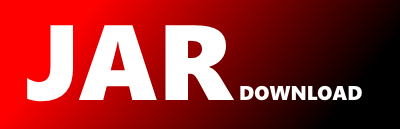
com.manoelcampos.gossipsimulator.GossipNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gossip-simulator Show documentation
Show all versions of gossip-simulator Show documentation
An API for creation of applications to simulate the Gossip Protocol
package com.manoelcampos.gossipsimulator;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collection;
import java.util.Set;
/**
* A node that shares {@link #getMessage() data}
* across its {@link #getNeighbours() neighbourhood} using the Gossip Protocol.
*
* @param the type of the data the node shares
*/
public interface GossipNode extends Comparable>{
Logger LOGGER = LoggerFactory.getLogger(GossipNode.class.getSimpleName());
long getId();
/**
* Gets the latest message the node is storing.
* This data may have been generated for this node or received
* by other nodes, in order to spread such a data through the neighbourhood.
* @return the latest message stored or null if the node was never infected (if a message or
* never received from another node or manually set.
* @see #sendMessage()
*/
T getMessage();
/**
* Stores a message to be sent to nodes in the neighbourhood.
* @param message
* @see #sendMessage()
*/
void setMessage(T message);
/**
* Sends a {@link #getMessage() stored message} to {@link GossipConfig#getFanout() N (fanout)} randomly selected nodes
* in the {@link #getNeighbours() neighbourhood}.
* @return true if the node has some message to send and it was sent,
* false otherwise
* @see #getMessage()
*/
boolean sendMessage();
/**
* Receives a message from a source node and updates the list of know neighbours.
* @param source the node sending the message
* @param data the data sent
*/
void receiveMessage(GossipNode source, T data);
/**
* Adds a node as a neighbour
* @param neighbour the node to add
* @return true if the node was added, false it the given node is this one
*/
boolean addNeighbour(GossipNode neighbour);
/**
* Adds a collection of nodes as neighbours
* @param newNeighbours the nodes to add
* @return true if the nodes were added, false if the given nodes area already in the neighbourhood
*/
boolean addNeighbours(Collection> newNeighbours);
/**
* Gets an unmodifiable Set of neighbours.
* @return
*/
Set> getNeighbours();
int getNeighbourhoodSize();
/**
* Indicates if the node has received any data already.
* @return
*/
boolean isInfected();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy