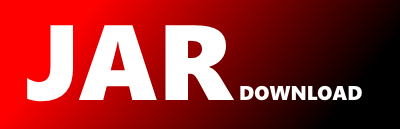
com.mapbox.mapboxsdk.annotations.Annotation Maven / Gradle / Ivy
package com.mapbox.mapboxsdk.annotations;
import android.support.annotation.NonNull;
import com.mapbox.mapboxsdk.maps.MapView;
import com.mapbox.mapboxsdk.maps.MapboxMap;
/**
* Annotation is an overlay on top of a {@link MapView},
* from which {@link Polygon}, {@link Polyline} and {@link Marker} are derived.
*
* it manages attachment to a map and identification, but does not require
* content to be placed at a geographical point.
*
*/
public abstract class Annotation implements Comparable {
/**
*
* The annotation id
*
* Internal C++ id is stored as unsigned int.
*/
private long id = -1; // -1 unless added to a MapView
private MapboxMap mapboxMap;
protected Annotation() {
}
/**
*
* Gets the annotation's unique ID.
*
* This ID is unique for a MapView instance and is suitable for associating your own extra
* data with.
*/
public long getId() {
return id;
}
public void remove() {
if (mapboxMap == null) {
return;
}
mapboxMap.removeAnnotation(this);
}
/**
* Do not use this method. Used internally by the SDK.
*/
public void setId(long id) {
this.id = id;
}
/**
* Do not use this method. Used internally by the SDK.
*/
public void setMapboxMap(MapboxMap mapboxMap) {
this.mapboxMap = mapboxMap;
}
/**
* Gets the associated MapboxMap
*
* @return The MapboxMap
*/
protected MapboxMap getMapboxMap() {
return mapboxMap;
}
@Override
public int compareTo(@NonNull Annotation annotation) {
if (id < annotation.getId()) {
return 1;
} else if (id > annotation.getId()) {
return -1;
}
// Equal
return 0;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Annotation that = (Annotation) o;
return getId() == that.getId();
}
@Override
public int hashCode() {
return (int) (getId() ^ (getId() >>> 32));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy