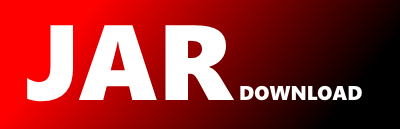
com.manywho.sdk.services.database.DatabaseRepository Maven / Gradle / Ivy
package com.manywho.sdk.services.database;
import com.manywho.sdk.services.types.Type;
import com.manywho.sdk.services.types.TypeParser;
import org.reflections.Reflections;
import javax.inject.Inject;
import java.util.Set;
public class DatabaseRepository {
private final Reflections reflections;
private Set> databases;
private Set> readOnlyDatabases;
private Set> writableDatabases;
@Inject
public DatabaseRepository(Reflections reflections) {
this.reflections = reflections;
}
public Class extends RawDatabase>> findRawDatabase() {
return (Class extends RawDatabase>>) reflections.getSubTypesOf(RawDatabase.class).stream()
.findFirst()
.orElseThrow(() -> new RuntimeException("Unable to find an implementation of " + RawDatabase.class.getCanonicalName()));
}
public Class extends Database, T>> findDatabase(Class type) {
return (Class extends Database, T>>) getDatabases().stream()
.filter(database -> TypeParser.findGenericType(database.getGenericInterfaces()[0], 1).equals(type))
.findFirst()
.orElseThrow(() -> new RuntimeException("Unable to find an implementation of Database for the type " + type.getCanonicalName()));
}
public Class extends ReadOnlyDatabase, T>> findReadOnlyDatabase(Class type) {
return (Class extends ReadOnlyDatabase, T>>) getReadOnlyDatabases().stream()
.filter(database -> !database.isAssignableFrom(Database.class))
.filter(database -> TypeParser.findGenericType(database.getGenericInterfaces()[0], 1).equals(type))
.findFirst()
.orElseThrow(() -> new RuntimeException("Unable to find an implementation of ReadOnlyDatabase for the type " + type.getCanonicalName()));
}
public Class extends WritableDatabase, T>> findWritableDatabase(Class type) {
return (Class extends WritableDatabase, T>>) getWritableDatabases().stream()
.filter(database -> !database.isAssignableFrom(Database.class))
.filter(database -> TypeParser.findGenericType(database.getGenericInterfaces()[0], 1).equals(type))
.findFirst()
.orElseThrow(() -> new RuntimeException("Unable to find an implementation of WritableDatabase for the type " + type.getCanonicalName()));
}
public Set> getDatabases() {
if (databases == null) {
databases = reflections.getSubTypesOf(Database.class);
}
return databases;
}
public Set> getReadOnlyDatabases() {
if (readOnlyDatabases == null) {
readOnlyDatabases = reflections.getSubTypesOf(ReadOnlyDatabase.class);
}
return readOnlyDatabases;
}
public Set> getWritableDatabases() {
if (writableDatabases == null) {
writableDatabases = reflections.getSubTypesOf(WritableDatabase.class);
}
return writableDatabases;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy