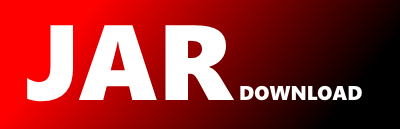
com.mangofactory.swagger.authorization.AuthorizationContext Maven / Gradle / Ivy
package com.mangofactory.swagger.authorization;
import com.mangofactory.swagger.scanners.RegexRequestMappingPatternMatcher;
import com.mangofactory.swagger.scanners.RequestMappingPatternMatcher;
import com.mangofactory.swagger.models.dto.Authorization;
import org.springframework.util.CollectionUtils;
import org.springframework.web.bind.annotation.RequestMethod;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* A class to represent a default set of authorizations to apply to each api operation
* To customize which request mappings the list of authorizations are applied to Specify the custom includePatterns
* or requestMethods
*/
public class AuthorizationContext {
private final List authorizations;
private final RequestMappingPatternMatcher requestMappingPatternMatcher;
private final List includePatterns;
private final RequestMethod[] requestMethods;
private AuthorizationContext(AuthorizationContextBuilder builder) {
this.authorizations = builder.authorizations;
this.includePatterns = builder.includePatterns;
this.requestMappingPatternMatcher = builder.requestMappingPatternMatcher;
this.requestMethods = builder.requestMethods;
}
public List getAuthorizationsForPath(String path) {
if (requestMappingPatternMatcher.pathMatchesOneOfIncluded(path, includePatterns)) {
return authorizations;
}
return null;
}
public List getAuthorizations() {
return authorizations;
}
public List getScalaAuthorizations() {
return CollectionUtils.isEmpty(authorizations) ? new ArrayList() : this.authorizations;
}
public static class AuthorizationContextBuilder {
private List authorizations;
private RequestMappingPatternMatcher requestMappingPatternMatcher = new RegexRequestMappingPatternMatcher();
private List includePatterns = Arrays.asList(new String[]{".*?"});
private RequestMethod[] requestMethods = RequestMethod.values();
public AuthorizationContextBuilder(List authorizations) {
this.authorizations = authorizations;
}
public AuthorizationContextBuilder withRequestMappingPatternMatcher(RequestMappingPatternMatcher matcher) {
this.requestMappingPatternMatcher = matcher;
return this;
}
public AuthorizationContextBuilder withIncludePatterns(List includePatterns) {
this.includePatterns = includePatterns;
return this;
}
public AuthorizationContextBuilder withRequestMethods(RequestMethod[] requestMethods) {
this.requestMethods = requestMethods;
return this;
}
public AuthorizationContext build() {
return new AuthorizationContext(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy