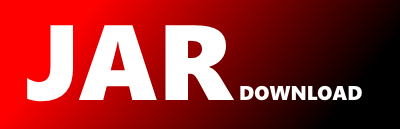
com.mangopay.entities.PayIn Maven / Gradle / Ivy
package com.mangopay.entities;
import com.google.gson.annotations.SerializedName;
import com.mangopay.core.enumerations.PayInExecutionType;
import com.mangopay.core.enumerations.PayInPaymentType;
import com.mangopay.core.interfaces.PayInExecutionDetails;
import com.mangopay.core.interfaces.PayInPaymentDetails;
import com.mangopay.entities.subentities.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/**
* PayIn entity.
*/
public class PayIn extends Transaction {
/**
* Credited wallet identifier.
*/
@SerializedName("CreditedWalletId")
private String creditedWalletId;
/**
* Type of payment.
*/
@SerializedName("PaymentType")
private PayInPaymentType paymentType;
/**
* One of PayInPaymentDetails implementations, depending on PaymentType.
*/
@SerializedName("PaymentDetails")
private PayInPaymentDetails paymentDetails;
/**
* Type of execution.
*/
@SerializedName("ExecutionType")
private PayInExecutionType executionType;
/**
* One of PayInExecutionDetails implementations, depending on ExecutionType.
*/
@SerializedName("ExecutionDetails")
private PayInExecutionDetails executionDetails;
public String getCreditedWalletId() {
return creditedWalletId;
}
public void setCreditedWalletId(String creditedWalletId) {
this.creditedWalletId = creditedWalletId;
}
public PayInPaymentType getPaymentType() {
return paymentType;
}
public void setPaymentType(PayInPaymentType paymentType) {
this.paymentType = paymentType;
}
public PayInPaymentDetails getPaymentDetails() {
return paymentDetails;
}
public void setPaymentDetails(PayInPaymentDetails paymentDetails) {
this.paymentDetails = paymentDetails;
}
public PayInExecutionType getExecutionType() {
return executionType;
}
public void setExecutionType(PayInExecutionType executionType) {
this.executionType = executionType;
}
public PayInExecutionDetails getExecutionDetails() {
return executionDetails;
}
public void setExecutionDetails(PayInExecutionDetails executionDetails) {
this.executionDetails = executionDetails;
}
/**
* Gets the structure that maps which property depends on other property.
*
* @return
*/
@Override
public Map>>> getDependentObjects() {
return new HashMap>>>() {{
put("PaymentType", new HashMap>>() {{
put("CARD", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsCard.class);
}}
);
put("PREAUTHORIZED", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsPreAuthorized.class);
}}
);
put("BANK_WIRE", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsBankWire.class);
}}
);
put("DIRECT_DEBIT", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsDirectDebit.class);
}}
);
put("PAYPAL", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsPayPal.class);
}}
);
put("GOOGLEPAY", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsGooglePay.class);
}}
);
put("GOOGLE_PAY", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsGooglePayV2.class);
}}
);
put("PAYCONIQ", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsPayconiq.class);
}}
);
put("MBWAY", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsMbway.class);
}}
);
put("SATISPAY", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsSatispay.class);
}}
);
put("BLIK", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsBlik.class);
}}
);
put("MULTIBANCO", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsMultibanco.class);
}}
);
put("KLARNA", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsKlarna.class);
}}
);
put("IDEAL", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsIdeal.class);
}}
);
put("GIROPAY", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsGiropay.class);
}}
);
put("BCMC", new HashMap>() {{
put("PaymentDetails", PayInPaymentDetailsBancontact.class);
}}
);
// ...and more in future...
}}
);
put("ExecutionType", new HashMap>>() {{
put("WEB", new HashMap>() {{
put("ExecutionDetails", PayInExecutionDetailsWeb.class);
}}
);
put("DIRECT", new HashMap>() {{
put("ExecutionDetails", PayInExecutionDetailsDirect.class);
}}
);
put("EXTERNAL_INSTRUCTION", new HashMap>() {{
put("ExecutionDetails", PayInExecutionDetailsBankingAlias.class);
}}
);
// ...and more in future...
}}
);
}};
}
/**
* Gets the collection of read-only fields names.
*
* @return List of field names.
*/
@Override
public ArrayList getReadOnlyProperties() {
ArrayList result = super.getReadOnlyProperties();
result.add("PaymentType");
result.add("ExecutionType");
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy