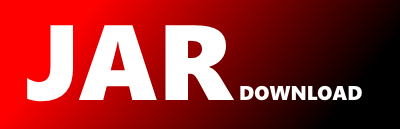
com.marcnuri.yakc.api.securityopenshiftio.v1.SecurityOpenshiftIoV1Api Maven / Gradle / Ivy
Show all versions of openshift Show documentation
/*
* Copyright 2020 Marc Nuri
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.marcnuri.yakc.api.securityopenshiftio.v1;
import com.marcnuri.yakc.api.Api;
import com.marcnuri.yakc.api.KubernetesCall;
import com.marcnuri.yakc.api.KubernetesListCall;
import com.marcnuri.yakc.model.com.github.openshift.api.security.v1.PodSecurityPolicyReview;
import com.marcnuri.yakc.model.com.github.openshift.api.security.v1.PodSecurityPolicySelfSubjectReview;
import com.marcnuri.yakc.model.com.github.openshift.api.security.v1.PodSecurityPolicySubjectReview;
import com.marcnuri.yakc.model.com.github.openshift.api.security.v1.RangeAllocation;
import com.marcnuri.yakc.model.com.github.openshift.api.security.v1.RangeAllocationList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.APIResourceList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.DeleteOptions;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.Status;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.WatchEvent;
import com.marcnuri.yakc.model.io.openshift.security.v1.SecurityContextConstraints;
import com.marcnuri.yakc.model.io.openshift.security.v1.SecurityContextConstraintsList;
import java.util.HashMap;
import retrofit2.http.Body;
import retrofit2.http.HTTP;
import retrofit2.http.Headers;
import retrofit2.http.Path;
import retrofit2.http.QueryMap;
@SuppressWarnings({"squid:S1192", "unused"})
public interface SecurityOpenshiftIoV1Api extends Api {
/**
* get available resources
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/"
)
@Headers({
"Accept: */*"
})
KubernetesCall getAPIResources();
/**
* create a PodSecurityPolicyReview
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/namespaces/{namespace}/podsecuritypolicyreviews",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedPodSecurityPolicyReview(
@Path("namespace") String namespace,
@Body PodSecurityPolicyReview body);
/**
* create a PodSecurityPolicyReview
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/namespaces/{namespace}/podsecuritypolicyreviews",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedPodSecurityPolicyReview(
@Path("namespace") String namespace,
@Body PodSecurityPolicyReview body,
@QueryMap CreateNamespacedPodSecurityPolicyReview queryParameters);
final class CreateNamespacedPodSecurityPolicyReview extends HashMap {
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedPodSecurityPolicyReview dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedPodSecurityPolicyReview fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedPodSecurityPolicyReview pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* create a PodSecurityPolicySelfSubjectReview
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/namespaces/{namespace}/podsecuritypolicyselfsubjectreviews",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedPodSecurityPolicySelfSubjectReview(
@Path("namespace") String namespace,
@Body PodSecurityPolicySelfSubjectReview body);
/**
* create a PodSecurityPolicySelfSubjectReview
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/namespaces/{namespace}/podsecuritypolicyselfsubjectreviews",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedPodSecurityPolicySelfSubjectReview(
@Path("namespace") String namespace,
@Body PodSecurityPolicySelfSubjectReview body,
@QueryMap CreateNamespacedPodSecurityPolicySelfSubjectReview queryParameters);
final class CreateNamespacedPodSecurityPolicySelfSubjectReview extends HashMap {
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedPodSecurityPolicySelfSubjectReview dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedPodSecurityPolicySelfSubjectReview fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedPodSecurityPolicySelfSubjectReview pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* create a PodSecurityPolicySubjectReview
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/namespaces/{namespace}/podsecuritypolicysubjectreviews",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedPodSecurityPolicySubjectReview(
@Path("namespace") String namespace,
@Body PodSecurityPolicySubjectReview body);
/**
* create a PodSecurityPolicySubjectReview
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/namespaces/{namespace}/podsecuritypolicysubjectreviews",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedPodSecurityPolicySubjectReview(
@Path("namespace") String namespace,
@Body PodSecurityPolicySubjectReview body,
@QueryMap CreateNamespacedPodSecurityPolicySubjectReview queryParameters);
final class CreateNamespacedPodSecurityPolicySubjectReview extends HashMap {
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedPodSecurityPolicySubjectReview dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedPodSecurityPolicySubjectReview fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedPodSecurityPolicySubjectReview pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* delete collection of RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionRangeAllocation(
@Body DeleteOptions body);
/**
* delete collection of RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionRangeAllocation();
/**
* delete collection of RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionRangeAllocation(
@Body DeleteOptions body,
@QueryMap DeleteCollectionRangeAllocation queryParameters);
/**
* delete collection of RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionRangeAllocation(
@QueryMap DeleteCollectionRangeAllocation queryParameters);
final class DeleteCollectionRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionRangeAllocation allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionRangeAllocation continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionRangeAllocation dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionRangeAllocation fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionRangeAllocation gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionRangeAllocation labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionRangeAllocation limit(Number limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionRangeAllocation orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionRangeAllocation propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionRangeAllocation resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionRangeAllocation timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionRangeAllocation watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind RangeAllocation
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/rangeallocations"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listRangeAllocation();
/**
* list or watch objects of kind RangeAllocation
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/rangeallocations"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listRangeAllocation(
@QueryMap ListRangeAllocation queryParameters);
final class ListRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListRangeAllocation allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListRangeAllocation continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListRangeAllocation fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListRangeAllocation labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListRangeAllocation limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListRangeAllocation resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListRangeAllocation timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListRangeAllocation watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a RangeAllocation
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/rangeallocations",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createRangeAllocation(
@Body RangeAllocation body);
/**
* create a RangeAllocation
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/rangeallocations",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createRangeAllocation(
@Body RangeAllocation body,
@QueryMap CreateRangeAllocation queryParameters);
final class CreateRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateRangeAllocation dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateRangeAllocation fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteRangeAllocation(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete a RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteRangeAllocation(
@Path("name") String name);
/**
* delete a RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteRangeAllocation(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteRangeAllocation queryParameters);
/**
* delete a RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteRangeAllocation(
@Path("name") String name,
@QueryMap DeleteRangeAllocation queryParameters);
final class DeleteRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteRangeAllocation dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteRangeAllocation gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteRangeAllocation orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteRangeAllocation propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readRangeAllocation(
@Path("name") String name);
/**
* read the specified RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readRangeAllocation(
@Path("name") String name,
@QueryMap ReadRangeAllocation queryParameters);
final class ReadRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadRangeAllocation exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadRangeAllocation export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "PATCH",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchRangeAllocation(
@Path("name") String name,
@Body RangeAllocation body);
/**
* partially update the specified RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "PATCH",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchRangeAllocation(
@Path("name") String name,
@Body RangeAllocation body,
@QueryMap PatchRangeAllocation queryParameters);
final class PatchRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchRangeAllocation dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchRangeAllocation fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchRangeAllocation force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "PUT",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceRangeAllocation(
@Path("name") String name,
@Body RangeAllocation body);
/**
* replace the specified RangeAllocation
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "PUT",
path = "/apis/security.openshift.io/v1/rangeallocations/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceRangeAllocation(
@Path("name") String name,
@Body RangeAllocation body,
@QueryMap ReplaceRangeAllocation queryParameters);
final class ReplaceRangeAllocation extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceRangeAllocation dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceRangeAllocation fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of SecurityContextConstraints
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/securitycontextconstraints"
)
@Headers({
"Accept: */*"
})
KubernetesCall deleteCollectionSecurityContextConstraints();
/**
* delete collection of SecurityContextConstraints
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/securitycontextconstraints"
)
@Headers({
"Accept: */*"
})
KubernetesCall deleteCollectionSecurityContextConstraints(
@QueryMap DeleteCollectionSecurityContextConstraints queryParameters);
final class DeleteCollectionSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionSecurityContextConstraints allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionSecurityContextConstraints continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionSecurityContextConstraints fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionSecurityContextConstraints labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionSecurityContextConstraints limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionSecurityContextConstraints resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionSecurityContextConstraints timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionSecurityContextConstraints watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list objects of kind SecurityContextConstraints
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/securitycontextconstraints"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listSecurityContextConstraints();
/**
* list objects of kind SecurityContextConstraints
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/securitycontextconstraints"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listSecurityContextConstraints(
@QueryMap ListSecurityContextConstraints queryParameters);
final class ListSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListSecurityContextConstraints allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListSecurityContextConstraints continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListSecurityContextConstraints fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListSecurityContextConstraints labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListSecurityContextConstraints limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListSecurityContextConstraints resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListSecurityContextConstraints timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListSecurityContextConstraints watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create SecurityContextConstraints
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/securitycontextconstraints",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createSecurityContextConstraints(
@Body SecurityContextConstraints body);
/**
* create SecurityContextConstraints
*/
@HTTP(
method = "POST",
path = "/apis/security.openshift.io/v1/securitycontextconstraints",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createSecurityContextConstraints(
@Body SecurityContextConstraints body,
@QueryMap CreateSecurityContextConstraints queryParameters);
final class CreateSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateSecurityContextConstraints dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateSecurityContextConstraints fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteSecurityContextConstraints(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteSecurityContextConstraints(
@Path("name") String name);
/**
* delete SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteSecurityContextConstraints(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteSecurityContextConstraints queryParameters);
/**
* delete SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "DELETE",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteSecurityContextConstraints(
@Path("name") String name,
@QueryMap DeleteSecurityContextConstraints queryParameters);
final class DeleteSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteSecurityContextConstraints dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteSecurityContextConstraints gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteSecurityContextConstraints orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteSecurityContextConstraints propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readSecurityContextConstraints(
@Path("name") String name);
/**
* read the specified SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readSecurityContextConstraints(
@Path("name") String name,
@QueryMap ReadSecurityContextConstraints queryParameters);
final class ReadSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ReadSecurityContextConstraints resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
}
/**
* partially update the specified SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "PATCH",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchSecurityContextConstraints(
@Path("name") String name,
@Body SecurityContextConstraints body);
/**
* partially update the specified SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "PATCH",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchSecurityContextConstraints(
@Path("name") String name,
@Body SecurityContextConstraints body,
@QueryMap PatchSecurityContextConstraints queryParameters);
final class PatchSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchSecurityContextConstraints dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public PatchSecurityContextConstraints fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* replace the specified SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "PUT",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceSecurityContextConstraints(
@Path("name") String name,
@Body SecurityContextConstraints body);
/**
* replace the specified SecurityContextConstraints
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "PUT",
path = "/apis/security.openshift.io/v1/securitycontextconstraints/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceSecurityContextConstraints(
@Path("name") String name,
@Body SecurityContextConstraints body,
@QueryMap ReplaceSecurityContextConstraints queryParameters);
final class ReplaceSecurityContextConstraints extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceSecurityContextConstraints dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceSecurityContextConstraints fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* watch individual changes to a list of RangeAllocation. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/rangeallocations"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchRangeAllocationList();
/**
* watch individual changes to a list of RangeAllocation. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/rangeallocations"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchRangeAllocationList(
@QueryMap WatchRangeAllocationList queryParameters);
final class WatchRangeAllocationList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchRangeAllocationList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchRangeAllocationList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchRangeAllocationList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchRangeAllocationList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchRangeAllocationList limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchRangeAllocationList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchRangeAllocationList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchRangeAllocationList timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchRangeAllocationList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind RangeAllocation. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/rangeallocations/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchRangeAllocation(
@Path("name") String name);
/**
* watch changes to an object of kind RangeAllocation. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the RangeAllocation
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/rangeallocations/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchRangeAllocation(
@Path("name") String name,
@QueryMap WatchRangeAllocation queryParameters);
final class WatchRangeAllocation extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchRangeAllocation allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchRangeAllocation continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchRangeAllocation fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchRangeAllocation labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchRangeAllocation limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchRangeAllocation pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchRangeAllocation resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchRangeAllocation timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchRangeAllocation watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of SecurityContextConstraints. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/securitycontextconstraints"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchSecurityContextConstraintsList();
/**
* watch individual changes to a list of SecurityContextConstraints. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/securitycontextconstraints"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchSecurityContextConstraintsList(
@QueryMap WatchSecurityContextConstraintsList queryParameters);
final class WatchSecurityContextConstraintsList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchSecurityContextConstraintsList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchSecurityContextConstraintsList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchSecurityContextConstraintsList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchSecurityContextConstraintsList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchSecurityContextConstraintsList limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchSecurityContextConstraintsList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchSecurityContextConstraintsList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchSecurityContextConstraintsList timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchSecurityContextConstraintsList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind SecurityContextConstraints. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/securitycontextconstraints/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchSecurityContextConstraints(
@Path("name") String name);
/**
* watch changes to an object of kind SecurityContextConstraints. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the SecurityContextConstraints
*/
@HTTP(
method = "GET",
path = "/apis/security.openshift.io/v1/watch/securitycontextconstraints/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchSecurityContextConstraints(
@Path("name") String name,
@QueryMap WatchSecurityContextConstraints queryParameters);
final class WatchSecurityContextConstraints extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchSecurityContextConstraints allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchSecurityContextConstraints continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchSecurityContextConstraints fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchSecurityContextConstraints labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchSecurityContextConstraints limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchSecurityContextConstraints pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchSecurityContextConstraints resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchSecurityContextConstraints timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchSecurityContextConstraints watch(Boolean watch) {
put("watch", watch);
return this;
}
}
}