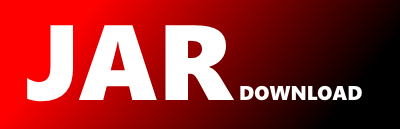
com.mailslurp.models.ImapSmtpAccessServers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mailslurp-client-java Show documentation
Show all versions of mailslurp-client-java Show documentation
Official MailSlurp email API - create real inboxes then send and receive emails and attachments from tests and code.
The newest version!
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.mailslurp.models.ServerEndpoints;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* IMAP and SMTP server endpoints for MailSlurp
*/
@ApiModel(description = "IMAP and SMTP server endpoints for MailSlurp")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-03T06:03:24.997Z[GMT]")
public class ImapSmtpAccessServers {
public static final String SERIALIZED_NAME_IMAP_SERVER = "imapServer";
@SerializedName(SERIALIZED_NAME_IMAP_SERVER)
private ServerEndpoints imapServer;
public static final String SERIALIZED_NAME_SECURE_IMAP_SERVER = "secureImapServer";
@SerializedName(SERIALIZED_NAME_SECURE_IMAP_SERVER)
private ServerEndpoints secureImapServer;
public static final String SERIALIZED_NAME_SMTP_SERVER = "smtpServer";
@SerializedName(SERIALIZED_NAME_SMTP_SERVER)
private ServerEndpoints smtpServer;
public static final String SERIALIZED_NAME_SECURE_SMTP_SERVER = "secureSmtpServer";
@SerializedName(SERIALIZED_NAME_SECURE_SMTP_SERVER)
private ServerEndpoints secureSmtpServer;
public ImapSmtpAccessServers imapServer(ServerEndpoints imapServer) {
this.imapServer = imapServer;
return this;
}
/**
* Get imapServer
* @return imapServer
**/
@ApiModelProperty(required = true, value = "")
public ServerEndpoints getImapServer() {
return imapServer;
}
public void setImapServer(ServerEndpoints imapServer) {
this.imapServer = imapServer;
}
public ImapSmtpAccessServers secureImapServer(ServerEndpoints secureImapServer) {
this.secureImapServer = secureImapServer;
return this;
}
/**
* Get secureImapServer
* @return secureImapServer
**/
@ApiModelProperty(required = true, value = "")
public ServerEndpoints getSecureImapServer() {
return secureImapServer;
}
public void setSecureImapServer(ServerEndpoints secureImapServer) {
this.secureImapServer = secureImapServer;
}
public ImapSmtpAccessServers smtpServer(ServerEndpoints smtpServer) {
this.smtpServer = smtpServer;
return this;
}
/**
* Get smtpServer
* @return smtpServer
**/
@ApiModelProperty(required = true, value = "")
public ServerEndpoints getSmtpServer() {
return smtpServer;
}
public void setSmtpServer(ServerEndpoints smtpServer) {
this.smtpServer = smtpServer;
}
public ImapSmtpAccessServers secureSmtpServer(ServerEndpoints secureSmtpServer) {
this.secureSmtpServer = secureSmtpServer;
return this;
}
/**
* Get secureSmtpServer
* @return secureSmtpServer
**/
@ApiModelProperty(required = true, value = "")
public ServerEndpoints getSecureSmtpServer() {
return secureSmtpServer;
}
public void setSecureSmtpServer(ServerEndpoints secureSmtpServer) {
this.secureSmtpServer = secureSmtpServer;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ImapSmtpAccessServers imapSmtpAccessServers = (ImapSmtpAccessServers) o;
return Objects.equals(this.imapServer, imapSmtpAccessServers.imapServer) &&
Objects.equals(this.secureImapServer, imapSmtpAccessServers.secureImapServer) &&
Objects.equals(this.smtpServer, imapSmtpAccessServers.smtpServer) &&
Objects.equals(this.secureSmtpServer, imapSmtpAccessServers.secureSmtpServer);
}
@Override
public int hashCode() {
return Objects.hash(imapServer, secureImapServer, smtpServer, secureSmtpServer);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ImapSmtpAccessServers {\n");
sb.append(" imapServer: ").append(toIndentedString(imapServer)).append("\n");
sb.append(" secureImapServer: ").append(toIndentedString(secureImapServer)).append("\n");
sb.append(" smtpServer: ").append(toIndentedString(smtpServer)).append("\n");
sb.append(" secureSmtpServer: ").append(toIndentedString(secureSmtpServer)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy