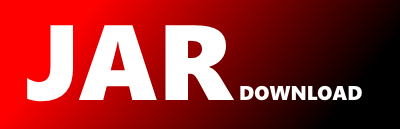
com.mapbox.mapboxsdk.util.DataLoadingUtils Maven / Gradle / Ivy
package com.mapbox.mapboxsdk.util;
import android.content.Context;
import android.graphics.Paint;
import android.text.TextUtils;
import android.util.Log;
import com.cocoahero.android.geojson.Feature;
import com.cocoahero.android.geojson.FeatureCollection;
import com.cocoahero.android.geojson.GeoJSON;
import com.cocoahero.android.geojson.LineString;
import com.cocoahero.android.geojson.MultiLineString;
import com.cocoahero.android.geojson.MultiPoint;
import com.cocoahero.android.geojson.MultiPolygon;
import com.cocoahero.android.geojson.Point;
import com.cocoahero.android.geojson.Polygon;
import com.mapbox.mapboxsdk.geometry.LatLng;
import com.mapbox.mapboxsdk.overlay.Icon;
import com.mapbox.mapboxsdk.overlay.Marker;
import com.mapbox.mapboxsdk.overlay.PathOverlay;
import com.mapbox.mapboxsdk.util.constants.UtilConstants;
import org.json.JSONArray;
import org.json.JSONException;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Locale;
public class DataLoadingUtils {
/**
* Load GeoJSON from URL (in synchronous manner) and return GeoJSON FeatureCollection
* @param url URL of GeoJSON data
* @return Remote GeoJSON parsed into Library objects
* @throws IOException
* @throws JSONException
*/
public static FeatureCollection loadGeoJSONFromUrl(final String url) throws IOException, JSONException {
if (TextUtils.isEmpty(url)) {
throw new NullPointerException("No GeoJSON URL passed in.");
}
if (UtilConstants.DEBUGMODE) {
Log.d(DataLoadingUtils.class.getCanonicalName(), "Mapbox SDK downloading GeoJSON URL: " + url);
}
InputStream is;
if (url.toLowerCase(Locale.US).indexOf("http") == 0) {
is = NetworkUtils.getHttpURLConnection(new URL(url)).getInputStream();
} else {
is = new URL(url).openStream();
}
BufferedReader rd = new BufferedReader(new InputStreamReader(is, Charset.forName("UTF-8")));
String jsonText = readAll(rd);
FeatureCollection parsed = (FeatureCollection) GeoJSON.parse(jsonText);
if (UtilConstants.DEBUGMODE) {
Log.d(DataLoadingUtils.class.getCanonicalName(), "Parsed GeoJSON with " + parsed.getFeatures().size() + " features.");
}
return parsed;
}
/**
* Load GeoJSON from URL (in synchronous manner) and return GeoJSON FeatureCollection
* @param context Application's Context
* @param fileName Name of file in assets directory
* @return Local GeoJSON file parsed into Library objects
* @throws IOException
* @throws JSONException
*/
public static FeatureCollection loadGeoJSONFromAssets(final Context context, final String fileName) throws IOException, JSONException {
if (TextUtils.isEmpty(fileName)) {
throw new NullPointerException("No GeoJSON File Name passed in.");
}
if (UtilConstants.DEBUGMODE) {
Log.d(DataLoadingUtils.class.getCanonicalName(), "Mapbox SDK loading GeoJSON URL: " + fileName);
}
InputStream is = context.getAssets().open(fileName);
BufferedReader rd = new BufferedReader(new InputStreamReader(is, Charset.forName("UTF-8")));
String jsonText = readAll(rd);
FeatureCollection parsed = (FeatureCollection) GeoJSON.parse(jsonText);
if (UtilConstants.DEBUGMODE) {
Log.d(DataLoadingUtils.class.getCanonicalName(), "Parsed GeoJSON with " + parsed.getFeatures().size() + " features.");
}
return parsed;
}
public static String readAll(Reader rd) throws IOException {
StringBuilder sb = new StringBuilder();
int cp;
while ((cp = rd.read()) != -1) {
sb.append((char) cp);
}
return sb.toString();
}
/**
* Converts GeoJSON objects into Mapbox SDK UI Objects
* @param featureCollection Parsed GeoJSON Objects
* @param markerIcon Optional Icon to use for markers
* @return Collection of Mapbox SDK UI Objects
* @throws JSONException
*/
public static ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy