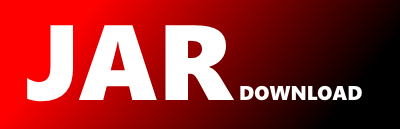
com.mapbox.mapboxsdk.style.functions.stops.Stops Maven / Gradle / Ivy
package com.mapbox.mapboxsdk.style.functions.stops;
import android.support.annotation.CallSuper;
import android.support.annotation.NonNull;
import android.support.annotation.Size;
import java.util.HashMap;
import java.util.Map;
/**
* The base class for different stops implementations
*
* @param the input type
* @param the output type
* @see The style specification
*/
public abstract class Stops {
/**
* Convenience method for use in function declarations
*
* @param stops the collections of discrete stops
* @param the Stops input type
* @param the Stops output type
* @return the {@link Stops} implementation
* @see Stop#stop
* @see CategoricalStops
*/
@SafeVarargs
public static CategoricalStops categorical(@NonNull @Size(min = 1) Stop... stops) {
return new CategoricalStops<>(stops);
}
/**
* Convenience method for use in function declarations
*
* @param stops the collections of discrete stops
* @param the Stops input type
* @param the Stops output type
* @return the {@link Stops} implementation
* @see Stop#stop
* @see ExponentialStops
*/
@SafeVarargs
public static ExponentialStops exponential(@NonNull @Size(min = 1) Stop... stops) {
return new ExponentialStops<>(stops);
}
/**
* Convenience method for use in function declarations
*
* @param the Stops input/output type
* @return the {@link IdentityStops} implementation
* @see Stop#stop
* @see IdentityStops
*/
public static IdentityStops identity() {
return new IdentityStops<>();
}
/**
* Convenience method for use in function declarations
*
* @param stops the collections of discrete stops
* @param the Stops input type
* @param the Stops output type
* @return the {@link Stops} implementation
* @see Stop#stop
* @see IntervalStops
*/
@SafeVarargs
public static IntervalStops interval(@NonNull @Size(min = 1) Stop... stops) {
return new IntervalStops<>(stops);
}
/**
* INTERNAL USAGE ONLY
*
* @return the value object representation for conversion to core
*/
@CallSuper
public Map toValueObject() {
HashMap map = new HashMap<>();
map.put("type", getTypeName());
return map;
}
/**
* INTERNAL USAGE ONLY
*
* @return the unique type name as a string according to the style specification
*/
protected abstract String getTypeName();
@Override
public String toString() {
return getTypeName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy