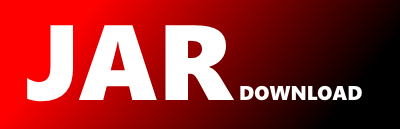
com.mapbox.mapboxsdk.style.layers.SymbolLayer Maven / Gradle / Ivy
// This file is generated. Edit android/platform/scripts/generate-style-code.js, then run `make android-style-code`.
package com.mapbox.mapboxsdk.style.layers;
import android.support.annotation.ColorInt;
import android.support.annotation.NonNull;
import android.support.annotation.UiThread;
import static com.mapbox.mapboxsdk.utils.ColorUtils.rgbaToColor;
/**
* An icon or a text label.
*
* @see The online documentation
*/
@UiThread
public class SymbolLayer extends Layer {
/**
* Creates a SymbolLayer.
*
* @param nativePtr pointer used by core
*/
public SymbolLayer(long nativePtr) {
super(nativePtr);
}
/**
* Creates a SymbolLayer.
*
* @param layerId the id of the layer
* @param sourceId the id of the source
*/
public SymbolLayer(String layerId, String sourceId) {
initialize(layerId, sourceId);
}
protected native void initialize(String layerId, String sourceId);
/**
* Set the source layer.
*
* @param sourceLayer the source layer to set
*/
public void setSourceLayer(String sourceLayer) {
nativeSetSourceLayer(sourceLayer);
}
/**
* Set the source Layer.
*
* @param sourceLayer the source layer to set
* @return This
*/
public SymbolLayer withSourceLayer(String sourceLayer) {
setSourceLayer(sourceLayer);
return this;
}
/**
* Set a single filter.
*
* @param filter the filter to set
*/
public void setFilter(Filter.Statement filter) {
nativeSetFilter(filter.toArray());
}
/**
* Set a single filter.
*
* @param filter the filter to set
* @return This
*/
public SymbolLayer withFilter(Filter.Statement filter) {
setFilter(filter);
return this;
}
/**
* Set a property or properties.
*
* @param properties the var-args properties
* @return This
*/
public SymbolLayer withProperties(@NonNull PropertyValue>... properties) {
setProperties(properties);
return this;
}
// Property getters
/**
* Get the SymbolPlacement property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getSymbolPlacement() {
return (PropertyValue) new PropertyValue("symbol-placement", nativeGetSymbolPlacement());
}
/**
* Get the SymbolSpacing property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getSymbolSpacing() {
return (PropertyValue) new PropertyValue("symbol-spacing", nativeGetSymbolSpacing());
}
/**
* Get the SymbolAvoidEdges property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getSymbolAvoidEdges() {
return (PropertyValue) new PropertyValue("symbol-avoid-edges", nativeGetSymbolAvoidEdges());
}
/**
* Get the IconAllowOverlap property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconAllowOverlap() {
return (PropertyValue) new PropertyValue("icon-allow-overlap", nativeGetIconAllowOverlap());
}
/**
* Get the IconIgnorePlacement property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconIgnorePlacement() {
return (PropertyValue) new PropertyValue("icon-ignore-placement", nativeGetIconIgnorePlacement());
}
/**
* Get the IconOptional property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconOptional() {
return (PropertyValue) new PropertyValue("icon-optional", nativeGetIconOptional());
}
/**
* Get the IconRotationAlignment property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconRotationAlignment() {
return (PropertyValue) new PropertyValue("icon-rotation-alignment", nativeGetIconRotationAlignment());
}
/**
* Get the IconSize property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconSize() {
return (PropertyValue) new PropertyValue("icon-size", nativeGetIconSize());
}
/**
* Get the IconTextFit property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTextFit() {
return (PropertyValue) new PropertyValue("icon-text-fit", nativeGetIconTextFit());
}
/**
* Get the IconTextFitPadding property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTextFitPadding() {
return (PropertyValue) new PropertyValue("icon-text-fit-padding", nativeGetIconTextFitPadding());
}
/**
* Get the IconImage property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconImage() {
return (PropertyValue) new PropertyValue("icon-image", nativeGetIconImage());
}
/**
* Get the IconRotate property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconRotate() {
return (PropertyValue) new PropertyValue("icon-rotate", nativeGetIconRotate());
}
/**
* Get the IconPadding property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconPadding() {
return (PropertyValue) new PropertyValue("icon-padding", nativeGetIconPadding());
}
/**
* Get the IconKeepUpright property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconKeepUpright() {
return (PropertyValue) new PropertyValue("icon-keep-upright", nativeGetIconKeepUpright());
}
/**
* Get the IconOffset property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconOffset() {
return (PropertyValue) new PropertyValue("icon-offset", nativeGetIconOffset());
}
/**
* Get the TextPitchAlignment property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextPitchAlignment() {
return (PropertyValue) new PropertyValue("text-pitch-alignment", nativeGetTextPitchAlignment());
}
/**
* Get the TextRotationAlignment property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextRotationAlignment() {
return (PropertyValue) new PropertyValue("text-rotation-alignment", nativeGetTextRotationAlignment());
}
/**
* Get the TextField property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextField() {
return (PropertyValue) new PropertyValue("text-field", nativeGetTextField());
}
/**
* Get the TextFont property
*
* @return property wrapper value around String[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextFont() {
return (PropertyValue) new PropertyValue("text-font", nativeGetTextFont());
}
/**
* Get the TextSize property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextSize() {
return (PropertyValue) new PropertyValue("text-size", nativeGetTextSize());
}
/**
* Get the TextMaxWidth property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextMaxWidth() {
return (PropertyValue) new PropertyValue("text-max-width", nativeGetTextMaxWidth());
}
/**
* Get the TextLineHeight property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextLineHeight() {
return (PropertyValue) new PropertyValue("text-line-height", nativeGetTextLineHeight());
}
/**
* Get the TextLetterSpacing property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextLetterSpacing() {
return (PropertyValue) new PropertyValue("text-letter-spacing", nativeGetTextLetterSpacing());
}
/**
* Get the TextJustify property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextJustify() {
return (PropertyValue) new PropertyValue("text-justify", nativeGetTextJustify());
}
/**
* Get the TextAnchor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextAnchor() {
return (PropertyValue) new PropertyValue("text-anchor", nativeGetTextAnchor());
}
/**
* Get the TextMaxAngle property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextMaxAngle() {
return (PropertyValue) new PropertyValue("text-max-angle", nativeGetTextMaxAngle());
}
/**
* Get the TextRotate property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextRotate() {
return (PropertyValue) new PropertyValue("text-rotate", nativeGetTextRotate());
}
/**
* Get the TextPadding property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextPadding() {
return (PropertyValue) new PropertyValue("text-padding", nativeGetTextPadding());
}
/**
* Get the TextKeepUpright property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextKeepUpright() {
return (PropertyValue) new PropertyValue("text-keep-upright", nativeGetTextKeepUpright());
}
/**
* Get the TextTransform property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextTransform() {
return (PropertyValue) new PropertyValue("text-transform", nativeGetTextTransform());
}
/**
* Get the TextOffset property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextOffset() {
return (PropertyValue) new PropertyValue("text-offset", nativeGetTextOffset());
}
/**
* Get the TextAllowOverlap property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextAllowOverlap() {
return (PropertyValue) new PropertyValue("text-allow-overlap", nativeGetTextAllowOverlap());
}
/**
* Get the TextIgnorePlacement property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextIgnorePlacement() {
return (PropertyValue) new PropertyValue("text-ignore-placement", nativeGetTextIgnorePlacement());
}
/**
* Get the TextOptional property
*
* @return property wrapper value around Boolean
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextOptional() {
return (PropertyValue) new PropertyValue("text-optional", nativeGetTextOptional());
}
/**
* Get the IconOpacity property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconOpacity() {
return (PropertyValue) new PropertyValue("icon-opacity", nativeGetIconOpacity());
}
/**
* Get the IconColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconColor() {
return (PropertyValue) new PropertyValue("icon-color", nativeGetIconColor());
}
/**
* The color of the icon. This can only be used with sdf icons.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getIconColorAsInt() {
PropertyValue value = getIconColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("icon-color was set as a Function");
}
}
/**
* Get the IconHaloColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconHaloColor() {
return (PropertyValue) new PropertyValue("icon-halo-color", nativeGetIconHaloColor());
}
/**
* The color of the icon's halo. Icon halos can only be used with SDF icons.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getIconHaloColorAsInt() {
PropertyValue value = getIconHaloColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("icon-halo-color was set as a Function");
}
}
/**
* Get the IconHaloWidth property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconHaloWidth() {
return (PropertyValue) new PropertyValue("icon-halo-width", nativeGetIconHaloWidth());
}
/**
* Get the IconHaloBlur property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconHaloBlur() {
return (PropertyValue) new PropertyValue("icon-halo-blur", nativeGetIconHaloBlur());
}
/**
* Get the IconTranslate property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTranslate() {
return (PropertyValue) new PropertyValue("icon-translate", nativeGetIconTranslate());
}
/**
* Get the IconTranslateAnchor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getIconTranslateAnchor() {
return (PropertyValue) new PropertyValue("icon-translate-anchor", nativeGetIconTranslateAnchor());
}
/**
* Get the TextOpacity property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextOpacity() {
return (PropertyValue) new PropertyValue("text-opacity", nativeGetTextOpacity());
}
/**
* Get the TextColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextColor() {
return (PropertyValue) new PropertyValue("text-color", nativeGetTextColor());
}
/**
* The color with which the text will be drawn.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getTextColorAsInt() {
PropertyValue value = getTextColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("text-color was set as a Function");
}
}
/**
* Get the TextHaloColor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextHaloColor() {
return (PropertyValue) new PropertyValue("text-halo-color", nativeGetTextHaloColor());
}
/**
* The color of the text's halo, which helps it stand out from backgrounds.
*
* @return int representation of a rgba string color
* @throws RuntimeException thrown if property isn't a value
*/
@ColorInt
public int getTextHaloColorAsInt() {
PropertyValue value = getTextHaloColor();
if (value.isValue()) {
return rgbaToColor(value.getValue());
} else {
throw new RuntimeException("text-halo-color was set as a Function");
}
}
/**
* Get the TextHaloWidth property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextHaloWidth() {
return (PropertyValue) new PropertyValue("text-halo-width", nativeGetTextHaloWidth());
}
/**
* Get the TextHaloBlur property
*
* @return property wrapper value around Float
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextHaloBlur() {
return (PropertyValue) new PropertyValue("text-halo-blur", nativeGetTextHaloBlur());
}
/**
* Get the TextTranslate property
*
* @return property wrapper value around Float[]
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextTranslate() {
return (PropertyValue) new PropertyValue("text-translate", nativeGetTextTranslate());
}
/**
* Get the TextTranslateAnchor property
*
* @return property wrapper value around String
*/
@SuppressWarnings("unchecked")
public PropertyValue getTextTranslateAnchor() {
return (PropertyValue) new PropertyValue("text-translate-anchor", nativeGetTextTranslateAnchor());
}
private native Object nativeGetSymbolPlacement();
private native Object nativeGetSymbolSpacing();
private native Object nativeGetSymbolAvoidEdges();
private native Object nativeGetIconAllowOverlap();
private native Object nativeGetIconIgnorePlacement();
private native Object nativeGetIconOptional();
private native Object nativeGetIconRotationAlignment();
private native Object nativeGetIconSize();
private native Object nativeGetIconTextFit();
private native Object nativeGetIconTextFitPadding();
private native Object nativeGetIconImage();
private native Object nativeGetIconRotate();
private native Object nativeGetIconPadding();
private native Object nativeGetIconKeepUpright();
private native Object nativeGetIconOffset();
private native Object nativeGetTextPitchAlignment();
private native Object nativeGetTextRotationAlignment();
private native Object nativeGetTextField();
private native Object nativeGetTextFont();
private native Object nativeGetTextSize();
private native Object nativeGetTextMaxWidth();
private native Object nativeGetTextLineHeight();
private native Object nativeGetTextLetterSpacing();
private native Object nativeGetTextJustify();
private native Object nativeGetTextAnchor();
private native Object nativeGetTextMaxAngle();
private native Object nativeGetTextRotate();
private native Object nativeGetTextPadding();
private native Object nativeGetTextKeepUpright();
private native Object nativeGetTextTransform();
private native Object nativeGetTextOffset();
private native Object nativeGetTextAllowOverlap();
private native Object nativeGetTextIgnorePlacement();
private native Object nativeGetTextOptional();
private native Object nativeGetIconOpacity();
private native Object nativeGetIconColor();
private native Object nativeGetIconHaloColor();
private native Object nativeGetIconHaloWidth();
private native Object nativeGetIconHaloBlur();
private native Object nativeGetIconTranslate();
private native Object nativeGetIconTranslateAnchor();
private native Object nativeGetTextOpacity();
private native Object nativeGetTextColor();
private native Object nativeGetTextHaloColor();
private native Object nativeGetTextHaloWidth();
private native Object nativeGetTextHaloBlur();
private native Object nativeGetTextTranslate();
private native Object nativeGetTextTranslateAnchor();
@Override
protected native void finalize() throws Throwable;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy