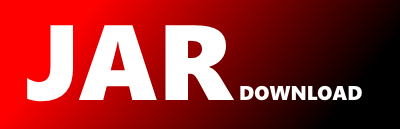
com.marcnuri.yakc.api.apps.v1beta2.AppsV1beta2Api Maven / Gradle / Ivy
Show all versions of kubernetes-api Show documentation
/*
* Copyright 2020 Marc Nuri
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.marcnuri.yakc.api.apps.v1beta2;
import com.marcnuri.yakc.api.Api;
import com.marcnuri.yakc.api.KubernetesCall;
import com.marcnuri.yakc.api.KubernetesListCall;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.ControllerRevision;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.ControllerRevisionList;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.DaemonSet;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.DaemonSetList;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.Deployment;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.DeploymentList;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.ReplicaSet;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.ReplicaSetList;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.Scale;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.StatefulSet;
import com.marcnuri.yakc.model.io.k8s.api.apps.v1beta2.StatefulSetList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.APIResourceList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.DeleteOptions;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.Status;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.WatchEvent;
import java.util.HashMap;
import retrofit2.http.Body;
import retrofit2.http.HTTP;
import retrofit2.http.Headers;
import retrofit2.http.Path;
import retrofit2.http.QueryMap;
@SuppressWarnings({"squid:S1192", "unused"})
public interface AppsV1beta2Api extends Api {
/**
* get available resources
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/"
)
@Headers({
"Accept: */*"
})
KubernetesCall getAPIResources();
/**
* list or watch objects of kind ControllerRevision
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listControllerRevisionForAllNamespaces();
/**
* list or watch objects of kind ControllerRevision
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listControllerRevisionForAllNamespaces(
@QueryMap ListControllerRevisionForAllNamespaces queryParameters);
final class ListControllerRevisionForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListControllerRevisionForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListControllerRevisionForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListControllerRevisionForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListControllerRevisionForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListControllerRevisionForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public ListControllerRevisionForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListControllerRevisionForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListControllerRevisionForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListControllerRevisionForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind DaemonSet
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listDaemonSetForAllNamespaces();
/**
* list or watch objects of kind DaemonSet
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listDaemonSetForAllNamespaces(
@QueryMap ListDaemonSetForAllNamespaces queryParameters);
final class ListDaemonSetForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListDaemonSetForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListDaemonSetForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListDaemonSetForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListDaemonSetForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListDaemonSetForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public ListDaemonSetForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListDaemonSetForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListDaemonSetForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListDaemonSetForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind Deployment
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listDeploymentForAllNamespaces();
/**
* list or watch objects of kind Deployment
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listDeploymentForAllNamespaces(
@QueryMap ListDeploymentForAllNamespaces queryParameters);
final class ListDeploymentForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListDeploymentForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListDeploymentForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListDeploymentForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListDeploymentForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListDeploymentForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public ListDeploymentForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListDeploymentForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListDeploymentForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListDeploymentForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* delete collection of ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedControllerRevision(
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete collection of ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedControllerRevision(
@Path("namespace") String namespace);
/**
* delete collection of ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedControllerRevision(
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteCollectionNamespacedControllerRevision queryParameters);
/**
* delete collection of ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedControllerRevision(
@Path("namespace") String namespace,
@QueryMap DeleteCollectionNamespacedControllerRevision queryParameters);
final class DeleteCollectionNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public DeleteCollectionNamespacedControllerRevision allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionNamespacedControllerRevision continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionNamespacedControllerRevision dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionNamespacedControllerRevision fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionNamespacedControllerRevision gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionNamespacedControllerRevision labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionNamespacedControllerRevision limit(Number limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionNamespacedControllerRevision orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionNamespacedControllerRevision propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionNamespacedControllerRevision resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionNamespacedControllerRevision timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionNamespacedControllerRevision watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedControllerRevision(
@Path("namespace") String namespace);
/**
* list or watch objects of kind ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedControllerRevision(
@Path("namespace") String namespace,
@QueryMap ListNamespacedControllerRevision queryParameters);
final class ListNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListNamespacedControllerRevision allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListNamespacedControllerRevision continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListNamespacedControllerRevision fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListNamespacedControllerRevision labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListNamespacedControllerRevision limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListNamespacedControllerRevision resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListNamespacedControllerRevision timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListNamespacedControllerRevision watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedControllerRevision(
@Path("namespace") String namespace,
@Body ControllerRevision body);
/**
* create a ControllerRevision
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedControllerRevision(
@Path("namespace") String namespace,
@Body ControllerRevision body,
@QueryMap CreateNamespacedControllerRevision queryParameters);
final class CreateNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedControllerRevision dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedControllerRevision fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete a ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* delete a ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteNamespacedControllerRevision queryParameters);
/**
* delete a ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap DeleteNamespacedControllerRevision queryParameters);
final class DeleteNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteNamespacedControllerRevision dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteNamespacedControllerRevision gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteNamespacedControllerRevision orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteNamespacedControllerRevision propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read the specified ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedControllerRevision queryParameters);
final class ReadNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedControllerRevision exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedControllerRevision export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ControllerRevision body);
/**
* partially update the specified ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ControllerRevision body,
@QueryMap PatchNamespacedControllerRevision queryParameters);
final class PatchNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedControllerRevision dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedControllerRevision fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedControllerRevision force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ControllerRevision body);
/**
* replace the specified ControllerRevision
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/controllerrevisions/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ControllerRevision body,
@QueryMap ReplaceNamespacedControllerRevision queryParameters);
final class ReplaceNamespacedControllerRevision extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedControllerRevision dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedControllerRevision fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDaemonSet(
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete collection of DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDaemonSet(
@Path("namespace") String namespace);
/**
* delete collection of DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDaemonSet(
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteCollectionNamespacedDaemonSet queryParameters);
/**
* delete collection of DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDaemonSet(
@Path("namespace") String namespace,
@QueryMap DeleteCollectionNamespacedDaemonSet queryParameters);
final class DeleteCollectionNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public DeleteCollectionNamespacedDaemonSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionNamespacedDaemonSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionNamespacedDaemonSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionNamespacedDaemonSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionNamespacedDaemonSet gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionNamespacedDaemonSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionNamespacedDaemonSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionNamespacedDaemonSet orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionNamespacedDaemonSet propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionNamespacedDaemonSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionNamespacedDaemonSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionNamespacedDaemonSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedDaemonSet(
@Path("namespace") String namespace);
/**
* list or watch objects of kind DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedDaemonSet(
@Path("namespace") String namespace,
@QueryMap ListNamespacedDaemonSet queryParameters);
final class ListNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListNamespacedDaemonSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListNamespacedDaemonSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListNamespacedDaemonSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListNamespacedDaemonSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListNamespacedDaemonSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListNamespacedDaemonSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListNamespacedDaemonSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListNamespacedDaemonSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedDaemonSet(
@Path("namespace") String namespace,
@Body DaemonSet body);
/**
* create a DaemonSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedDaemonSet(
@Path("namespace") String namespace,
@Body DaemonSet body,
@QueryMap CreateNamespacedDaemonSet queryParameters);
final class CreateNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedDaemonSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedDaemonSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete a DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* delete a DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteNamespacedDaemonSet queryParameters);
/**
* delete a DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap DeleteNamespacedDaemonSet queryParameters);
final class DeleteNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteNamespacedDaemonSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteNamespacedDaemonSet gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteNamespacedDaemonSet orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteNamespacedDaemonSet propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedDaemonSet queryParameters);
final class ReadNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedDaemonSet exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedDaemonSet export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body);
/**
* partially update the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body,
@QueryMap PatchNamespacedDaemonSet queryParameters);
final class PatchNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedDaemonSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedDaemonSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedDaemonSet force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body);
/**
* replace the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body,
@QueryMap ReplaceNamespacedDaemonSet queryParameters);
final class ReplaceNamespacedDaemonSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedDaemonSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedDaemonSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read status of the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDaemonSetStatus(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read status of the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDaemonSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedDaemonSetStatus queryParameters);
final class ReadNamespacedDaemonSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedDaemonSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update status of the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDaemonSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body);
/**
* partially update status of the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDaemonSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body,
@QueryMap PatchNamespacedDaemonSetStatus queryParameters);
final class PatchNamespacedDaemonSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedDaemonSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedDaemonSetStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedDaemonSetStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedDaemonSetStatus force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace status of the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDaemonSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body);
/**
* replace status of the specified DaemonSet
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/daemonsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDaemonSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DaemonSet body,
@QueryMap ReplaceNamespacedDaemonSetStatus queryParameters);
final class ReplaceNamespacedDaemonSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedDaemonSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedDaemonSetStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedDaemonSetStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDeployment(
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete collection of Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDeployment(
@Path("namespace") String namespace);
/**
* delete collection of Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDeployment(
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteCollectionNamespacedDeployment queryParameters);
/**
* delete collection of Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedDeployment(
@Path("namespace") String namespace,
@QueryMap DeleteCollectionNamespacedDeployment queryParameters);
final class DeleteCollectionNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public DeleteCollectionNamespacedDeployment allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionNamespacedDeployment continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionNamespacedDeployment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionNamespacedDeployment fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionNamespacedDeployment gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionNamespacedDeployment labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionNamespacedDeployment limit(Number limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionNamespacedDeployment orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionNamespacedDeployment propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionNamespacedDeployment resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionNamespacedDeployment timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionNamespacedDeployment watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedDeployment(
@Path("namespace") String namespace);
/**
* list or watch objects of kind Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedDeployment(
@Path("namespace") String namespace,
@QueryMap ListNamespacedDeployment queryParameters);
final class ListNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListNamespacedDeployment allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListNamespacedDeployment continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListNamespacedDeployment fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListNamespacedDeployment labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListNamespacedDeployment limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListNamespacedDeployment resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListNamespacedDeployment timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListNamespacedDeployment watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedDeployment(
@Path("namespace") String namespace,
@Body Deployment body);
/**
* create a Deployment
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedDeployment(
@Path("namespace") String namespace,
@Body Deployment body,
@QueryMap CreateNamespacedDeployment queryParameters);
final class CreateNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedDeployment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedDeployment fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete a Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* delete a Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteNamespacedDeployment queryParameters);
/**
* delete a Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap DeleteNamespacedDeployment queryParameters);
final class DeleteNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteNamespacedDeployment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteNamespacedDeployment gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteNamespacedDeployment orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteNamespacedDeployment propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedDeployment queryParameters);
final class ReadNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedDeployment exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedDeployment export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body);
/**
* partially update the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body,
@QueryMap PatchNamespacedDeployment queryParameters);
final class PatchNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedDeployment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedDeployment fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedDeployment force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body);
/**
* replace the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDeployment(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body,
@QueryMap ReplaceNamespacedDeployment queryParameters);
final class ReplaceNamespacedDeployment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedDeployment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedDeployment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedDeployment fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read scale of the specified Deployment
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/scale"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDeploymentScale(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read scale of the specified Deployment
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/scale"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDeploymentScale(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedDeploymentScale queryParameters);
final class ReadNamespacedDeploymentScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedDeploymentScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update scale of the specified Deployment
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDeploymentScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body);
/**
* partially update scale of the specified Deployment
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDeploymentScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body,
@QueryMap PatchNamespacedDeploymentScale queryParameters);
final class PatchNamespacedDeploymentScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedDeploymentScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedDeploymentScale dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedDeploymentScale fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedDeploymentScale force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace scale of the specified Deployment
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDeploymentScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body);
/**
* replace scale of the specified Deployment
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDeploymentScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body,
@QueryMap ReplaceNamespacedDeploymentScale queryParameters);
final class ReplaceNamespacedDeploymentScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedDeploymentScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedDeploymentScale dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedDeploymentScale fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read status of the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDeploymentStatus(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read status of the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedDeploymentStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedDeploymentStatus queryParameters);
final class ReadNamespacedDeploymentStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedDeploymentStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update status of the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDeploymentStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body);
/**
* partially update status of the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedDeploymentStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body,
@QueryMap PatchNamespacedDeploymentStatus queryParameters);
final class PatchNamespacedDeploymentStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedDeploymentStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedDeploymentStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedDeploymentStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedDeploymentStatus force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace status of the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDeploymentStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body);
/**
* replace status of the specified Deployment
*
* @param name name of the Deployment
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/deployments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedDeploymentStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Deployment body,
@QueryMap ReplaceNamespacedDeploymentStatus queryParameters);
final class ReplaceNamespacedDeploymentStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedDeploymentStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedDeploymentStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedDeploymentStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedReplicaSet(
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete collection of ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedReplicaSet(
@Path("namespace") String namespace);
/**
* delete collection of ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedReplicaSet(
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteCollectionNamespacedReplicaSet queryParameters);
/**
* delete collection of ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedReplicaSet(
@Path("namespace") String namespace,
@QueryMap DeleteCollectionNamespacedReplicaSet queryParameters);
final class DeleteCollectionNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public DeleteCollectionNamespacedReplicaSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionNamespacedReplicaSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionNamespacedReplicaSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionNamespacedReplicaSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionNamespacedReplicaSet gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionNamespacedReplicaSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionNamespacedReplicaSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionNamespacedReplicaSet orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionNamespacedReplicaSet propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionNamespacedReplicaSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionNamespacedReplicaSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionNamespacedReplicaSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedReplicaSet(
@Path("namespace") String namespace);
/**
* list or watch objects of kind ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedReplicaSet(
@Path("namespace") String namespace,
@QueryMap ListNamespacedReplicaSet queryParameters);
final class ListNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListNamespacedReplicaSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListNamespacedReplicaSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListNamespacedReplicaSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListNamespacedReplicaSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListNamespacedReplicaSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListNamespacedReplicaSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListNamespacedReplicaSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListNamespacedReplicaSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedReplicaSet(
@Path("namespace") String namespace,
@Body ReplicaSet body);
/**
* create a ReplicaSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedReplicaSet(
@Path("namespace") String namespace,
@Body ReplicaSet body,
@QueryMap CreateNamespacedReplicaSet queryParameters);
final class CreateNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedReplicaSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedReplicaSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete a ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* delete a ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteNamespacedReplicaSet queryParameters);
/**
* delete a ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap DeleteNamespacedReplicaSet queryParameters);
final class DeleteNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteNamespacedReplicaSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteNamespacedReplicaSet gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteNamespacedReplicaSet orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteNamespacedReplicaSet propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedReplicaSet queryParameters);
final class ReadNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedReplicaSet exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedReplicaSet export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body);
/**
* partially update the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body,
@QueryMap PatchNamespacedReplicaSet queryParameters);
final class PatchNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedReplicaSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedReplicaSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedReplicaSet force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body);
/**
* replace the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedReplicaSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body,
@QueryMap ReplaceNamespacedReplicaSet queryParameters);
final class ReplaceNamespacedReplicaSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedReplicaSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedReplicaSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedReplicaSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read scale of the specified ReplicaSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/scale"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedReplicaSetScale(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read scale of the specified ReplicaSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/scale"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedReplicaSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedReplicaSetScale queryParameters);
final class ReadNamespacedReplicaSetScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedReplicaSetScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update scale of the specified ReplicaSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedReplicaSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body);
/**
* partially update scale of the specified ReplicaSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedReplicaSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body,
@QueryMap PatchNamespacedReplicaSetScale queryParameters);
final class PatchNamespacedReplicaSetScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedReplicaSetScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedReplicaSetScale dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedReplicaSetScale fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedReplicaSetScale force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace scale of the specified ReplicaSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedReplicaSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body);
/**
* replace scale of the specified ReplicaSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedReplicaSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body,
@QueryMap ReplaceNamespacedReplicaSetScale queryParameters);
final class ReplaceNamespacedReplicaSetScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedReplicaSetScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedReplicaSetScale dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedReplicaSetScale fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read status of the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedReplicaSetStatus(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read status of the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedReplicaSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedReplicaSetStatus queryParameters);
final class ReadNamespacedReplicaSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedReplicaSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update status of the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedReplicaSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body);
/**
* partially update status of the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedReplicaSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body,
@QueryMap PatchNamespacedReplicaSetStatus queryParameters);
final class PatchNamespacedReplicaSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedReplicaSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedReplicaSetStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedReplicaSetStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedReplicaSetStatus force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace status of the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedReplicaSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body);
/**
* replace status of the specified ReplicaSet
*
* @param name name of the ReplicaSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/replicasets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedReplicaSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body ReplicaSet body,
@QueryMap ReplaceNamespacedReplicaSetStatus queryParameters);
final class ReplaceNamespacedReplicaSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedReplicaSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedReplicaSetStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedReplicaSetStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedStatefulSet(
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete collection of StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedStatefulSet(
@Path("namespace") String namespace);
/**
* delete collection of StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedStatefulSet(
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteCollectionNamespacedStatefulSet queryParameters);
/**
* delete collection of StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedStatefulSet(
@Path("namespace") String namespace,
@QueryMap DeleteCollectionNamespacedStatefulSet queryParameters);
final class DeleteCollectionNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public DeleteCollectionNamespacedStatefulSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionNamespacedStatefulSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionNamespacedStatefulSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionNamespacedStatefulSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionNamespacedStatefulSet gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionNamespacedStatefulSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionNamespacedStatefulSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionNamespacedStatefulSet orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionNamespacedStatefulSet propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionNamespacedStatefulSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionNamespacedStatefulSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionNamespacedStatefulSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedStatefulSet(
@Path("namespace") String namespace);
/**
* list or watch objects of kind StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedStatefulSet(
@Path("namespace") String namespace,
@QueryMap ListNamespacedStatefulSet queryParameters);
final class ListNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListNamespacedStatefulSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListNamespacedStatefulSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListNamespacedStatefulSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListNamespacedStatefulSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListNamespacedStatefulSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListNamespacedStatefulSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListNamespacedStatefulSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListNamespacedStatefulSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedStatefulSet(
@Path("namespace") String namespace,
@Body StatefulSet body);
/**
* create a StatefulSet
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedStatefulSet(
@Path("namespace") String namespace,
@Body StatefulSet body,
@QueryMap CreateNamespacedStatefulSet queryParameters);
final class CreateNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedStatefulSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedStatefulSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete a StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* delete a StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteNamespacedStatefulSet queryParameters);
/**
* delete a StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap DeleteNamespacedStatefulSet queryParameters);
final class DeleteNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteNamespacedStatefulSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteNamespacedStatefulSet gracePeriodSeconds(Number gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteNamespacedStatefulSet orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteNamespacedStatefulSet propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedStatefulSet queryParameters);
final class ReadNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedStatefulSet exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedStatefulSet export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body);
/**
* partially update the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body,
@QueryMap PatchNamespacedStatefulSet queryParameters);
final class PatchNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedStatefulSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedStatefulSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedStatefulSet force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body);
/**
* replace the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedStatefulSet(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body,
@QueryMap ReplaceNamespacedStatefulSet queryParameters);
final class ReplaceNamespacedStatefulSet extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedStatefulSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedStatefulSet dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedStatefulSet fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read scale of the specified StatefulSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/scale"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedStatefulSetScale(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read scale of the specified StatefulSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/scale"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedStatefulSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedStatefulSetScale queryParameters);
final class ReadNamespacedStatefulSetScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedStatefulSetScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update scale of the specified StatefulSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedStatefulSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body);
/**
* partially update scale of the specified StatefulSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedStatefulSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body,
@QueryMap PatchNamespacedStatefulSetScale queryParameters);
final class PatchNamespacedStatefulSetScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedStatefulSetScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedStatefulSetScale dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedStatefulSetScale fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedStatefulSetScale force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace scale of the specified StatefulSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedStatefulSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body);
/**
* replace scale of the specified StatefulSet
*
* @param name name of the Scale
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/scale",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedStatefulSetScale(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Scale body,
@QueryMap ReplaceNamespacedStatefulSetScale queryParameters);
final class ReplaceNamespacedStatefulSetScale extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedStatefulSetScale pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedStatefulSetScale dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedStatefulSetScale fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read status of the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedStatefulSetStatus(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read status of the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedStatefulSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedStatefulSetStatus queryParameters);
final class ReadNamespacedStatefulSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedStatefulSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update status of the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedStatefulSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body);
/**
* partially update status of the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedStatefulSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body,
@QueryMap PatchNamespacedStatefulSetStatus queryParameters);
final class PatchNamespacedStatefulSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedStatefulSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedStatefulSetStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedStatefulSetStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedStatefulSetStatus force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace status of the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedStatefulSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body);
/**
* replace status of the specified StatefulSet
*
* @param name name of the StatefulSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/apps/v1beta2/namespaces/{namespace}/statefulsets/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedStatefulSetStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body StatefulSet body,
@QueryMap ReplaceNamespacedStatefulSetStatus queryParameters);
final class ReplaceNamespacedStatefulSetStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedStatefulSetStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedStatefulSetStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedStatefulSetStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* list or watch objects of kind ReplicaSet
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/replicasets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listReplicaSetForAllNamespaces();
/**
* list or watch objects of kind ReplicaSet
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/replicasets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listReplicaSetForAllNamespaces(
@QueryMap ListReplicaSetForAllNamespaces queryParameters);
final class ListReplicaSetForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListReplicaSetForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListReplicaSetForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListReplicaSetForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListReplicaSetForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListReplicaSetForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public ListReplicaSetForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListReplicaSetForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListReplicaSetForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListReplicaSetForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind StatefulSet
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/statefulsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listStatefulSetForAllNamespaces();
/**
* list or watch objects of kind StatefulSet
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/statefulsets"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listStatefulSetForAllNamespaces(
@QueryMap ListStatefulSetForAllNamespaces queryParameters);
final class ListStatefulSetForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public ListStatefulSetForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListStatefulSetForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListStatefulSetForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListStatefulSetForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListStatefulSetForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public ListStatefulSetForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListStatefulSetForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListStatefulSetForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListStatefulSetForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of ControllerRevision. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchControllerRevisionListForAllNamespaces();
/**
* watch individual changes to a list of ControllerRevision. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchControllerRevisionListForAllNamespaces(
@QueryMap WatchControllerRevisionListForAllNamespaces queryParameters);
final class WatchControllerRevisionListForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchControllerRevisionListForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchControllerRevisionListForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchControllerRevisionListForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchControllerRevisionListForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchControllerRevisionListForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchControllerRevisionListForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchControllerRevisionListForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchControllerRevisionListForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchControllerRevisionListForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of DaemonSet. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchDaemonSetListForAllNamespaces();
/**
* watch individual changes to a list of DaemonSet. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchDaemonSetListForAllNamespaces(
@QueryMap WatchDaemonSetListForAllNamespaces queryParameters);
final class WatchDaemonSetListForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchDaemonSetListForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchDaemonSetListForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchDaemonSetListForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchDaemonSetListForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchDaemonSetListForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchDaemonSetListForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchDaemonSetListForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchDaemonSetListForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchDaemonSetListForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of Deployment. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchDeploymentListForAllNamespaces();
/**
* watch individual changes to a list of Deployment. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchDeploymentListForAllNamespaces(
@QueryMap WatchDeploymentListForAllNamespaces queryParameters);
final class WatchDeploymentListForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchDeploymentListForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchDeploymentListForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchDeploymentListForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchDeploymentListForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchDeploymentListForAllNamespaces limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchDeploymentListForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchDeploymentListForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchDeploymentListForAllNamespaces timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchDeploymentListForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of ControllerRevision. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedControllerRevisionList(
@Path("namespace") String namespace);
/**
* watch individual changes to a list of ControllerRevision. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/controllerrevisions"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedControllerRevisionList(
@Path("namespace") String namespace,
@QueryMap WatchNamespacedControllerRevisionList queryParameters);
final class WatchNamespacedControllerRevisionList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchNamespacedControllerRevisionList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchNamespacedControllerRevisionList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchNamespacedControllerRevisionList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchNamespacedControllerRevisionList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchNamespacedControllerRevisionList limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchNamespacedControllerRevisionList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchNamespacedControllerRevisionList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchNamespacedControllerRevisionList timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchNamespacedControllerRevisionList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind ControllerRevision. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/controllerrevisions/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* watch changes to an object of kind ControllerRevision. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the ControllerRevision
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/controllerrevisions/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedControllerRevision(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap WatchNamespacedControllerRevision queryParameters);
final class WatchNamespacedControllerRevision extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchNamespacedControllerRevision allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchNamespacedControllerRevision continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchNamespacedControllerRevision fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchNamespacedControllerRevision labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchNamespacedControllerRevision limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchNamespacedControllerRevision pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchNamespacedControllerRevision resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchNamespacedControllerRevision timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchNamespacedControllerRevision watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of DaemonSet. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedDaemonSetList(
@Path("namespace") String namespace);
/**
* watch individual changes to a list of DaemonSet. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/daemonsets"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedDaemonSetList(
@Path("namespace") String namespace,
@QueryMap WatchNamespacedDaemonSetList queryParameters);
final class WatchNamespacedDaemonSetList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchNamespacedDaemonSetList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchNamespacedDaemonSetList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchNamespacedDaemonSetList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchNamespacedDaemonSetList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchNamespacedDaemonSetList limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchNamespacedDaemonSetList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchNamespacedDaemonSetList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchNamespacedDaemonSetList timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchNamespacedDaemonSetList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind DaemonSet. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/daemonsets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* watch changes to an object of kind DaemonSet. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the DaemonSet
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/daemonsets/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedDaemonSet(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap WatchNamespacedDaemonSet queryParameters);
final class WatchNamespacedDaemonSet extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
This field is alpha and can be changed or removed without notice.
*/
public WatchNamespacedDaemonSet allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchNamespacedDaemonSet continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchNamespacedDaemonSet fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchNamespacedDaemonSet labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchNamespacedDaemonSet limit(Number limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchNamespacedDaemonSet pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchNamespacedDaemonSet resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchNamespacedDaemonSet timeoutSeconds(Number timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchNamespacedDaemonSet watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of Deployment. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedDeploymentList(
@Path("namespace") String namespace);
/**
* watch individual changes to a list of Deployment. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/apps/v1beta2/watch/namespaces/{namespace}/deployments"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedDeploymentList(
@Path("namespace") String namespace,
@QueryMap WatchNamespacedDeploymentList queryParameters);
final class WatchNamespacedDeploymentList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.