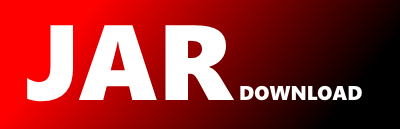
com.mailslurp.models.WebhookBouncePayload Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mailslurp-client-java Show documentation
Show all versions of mailslurp-client-java Show documentation
Official MailSlurp email API - create real inboxes then send and receive emails and attachments from tests and code.
The newest version!
/*
* MailSlurp API
* MailSlurp is an API for sending and receiving emails from dynamically allocated email addresses. It's designed for developers and QA teams to test applications, process inbound emails, send templated notifications, attachments, and more. ## Resources - [Homepage](https://www.mailslurp.com) - Get an [API KEY](https://app.mailslurp.com/sign-up/) - Generated [SDK Clients](https://docs.mailslurp.com/) - [Examples](https://github.com/mailslurp/examples) repository
*
* The version of the OpenAPI document: 6.5.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mailslurp.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
/**
* BOUNCE webhook payload. Sent to your webhook url endpoint via HTTP POST when an email bounced or was rejected by a recipient. Save the recipients to a ban list on your server and avoid emailing them again. It is recommended you also listen to the BOUNCE_RECIPIENT payload.
*/
@ApiModel(description = "BOUNCE webhook payload. Sent to your webhook url endpoint via HTTP POST when an email bounced or was rejected by a recipient. Save the recipients to a ban list on your server and avoid emailing them again. It is recommended you also listen to the BOUNCE_RECIPIENT payload.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-03T06:03:24.997Z[GMT]")
public class WebhookBouncePayload {
public static final String SERIALIZED_NAME_MESSAGE_ID = "messageId";
@SerializedName(SERIALIZED_NAME_MESSAGE_ID)
private String messageId;
public static final String SERIALIZED_NAME_WEBHOOK_ID = "webhookId";
@SerializedName(SERIALIZED_NAME_WEBHOOK_ID)
private UUID webhookId;
/**
* Name of the event type webhook is being triggered for.
*/
@JsonAdapter(EventNameEnum.Adapter.class)
public enum EventNameEnum {
EMAIL_RECEIVED("EMAIL_RECEIVED"),
NEW_EMAIL("NEW_EMAIL"),
NEW_CONTACT("NEW_CONTACT"),
NEW_ATTACHMENT("NEW_ATTACHMENT"),
EMAIL_OPENED("EMAIL_OPENED"),
EMAIL_READ("EMAIL_READ"),
DELIVERY_STATUS("DELIVERY_STATUS"),
BOUNCE("BOUNCE"),
BOUNCE_RECIPIENT("BOUNCE_RECIPIENT"),
NEW_SMS("NEW_SMS");
private String value;
EventNameEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static EventNameEnum fromValue(String value) {
for (EventNameEnum b : EventNameEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final EventNameEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public EventNameEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return EventNameEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_EVENT_NAME = "eventName";
@SerializedName(SERIALIZED_NAME_EVENT_NAME)
private EventNameEnum eventName;
public static final String SERIALIZED_NAME_WEBHOOK_NAME = "webhookName";
@SerializedName(SERIALIZED_NAME_WEBHOOK_NAME)
private String webhookName;
public static final String SERIALIZED_NAME_BOUNCE_ID = "bounceId";
@SerializedName(SERIALIZED_NAME_BOUNCE_ID)
private UUID bounceId;
public static final String SERIALIZED_NAME_SENT_TO_RECIPIENTS = "sentToRecipients";
@SerializedName(SERIALIZED_NAME_SENT_TO_RECIPIENTS)
private List sentToRecipients = null;
public static final String SERIALIZED_NAME_SENDER = "sender";
@SerializedName(SERIALIZED_NAME_SENDER)
private String sender;
public static final String SERIALIZED_NAME_BOUNCE_RECIPIENTS = "bounceRecipients";
@SerializedName(SERIALIZED_NAME_BOUNCE_RECIPIENTS)
private List bounceRecipients = null;
public WebhookBouncePayload messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Idempotent message ID. Store this ID locally or in a database to prevent message duplication.
* @return messageId
**/
@ApiModelProperty(required = true, value = "Idempotent message ID. Store this ID locally or in a database to prevent message duplication.")
public String getMessageId() {
return messageId;
}
public void setMessageId(String messageId) {
this.messageId = messageId;
}
public WebhookBouncePayload webhookId(UUID webhookId) {
this.webhookId = webhookId;
return this;
}
/**
* ID of webhook entity being triggered
* @return webhookId
**/
@ApiModelProperty(required = true, value = "ID of webhook entity being triggered")
public UUID getWebhookId() {
return webhookId;
}
public void setWebhookId(UUID webhookId) {
this.webhookId = webhookId;
}
public WebhookBouncePayload eventName(EventNameEnum eventName) {
this.eventName = eventName;
return this;
}
/**
* Name of the event type webhook is being triggered for.
* @return eventName
**/
@ApiModelProperty(required = true, value = "Name of the event type webhook is being triggered for.")
public EventNameEnum getEventName() {
return eventName;
}
public void setEventName(EventNameEnum eventName) {
this.eventName = eventName;
}
public WebhookBouncePayload webhookName(String webhookName) {
this.webhookName = webhookName;
return this;
}
/**
* Name of the webhook being triggered
* @return webhookName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the webhook being triggered")
public String getWebhookName() {
return webhookName;
}
public void setWebhookName(String webhookName) {
this.webhookName = webhookName;
}
public WebhookBouncePayload bounceId(UUID bounceId) {
this.bounceId = bounceId;
return this;
}
/**
* ID of the bounce email record. Use the ID with the bounce controller to view more information
* @return bounceId
**/
@ApiModelProperty(required = true, value = "ID of the bounce email record. Use the ID with the bounce controller to view more information")
public UUID getBounceId() {
return bounceId;
}
public void setBounceId(UUID bounceId) {
this.bounceId = bounceId;
}
public WebhookBouncePayload sentToRecipients(List sentToRecipients) {
this.sentToRecipients = sentToRecipients;
return this;
}
public WebhookBouncePayload addSentToRecipientsItem(String sentToRecipientsItem) {
if (this.sentToRecipients == null) {
this.sentToRecipients = new ArrayList<>();
}
this.sentToRecipients.add(sentToRecipientsItem);
return this;
}
/**
* Email sent to recipients
* @return sentToRecipients
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Email sent to recipients")
public List getSentToRecipients() {
return sentToRecipients;
}
public void setSentToRecipients(List sentToRecipients) {
this.sentToRecipients = sentToRecipients;
}
public WebhookBouncePayload sender(String sender) {
this.sender = sender;
return this;
}
/**
* Sender causing bounce
* @return sender
**/
@ApiModelProperty(required = true, value = "Sender causing bounce")
public String getSender() {
return sender;
}
public void setSender(String sender) {
this.sender = sender;
}
public WebhookBouncePayload bounceRecipients(List bounceRecipients) {
this.bounceRecipients = bounceRecipients;
return this;
}
public WebhookBouncePayload addBounceRecipientsItem(String bounceRecipientsItem) {
if (this.bounceRecipients == null) {
this.bounceRecipients = new ArrayList<>();
}
this.bounceRecipients.add(bounceRecipientsItem);
return this;
}
/**
* Email addresses that resulted in a bounce or email being rejected. Please save these recipients and avoid emailing them in the future to maintain your reputation.
* @return bounceRecipients
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Email addresses that resulted in a bounce or email being rejected. Please save these recipients and avoid emailing them in the future to maintain your reputation.")
public List getBounceRecipients() {
return bounceRecipients;
}
public void setBounceRecipients(List bounceRecipients) {
this.bounceRecipients = bounceRecipients;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WebhookBouncePayload webhookBouncePayload = (WebhookBouncePayload) o;
return Objects.equals(this.messageId, webhookBouncePayload.messageId) &&
Objects.equals(this.webhookId, webhookBouncePayload.webhookId) &&
Objects.equals(this.eventName, webhookBouncePayload.eventName) &&
Objects.equals(this.webhookName, webhookBouncePayload.webhookName) &&
Objects.equals(this.bounceId, webhookBouncePayload.bounceId) &&
Objects.equals(this.sentToRecipients, webhookBouncePayload.sentToRecipients) &&
Objects.equals(this.sender, webhookBouncePayload.sender) &&
Objects.equals(this.bounceRecipients, webhookBouncePayload.bounceRecipients);
}
@Override
public int hashCode() {
return Objects.hash(messageId, webhookId, eventName, webhookName, bounceId, sentToRecipients, sender, bounceRecipients);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WebhookBouncePayload {\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" webhookId: ").append(toIndentedString(webhookId)).append("\n");
sb.append(" eventName: ").append(toIndentedString(eventName)).append("\n");
sb.append(" webhookName: ").append(toIndentedString(webhookName)).append("\n");
sb.append(" bounceId: ").append(toIndentedString(bounceId)).append("\n");
sb.append(" sentToRecipients: ").append(toIndentedString(sentToRecipients)).append("\n");
sb.append(" sender: ").append(toIndentedString(sender)).append("\n");
sb.append(" bounceRecipients: ").append(toIndentedString(bounceRecipients)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy