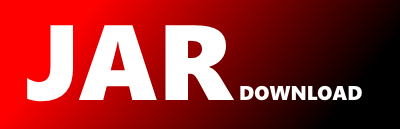
com.mapbox.mapboxsdk.style.layers.SymbolLayer Maven / Gradle / Ivy
// This file is generated. Edit android/platform/scripts/generate-style-code.js, then run `make style-code-android`.
package com.mapbox.mapboxsdk.style.layers;
import com.mapbox.mapboxsdk.exceptions.ConversionException;
import android.support.annotation.ColorInt;
import android.support.annotation.NonNull;
import static com.mapbox.mapboxsdk.utils.ColorUtils.*;
/**
* An icon or a text label.
*
* @see The online documentation
*/
public class SymbolLayer extends Layer {
/**
* Creates a SymbolLayer.
*
* @param nativePtr pointer used by core
*/
public SymbolLayer(long nativePtr) {
super(nativePtr);
}
/**
* Creates a SymbolLayer.
*
* @param layerId the id of the layer
* @param sourceId the id of the source
*/
public SymbolLayer(String layerId, String sourceId) {
initialize(layerId, sourceId);
}
protected native void initialize(String layerId, String sourceId);
/**
* Set the source layer.
*
* @param sourceLayer the source layer to set
*/
public void setSourceLayer(String sourceLayer) {
nativeSetSourceLayer(sourceLayer);
}
/**
* Set the source Layer.
*
* @param sourceLayer the source layer to set
* @return This
*/
public SymbolLayer withSourceLayer(String sourceLayer) {
setSourceLayer(sourceLayer);
return this;
}
/**
* Set a single filter.
*
* @param filter the filter to set
*/
public void setFilter(Filter.Statement filter) {
this.setFilter(filter.toArray());
}
/**
* Set an array of filters.
*
* @param filter the filter array to set
*/
public void setFilter(Object[] filter) {
nativeSetFilter(filter);
}
/**
* Set an array of filters.
*
* @param filter tthe filter array to set
* @return This
*/
public SymbolLayer withFilter(Object[] filter) {
setFilter(filter);
return this;
}
/**
* Set a single filter.
*
* @param filter the filter to set
* @return This
*/
public SymbolLayer withFilter(Filter.Statement filter) {
setFilter(filter);
return this;
}
/**
* Set a property or properties.
*
* @param properties the var-args properties
* @return This
*/
public SymbolLayer withProperties(@NonNull Property>... properties) {
setProperties(properties);
return this;
}
// Property getters
/**
* Get the SymbolPlacement property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getSymbolPlacement() {
return (PropertyValue) new PropertyValue(nativeGetSymbolPlacement());
}
/**
* Get the SymbolSpacing property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getSymbolSpacing() {
return (PropertyValue) new PropertyValue(nativeGetSymbolSpacing());
}
/**
* Get the SymbolAvoidEdges property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getSymbolAvoidEdges() {
return (PropertyValue) new PropertyValue(nativeGetSymbolAvoidEdges());
}
/**
* Get the IconAllowOverlap property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconAllowOverlap() {
return (PropertyValue) new PropertyValue(nativeGetIconAllowOverlap());
}
/**
* Get the IconIgnorePlacement property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconIgnorePlacement() {
return (PropertyValue) new PropertyValue(nativeGetIconIgnorePlacement());
}
/**
* Get the IconOptional property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconOptional() {
return (PropertyValue) new PropertyValue(nativeGetIconOptional());
}
/**
* Get the IconRotationAlignment property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconRotationAlignment() {
return (PropertyValue) new PropertyValue(nativeGetIconRotationAlignment());
}
/**
* Get the IconSize property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconSize() {
return (PropertyValue) new PropertyValue(nativeGetIconSize());
}
/**
* Get the IconTextFit property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTextFit() {
return (PropertyValue) new PropertyValue(nativeGetIconTextFit());
}
/**
* Get the IconTextFitPadding property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTextFitPadding() {
return (PropertyValue) new PropertyValue(nativeGetIconTextFitPadding());
}
/**
* Get the IconImage property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconImage() {
return (PropertyValue) new PropertyValue(nativeGetIconImage());
}
/**
* Get the IconRotate property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconRotate() {
return (PropertyValue) new PropertyValue(nativeGetIconRotate());
}
/**
* Get the IconPadding property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconPadding() {
return (PropertyValue) new PropertyValue(nativeGetIconPadding());
}
/**
* Get the IconKeepUpright property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconKeepUpright() {
return (PropertyValue) new PropertyValue(nativeGetIconKeepUpright());
}
/**
* Get the IconOffset property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconOffset() {
return (PropertyValue) new PropertyValue(nativeGetIconOffset());
}
/**
* Get the TextPitchAlignment property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextPitchAlignment() {
return (PropertyValue) new PropertyValue(nativeGetTextPitchAlignment());
}
/**
* Get the TextRotationAlignment property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextRotationAlignment() {
return (PropertyValue) new PropertyValue(nativeGetTextRotationAlignment());
}
/**
* Get the TextField property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextField() {
return (PropertyValue) new PropertyValue(nativeGetTextField());
}
/**
* Get the TextFont property
*
* @return property wrapper value around String[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextFont() {
return (PropertyValue) new PropertyValue(nativeGetTextFont());
}
/**
* Get the TextSize property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextSize() {
return (PropertyValue) new PropertyValue(nativeGetTextSize());
}
/**
* Get the TextMaxWidth property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextMaxWidth() {
return (PropertyValue) new PropertyValue(nativeGetTextMaxWidth());
}
/**
* Get the TextLineHeight property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextLineHeight() {
return (PropertyValue) new PropertyValue(nativeGetTextLineHeight());
}
/**
* Get the TextLetterSpacing property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextLetterSpacing() {
return (PropertyValue) new PropertyValue(nativeGetTextLetterSpacing());
}
/**
* Get the TextJustify property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextJustify() {
return (PropertyValue) new PropertyValue(nativeGetTextJustify());
}
/**
* Get the TextAnchor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextAnchor() {
return (PropertyValue) new PropertyValue(nativeGetTextAnchor());
}
/**
* Get the TextMaxAngle property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextMaxAngle() {
return (PropertyValue) new PropertyValue(nativeGetTextMaxAngle());
}
/**
* Get the TextRotate property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextRotate() {
return (PropertyValue) new PropertyValue(nativeGetTextRotate());
}
/**
* Get the TextPadding property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextPadding() {
return (PropertyValue) new PropertyValue(nativeGetTextPadding());
}
/**
* Get the TextKeepUpright property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextKeepUpright() {
return (PropertyValue) new PropertyValue(nativeGetTextKeepUpright());
}
/**
* Get the TextTransform property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextTransform() {
return (PropertyValue) new PropertyValue(nativeGetTextTransform());
}
/**
* Get the TextOffset property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextOffset() {
return (PropertyValue) new PropertyValue(nativeGetTextOffset());
}
/**
* Get the TextAllowOverlap property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextAllowOverlap() {
return (PropertyValue) new PropertyValue(nativeGetTextAllowOverlap());
}
/**
* Get the TextIgnorePlacement property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextIgnorePlacement() {
return (PropertyValue) new PropertyValue(nativeGetTextIgnorePlacement());
}
/**
* Get the TextOptional property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextOptional() {
return (PropertyValue) new PropertyValue(nativeGetTextOptional());
}
/**
* Get the IconOpacity property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconOpacity() {
return (PropertyValue) new PropertyValue(nativeGetIconOpacity());
}
/**
* Get the IconColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconColor() {
return (PropertyValue) new PropertyValue(nativeGetIconColor());
}
/**
* The color of the icon. This can only be used with sdf icons.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getIconColorAsInt() {
PropertyValue value = getIconColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("icon-color was set as a Function");
}
}
/**
* Get the IconHaloColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconHaloColor() {
return (PropertyValue) new PropertyValue(nativeGetIconHaloColor());
}
/**
* The color of the icon's halo. Icon halos can only be used with SDF icons.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getIconHaloColorAsInt() {
PropertyValue value = getIconHaloColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("icon-halo-color was set as a Function");
}
}
/**
* Get the IconHaloWidth property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconHaloWidth() {
return (PropertyValue) new PropertyValue(nativeGetIconHaloWidth());
}
/**
* Get the IconHaloBlur property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconHaloBlur() {
return (PropertyValue) new PropertyValue(nativeGetIconHaloBlur());
}
/**
* Get the IconTranslate property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTranslate() {
return (PropertyValue) new PropertyValue(nativeGetIconTranslate());
}
/**
* Get the IconTranslateAnchor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTranslateAnchor() {
return (PropertyValue) new PropertyValue(nativeGetIconTranslateAnchor());
}
/**
* Get the TextOpacity property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextOpacity() {
return (PropertyValue) new PropertyValue(nativeGetTextOpacity());
}
/**
* Get the TextColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextColor() {
return (PropertyValue) new PropertyValue(nativeGetTextColor());
}
/**
* The color with which the text will be drawn.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getTextColorAsInt() {
PropertyValue value = getTextColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("text-color was set as a Function");
}
}
/**
* Get the TextHaloColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextHaloColor() {
return (PropertyValue) new PropertyValue(nativeGetTextHaloColor());
}
/**
* The color of the text's halo, which helps it stand out from backgrounds.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getTextHaloColorAsInt() {
PropertyValue value = getTextHaloColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("text-halo-color was set as a Function");
}
}
/**
* Get the TextHaloWidth property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextHaloWidth() {
return (PropertyValue) new PropertyValue(nativeGetTextHaloWidth());
}
/**
* Get the TextHaloBlur property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextHaloBlur() {
return (PropertyValue) new PropertyValue(nativeGetTextHaloBlur());
}
/**
* Get the TextTranslate property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextTranslate() {
return (PropertyValue) new PropertyValue(nativeGetTextTranslate());
}
/**
* Get the TextTranslateAnchor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextTranslateAnchor() {
return (PropertyValue) new PropertyValue(nativeGetTextTranslateAnchor());
}
private native Object nativeGetSymbolPlacement();
private native Object nativeGetSymbolSpacing();
private native Object nativeGetSymbolAvoidEdges();
private native Object nativeGetIconAllowOverlap();
private native Object nativeGetIconIgnorePlacement();
private native Object nativeGetIconOptional();
private native Object nativeGetIconRotationAlignment();
private native Object nativeGetIconSize();
private native Object nativeGetIconTextFit();
private native Object nativeGetIconTextFitPadding();
private native Object nativeGetIconImage();
private native Object nativeGetIconRotate();
private native Object nativeGetIconPadding();
private native Object nativeGetIconKeepUpright();
private native Object nativeGetIconOffset();
private native Object nativeGetTextPitchAlignment();
private native Object nativeGetTextRotationAlignment();
private native Object nativeGetTextField();
private native Object nativeGetTextFont();
private native Object nativeGetTextSize();
private native Object nativeGetTextMaxWidth();
private native Object nativeGetTextLineHeight();
private native Object nativeGetTextLetterSpacing();
private native Object nativeGetTextJustify();
private native Object nativeGetTextAnchor();
private native Object nativeGetTextMaxAngle();
private native Object nativeGetTextRotate();
private native Object nativeGetTextPadding();
private native Object nativeGetTextKeepUpright();
private native Object nativeGetTextTransform();
private native Object nativeGetTextOffset();
private native Object nativeGetTextAllowOverlap();
private native Object nativeGetTextIgnorePlacement();
private native Object nativeGetTextOptional();
private native Object nativeGetIconOpacity();
private native Object nativeGetIconColor();
private native Object nativeGetIconHaloColor();
private native Object nativeGetIconHaloWidth();
private native Object nativeGetIconHaloBlur();
private native Object nativeGetIconTranslate();
private native Object nativeGetIconTranslateAnchor();
private native Object nativeGetTextOpacity();
private native Object nativeGetTextColor();
private native Object nativeGetTextHaloColor();
private native Object nativeGetTextHaloWidth();
private native Object nativeGetTextHaloBlur();
private native Object nativeGetTextTranslate();
private native Object nativeGetTextTranslateAnchor();
@Override
protected native void finalize() throws Throwable;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy