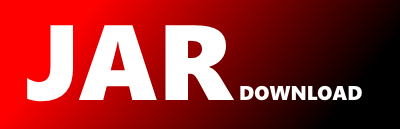
com.marcnuri.yakc.api.networking.v1beta1.NetworkingV1beta1Api Maven / Gradle / Ivy
Show all versions of kubernetes-api Show documentation
/*
* Copyright 2020 Marc Nuri
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.marcnuri.yakc.api.networking.v1beta1;
import com.marcnuri.yakc.api.Api;
import com.marcnuri.yakc.api.KubernetesCall;
import com.marcnuri.yakc.api.KubernetesListCall;
import com.marcnuri.yakc.model.io.k8s.api.networking.v1beta1.Ingress;
import com.marcnuri.yakc.model.io.k8s.api.networking.v1beta1.IngressClass;
import com.marcnuri.yakc.model.io.k8s.api.networking.v1beta1.IngressClassList;
import com.marcnuri.yakc.model.io.k8s.api.networking.v1beta1.IngressList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.APIResourceList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.DeleteOptions;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.Status;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.WatchEvent;
import java.util.HashMap;
import retrofit2.http.Body;
import retrofit2.http.HTTP;
import retrofit2.http.Headers;
import retrofit2.http.Path;
import retrofit2.http.QueryMap;
@SuppressWarnings({"squid:S1192", "unused"})
public interface NetworkingV1beta1Api extends Api {
/**
* get available resources
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/"
)
@Headers({
"Accept: */*"
})
KubernetesCall getAPIResources();
/**
* delete collection of IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionIngressClass(
@Body DeleteOptions body);
/**
* delete collection of IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionIngressClass();
/**
* delete collection of IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionIngressClass(
@Body DeleteOptions body,
@QueryMap DeleteCollectionIngressClass queryParameters);
/**
* delete collection of IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionIngressClass(
@QueryMap DeleteCollectionIngressClass queryParameters);
final class DeleteCollectionIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionIngressClass allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionIngressClass continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionIngressClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionIngressClass fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionIngressClass gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionIngressClass labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionIngressClass limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionIngressClass orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionIngressClass propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionIngressClass resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionIngressClass timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionIngressClass watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind IngressClass
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listIngressClass();
/**
* list or watch objects of kind IngressClass
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listIngressClass(
@QueryMap ListIngressClass queryParameters);
final class ListIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListIngressClass allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListIngressClass continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListIngressClass fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListIngressClass labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListIngressClass limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListIngressClass resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListIngressClass timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListIngressClass watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create an IngressClass
*/
@HTTP(
method = "POST",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createIngressClass(
@Body IngressClass body);
/**
* create an IngressClass
*/
@HTTP(
method = "POST",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createIngressClass(
@Body IngressClass body,
@QueryMap CreateIngressClass queryParameters);
final class CreateIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateIngressClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateIngressClass fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete an IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteIngressClass(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete an IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteIngressClass(
@Path("name") String name);
/**
* delete an IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteIngressClass(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteIngressClass queryParameters);
/**
* delete an IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteIngressClass(
@Path("name") String name,
@QueryMap DeleteIngressClass queryParameters);
final class DeleteIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteIngressClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteIngressClass gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteIngressClass orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteIngressClass propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readIngressClass(
@Path("name") String name);
/**
* read the specified IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readIngressClass(
@Path("name") String name,
@QueryMap ReadIngressClass queryParameters);
final class ReadIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadIngressClass exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadIngressClass export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "PATCH",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchIngressClass(
@Path("name") String name,
@Body IngressClass body);
/**
* partially update the specified IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "PATCH",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchIngressClass(
@Path("name") String name,
@Body IngressClass body,
@QueryMap PatchIngressClass queryParameters);
final class PatchIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchIngressClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchIngressClass fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchIngressClass force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "PUT",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceIngressClass(
@Path("name") String name,
@Body IngressClass body);
/**
* replace the specified IngressClass
*
* @param name name of the IngressClass
*/
@HTTP(
method = "PUT",
path = "/apis/networking.k8s.io/v1beta1/ingressclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceIngressClass(
@Path("name") String name,
@Body IngressClass body,
@QueryMap ReplaceIngressClass queryParameters);
final class ReplaceIngressClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceIngressClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceIngressClass fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* list or watch objects of kind Ingress
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listIngressForAllNamespaces();
/**
* list or watch objects of kind Ingress
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listIngressForAllNamespaces(
@QueryMap ListIngressForAllNamespaces queryParameters);
final class ListIngressForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListIngressForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListIngressForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListIngressForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListIngressForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListIngressForAllNamespaces limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public ListIngressForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListIngressForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListIngressForAllNamespaces timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListIngressForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* delete collection of Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedIngress(
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete collection of Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedIngress(
@Path("namespace") String namespace);
/**
* delete collection of Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedIngress(
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteCollectionNamespacedIngress queryParameters);
/**
* delete collection of Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionNamespacedIngress(
@Path("namespace") String namespace,
@QueryMap DeleteCollectionNamespacedIngress queryParameters);
final class DeleteCollectionNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionNamespacedIngress allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionNamespacedIngress continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionNamespacedIngress dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionNamespacedIngress fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionNamespacedIngress gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionNamespacedIngress labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionNamespacedIngress limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionNamespacedIngress orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionNamespacedIngress propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionNamespacedIngress resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionNamespacedIngress timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionNamespacedIngress watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedIngress(
@Path("namespace") String namespace);
/**
* list or watch objects of kind Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listNamespacedIngress(
@Path("namespace") String namespace,
@QueryMap ListNamespacedIngress queryParameters);
final class ListNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListNamespacedIngress allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListNamespacedIngress continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListNamespacedIngress fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListNamespacedIngress labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListNamespacedIngress limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListNamespacedIngress resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListNamespacedIngress timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListNamespacedIngress watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create an Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedIngress(
@Path("namespace") String namespace,
@Body Ingress body);
/**
* create an Ingress
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "POST",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createNamespacedIngress(
@Path("namespace") String namespace,
@Body Ingress body,
@QueryMap CreateNamespacedIngress queryParameters);
final class CreateNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateNamespacedIngress dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateNamespacedIngress fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete an Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body);
/**
* delete an Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* delete an Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@Body DeleteOptions body,
@QueryMap DeleteNamespacedIngress queryParameters);
/**
* delete an Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "DELETE",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap DeleteNamespacedIngress queryParameters);
final class DeleteNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteNamespacedIngress dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteNamespacedIngress gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteNamespacedIngress orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteNamespacedIngress propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedIngress queryParameters);
final class ReadNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedIngress exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadNamespacedIngress export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body);
/**
* partially update the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body,
@QueryMap PatchNamespacedIngress queryParameters);
final class PatchNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedIngress dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedIngress fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedIngress force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body);
/**
* replace the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body,
@QueryMap ReplaceNamespacedIngress queryParameters);
final class ReplaceNamespacedIngress extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedIngress dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedIngress fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read status of the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedIngressStatus(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* read status of the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readNamespacedIngressStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap ReadNamespacedIngressStatus queryParameters);
final class ReadNamespacedIngressStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadNamespacedIngressStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update status of the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedIngressStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body);
/**
* partially update status of the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PATCH",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchNamespacedIngressStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body,
@QueryMap PatchNamespacedIngressStatus queryParameters);
final class PatchNamespacedIngressStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchNamespacedIngressStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchNamespacedIngressStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchNamespacedIngressStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchNamespacedIngressStatus force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace status of the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedIngressStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body);
/**
* replace status of the specified Ingress
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "PUT",
path = "/apis/networking.k8s.io/v1beta1/namespaces/{namespace}/ingresses/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceNamespacedIngressStatus(
@Path("name") String name,
@Path("namespace") String namespace,
@Body Ingress body,
@QueryMap ReplaceNamespacedIngressStatus queryParameters);
final class ReplaceNamespacedIngressStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceNamespacedIngressStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceNamespacedIngressStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceNamespacedIngressStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* watch individual changes to a list of IngressClass. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/ingressclasses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchIngressClassList();
/**
* watch individual changes to a list of IngressClass. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/ingressclasses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchIngressClassList(
@QueryMap WatchIngressClassList queryParameters);
final class WatchIngressClassList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchIngressClassList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchIngressClassList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchIngressClassList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchIngressClassList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchIngressClassList limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchIngressClassList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchIngressClassList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchIngressClassList timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchIngressClassList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind IngressClass. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the IngressClass
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/ingressclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchIngressClass(
@Path("name") String name);
/**
* watch changes to an object of kind IngressClass. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the IngressClass
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/ingressclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchIngressClass(
@Path("name") String name,
@QueryMap WatchIngressClass queryParameters);
final class WatchIngressClass extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchIngressClass allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchIngressClass continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchIngressClass fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchIngressClass labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchIngressClass limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchIngressClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchIngressClass resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchIngressClass timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchIngressClass watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of Ingress. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchIngressListForAllNamespaces();
/**
* watch individual changes to a list of Ingress. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchIngressListForAllNamespaces(
@QueryMap WatchIngressListForAllNamespaces queryParameters);
final class WatchIngressListForAllNamespaces extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchIngressListForAllNamespaces allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchIngressListForAllNamespaces continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchIngressListForAllNamespaces fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchIngressListForAllNamespaces labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchIngressListForAllNamespaces limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchIngressListForAllNamespaces pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchIngressListForAllNamespaces resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchIngressListForAllNamespaces timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchIngressListForAllNamespaces watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of Ingress. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/namespaces/{namespace}/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedIngressList(
@Path("namespace") String namespace);
/**
* watch individual changes to a list of Ingress. deprecated: use the 'watch' parameter with a list operation instead.
*
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/namespaces/{namespace}/ingresses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedIngressList(
@Path("namespace") String namespace,
@QueryMap WatchNamespacedIngressList queryParameters);
final class WatchNamespacedIngressList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchNamespacedIngressList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchNamespacedIngressList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchNamespacedIngressList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchNamespacedIngressList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchNamespacedIngressList limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchNamespacedIngressList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchNamespacedIngressList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchNamespacedIngressList timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchNamespacedIngressList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind Ingress. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/namespaces/{namespace}/ingresses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace);
/**
* watch changes to an object of kind Ingress. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the Ingress
* @param namespace object name and auth scope, such as for teams and projects
*/
@HTTP(
method = "GET",
path = "/apis/networking.k8s.io/v1beta1/watch/namespaces/{namespace}/ingresses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchNamespacedIngress(
@Path("name") String name,
@Path("namespace") String namespace,
@QueryMap WatchNamespacedIngress queryParameters);
final class WatchNamespacedIngress extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchNamespacedIngress allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchNamespacedIngress continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchNamespacedIngress fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchNamespacedIngress labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchNamespacedIngress limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchNamespacedIngress pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchNamespacedIngress resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchNamespacedIngress timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchNamespacedIngress watch(Boolean watch) {
put("watch", watch);
return this;
}
}
}