending emails.")
public DomainTypeEnum getDomainType() {
return domainType;
}
public void setDomainType(DomainTypeEnum domainType) {
this.domainType = domainType;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateDomainOptions createDomainOptions = (CreateDomainOptions) o;
return Objects.equals(this.domain, createDomainOptions.domain) &&
Objects.equals(this.description, createDomainOptions.description) &&
Objects.equals(this.createdCatchAllInbox, createDomainOptions.createdCatchAllInbox) &&
Objects.equals(this.domainType, createDomainOptions.domainType);
}
@Override
public int hashCode() {
return Objects.hash(domain, description, createdCatchAllInbox, domainType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateDomainOptions {\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" createdCatchAllInbox: ").append(toIndentedString(createdCatchAllInbox)).append("\n");
sb.append(" domainType: ").append(toIndentedString(domainType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy