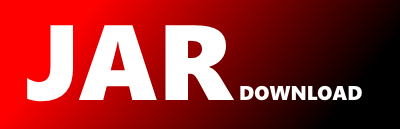
com.mapbox.mapboxsdk.style.functions.Function Maven / Gradle / Ivy
package com.mapbox.mapboxsdk.style.functions;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import com.mapbox.mapboxsdk.style.functions.stops.CategoricalStops;
import com.mapbox.mapboxsdk.style.functions.stops.ExponentialStops;
import com.mapbox.mapboxsdk.style.functions.stops.IdentityStops;
import com.mapbox.mapboxsdk.style.functions.stops.IntervalStops;
import com.mapbox.mapboxsdk.style.functions.stops.Stop;
import com.mapbox.mapboxsdk.style.functions.stops.Stops;
import java.util.Map;
import timber.log.Timber;
/**
* Functions are used to change properties in relation to the state of the map.
*
* The value for any layout or paint property may be specified as a function. Functions allow you to
* make the appearance of a map feature change with the current zoom level and/or the feature's properties.
*
* @param the function's input type
* @param the target property's value type. Make sure it matches.
* @see The style specification
*/
public class Function {
static final String PROPERTY_KEY = "property";
static final String DEFAULT_VALUE_KEY = "defaultValue";
/**
* Create an exponential {@link CameraFunction}
*
* Zoom functions allow the appearance of a map feature to change with map’s zoom.
* Zoom functions can be used to create the illusion of depth and control data density.
* Each stop is an array with two elements, the first is a zoom and the second is a function output value.
*
* @param the zoom level type (Float, Integer)
* @param the property type
* @param stops the stops implementation that define the function. @see {@link Stops#exponential(Stop[])}
* @return the {@link CameraFunction}
* @see CameraFunction
* @see ExponentialStops
* @see Stops#exponential(Stop[])
* @see Stops#exponential(Stop[])
* @see Stop#stop
*/
public static CameraFunction zoom(@NonNull ExponentialStops stops) {
return new CameraFunction<>(stops);
}
/**
* Create an interval {@link CameraFunction}
*
* Zoom functions allow the appearance of a map feature to change with map’s zoom.
* Zoom functions can be used to create the illusion of depth and control data density.
* Each stop is an array with two elements, the first is a zoom and the second is a function output value.
*
* @param the zoom level type (Float, Integer)
* @param the property type
* @param stops the stops implementation that define the function. @see {@link Stops#interval(Stop[])}
* @return the {@link CameraFunction}
* @see CameraFunction
* @see IntervalStops
* @see Stops#interval(Stop[])
* @see Stop#stop
*/
public static CameraFunction zoom(@NonNull IntervalStops stops) {
return new CameraFunction<>(stops);
}
/**
* Create an exponential {@link SourceFunction}
*
* Source functions allow the appearance of a map feature to change with
* its properties. Source functions can be used to visually differentiate
* types of features within the same layer or create data visualizations.
* Each stop is an array with two elements, the first is a property input
* value and the second is a function output value. Note that support for
* property functions is not available across all properties and platforms
* at this time.
*
* @param property the feature property name
* @param stops the stops
* @param the function input type
* @param the function output type
* @return the {@link SourceFunction}
* @see SourceFunction
* @see ExponentialStops
* @see Stops#exponential(Stop[])
* @see Stop#stop
*/
public static SourceFunction property(@NonNull String property, @NonNull ExponentialStops stops) {
return new SourceFunction<>(property, stops);
}
/**
* Create an identity {@link SourceFunction}
*
* Source functions allow the appearance of a map feature to change with
* its properties. Source functions can be used to visually differentiate
* types of features within the same layer or create data visualizations.
* Each stop is an array with two elements, the first is a property input
* value and the second is a function output value. Note that support for
* property functions is not available across all properties and platforms
* at this time.
*
* @param property the feature property name
* @param stops the stops
* @param the function input/output type
* @return the {@link SourceFunction}
* @see SourceFunction
* @see IdentityStops
* @see Stops#identity()
* @see Stop#stop
*/
public static SourceFunction property(@NonNull String property, @NonNull IdentityStops stops) {
return new SourceFunction<>(property, stops);
}
/**
* Create an interval {@link SourceFunction}
*
* Source functions allow the appearance of a map feature to change with
* its properties. Source functions can be used to visually differentiate
* types of features within the same layer or create data visualizations.
* Each stop is an array with two elements, the first is a property input
* value and the second is a function output value. Note that support for
* property functions is not available across all properties and platforms
* at this time.
*
* @param property the feature property name
* @param stops the stops
* @param the function input type
* @param the function output type
* @return the {@link SourceFunction}
* @see SourceFunction
* @see IntervalStops
* @see Stops#interval(Stop[])
* @see Stop#stop
*/
public static SourceFunction property(@NonNull String property, @NonNull IntervalStops stops) {
return new SourceFunction<>(property, stops);
}
/**
* Create an categorical {@link SourceFunction}
*
* Source functions allow the appearance of a map feature to change with
* its properties. Source functions can be used to visually differentiate
* types of features within the same layer or create data visualizations.
* Each stop is an array with two elements, the first is a property input
* value and the second is a function output value. Note that support for
* property functions is not available across all properties and platforms
* at this time.
*
* @param property the feature property name
* @param stops the stops
* @param the function input type
* @param the function output type
* @return the {@link SourceFunction}
* @see SourceFunction
* @see CategoricalStops
* @see Stops#categorical(Stop[])
* @see Stop#stop
*/
public static SourceFunction property(@NonNull String property, @NonNull CategoricalStops stops) {
return new SourceFunction<>(property, stops);
}
/**
* Create a composite, categorical function.
*
* Composite functions allow the appearance of a map feature to change with both its
* properties and zoom. Each stop is an array with two elements, the first is an object
* with a property input value and a zoom, and the second is a function output value. Note
* that support for property functions is not yet complete.
*
* @param property the feature property name for the source part of the function
* @param stops the stops
* @param the zoom function input type (Float usually)
* @param the function input type for the source part of the function
* @param the function output type
* @return the {@link CompositeFunction}
* @see CompositeFunction
* @see CategoricalStops
* @see Stops#categorical(Stop[])
* @see Stop#stop
*/
public static CompositeFunction composite(
@NonNull String property,
@NonNull CategoricalStops, O> stops) {
return new CompositeFunction<>(property, stops);
}
/**
* Create a composite, exponential function.
*
* Composite functions allow the appearance of a map feature to change with both its
* properties and zoom. Each stop is an array with two elements, the first is an object
* with a property input value and a zoom, and the second is a function output value. Note
* that support for property functions is not yet complete.
*
* @param property the feature property name for the source part of the function
* @param stops the stops
* @param the zoom function input type (Float usually)
* @param the function input type for the source part of the function
* @param the function output type
* @return the {@link CompositeFunction}
* @see CompositeFunction
* @see ExponentialStops
* @see Stops#exponential(Stop[])
* @see Stop#stop
*/
public static CompositeFunction composite(
@NonNull String property,
@NonNull ExponentialStops, O> stops) {
return new CompositeFunction<>(property, stops);
}
/**
* Create a composite, interval function.
*
* Composite functions allow the appearance of a map feature to change with both its
* properties and zoom. Each stop is an array with two elements, the first is an object
* with a property input value and a zoom, and the second is a function output value. Note
* that support for property functions is not yet complete.
*
* @param property the feature property name for the source part of the function
* @param stops the stops
* @param the zoom function input type (Float usually)
* @param the function input type for the source part of the function
* @param the function output type
* @return the {@link CompositeFunction}
* @see CompositeFunction
* @see IntervalStops
* @see Stops#interval(Stop[])
* @see Stop#stop
*/
public static CompositeFunction composite(
@NonNull String property,
@NonNull IntervalStops, O> stops) {
return new CompositeFunction<>(property, stops);
}
// Class definition //
private final Stops stops;
/**
* JNI Cosntructor for implementation classes
*
* @param stops the stops
*/
Function(@NonNull Stops stops) {
this.stops = stops;
}
/**
* @return the stops in this function
*/
public Stops getStops() {
return stops;
}
/**
* Convenience method
*
* @param the Stops implementation type
* @return the Stops implementation or null when the wrong type is specified
*/
@Nullable
public S getStopsAs() {
try {
// noinspection unchecked
return (S) stops;
} catch (ClassCastException exception) {
Timber.e(String.format("Stops: %s is a different type: %s", stops.getClass(), exception));
return null;
}
}
/**
* INTERNAL USAGE ONLY
*
* @return a value object representation for core conversion
*/
public Map toValueObject() {
return stops.toValueObject();
}
@Override
public String toString() {
return String.format("%s: %s", getClass().getSimpleName(), stops);
}
}