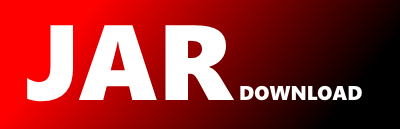
com.marcnuri.yakc.api.storage.v1.StorageV1Api Maven / Gradle / Ivy
Show all versions of kubernetes-api Show documentation
/*
* Copyright 2020 Marc Nuri
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.marcnuri.yakc.api.storage.v1;
import com.marcnuri.yakc.api.Api;
import com.marcnuri.yakc.api.KubernetesCall;
import com.marcnuri.yakc.api.KubernetesListCall;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.CSIDriver;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.CSIDriverList;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.CSINode;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.CSINodeList;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.StorageClass;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.StorageClassList;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.VolumeAttachment;
import com.marcnuri.yakc.model.io.k8s.api.storage.v1.VolumeAttachmentList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.APIResourceList;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.DeleteOptions;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.Status;
import com.marcnuri.yakc.model.io.k8s.apimachinery.pkg.apis.meta.v1.WatchEvent;
import java.util.HashMap;
import retrofit2.http.Body;
import retrofit2.http.HTTP;
import retrofit2.http.Headers;
import retrofit2.http.Path;
import retrofit2.http.QueryMap;
@SuppressWarnings({"squid:S1192", "unused"})
public interface StorageV1Api extends Api {
/**
* get available resources
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/"
)
@Headers({
"Accept: */*"
})
KubernetesCall getAPIResources();
/**
* delete collection of CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSIDriver(
@Body DeleteOptions body);
/**
* delete collection of CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSIDriver();
/**
* delete collection of CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSIDriver(
@Body DeleteOptions body,
@QueryMap DeleteCollectionCSIDriver queryParameters);
/**
* delete collection of CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSIDriver(
@QueryMap DeleteCollectionCSIDriver queryParameters);
final class DeleteCollectionCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionCSIDriver allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionCSIDriver continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionCSIDriver dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionCSIDriver fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionCSIDriver gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionCSIDriver labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionCSIDriver limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionCSIDriver orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionCSIDriver propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionCSIDriver resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionCSIDriver timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionCSIDriver watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind CSIDriver
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csidrivers"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listCSIDriver();
/**
* list or watch objects of kind CSIDriver
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csidrivers"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listCSIDriver(
@QueryMap ListCSIDriver queryParameters);
final class ListCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListCSIDriver allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListCSIDriver continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListCSIDriver fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListCSIDriver labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListCSIDriver limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListCSIDriver resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListCSIDriver timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListCSIDriver watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a CSIDriver
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/csidrivers",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createCSIDriver(
@Body CSIDriver body);
/**
* create a CSIDriver
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/csidrivers",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createCSIDriver(
@Body CSIDriver body,
@QueryMap CreateCSIDriver queryParameters);
final class CreateCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateCSIDriver dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateCSIDriver fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSIDriver(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete a CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSIDriver(
@Path("name") String name);
/**
* delete a CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSIDriver(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteCSIDriver queryParameters);
/**
* delete a CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSIDriver(
@Path("name") String name,
@QueryMap DeleteCSIDriver queryParameters);
final class DeleteCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCSIDriver dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCSIDriver gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCSIDriver orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCSIDriver propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readCSIDriver(
@Path("name") String name);
/**
* read the specified CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readCSIDriver(
@Path("name") String name,
@QueryMap ReadCSIDriver queryParameters);
final class ReadCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadCSIDriver exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadCSIDriver export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchCSIDriver(
@Path("name") String name,
@Body CSIDriver body);
/**
* partially update the specified CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchCSIDriver(
@Path("name") String name,
@Body CSIDriver body,
@QueryMap PatchCSIDriver queryParameters);
final class PatchCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchCSIDriver dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchCSIDriver fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchCSIDriver force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceCSIDriver(
@Path("name") String name,
@Body CSIDriver body);
/**
* replace the specified CSIDriver
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/csidrivers/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceCSIDriver(
@Path("name") String name,
@Body CSIDriver body,
@QueryMap ReplaceCSIDriver queryParameters);
final class ReplaceCSIDriver extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceCSIDriver dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceCSIDriver fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSINode(
@Body DeleteOptions body);
/**
* delete collection of CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSINode();
/**
* delete collection of CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSINode(
@Body DeleteOptions body,
@QueryMap DeleteCollectionCSINode queryParameters);
/**
* delete collection of CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionCSINode(
@QueryMap DeleteCollectionCSINode queryParameters);
final class DeleteCollectionCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionCSINode allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionCSINode continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionCSINode dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionCSINode fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionCSINode gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionCSINode labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionCSINode limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionCSINode orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionCSINode propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionCSINode resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionCSINode timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionCSINode watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind CSINode
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csinodes"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listCSINode();
/**
* list or watch objects of kind CSINode
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csinodes"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listCSINode(
@QueryMap ListCSINode queryParameters);
final class ListCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListCSINode allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListCSINode continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListCSINode fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListCSINode labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListCSINode limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListCSINode resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListCSINode timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListCSINode watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a CSINode
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/csinodes",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createCSINode(
@Body CSINode body);
/**
* create a CSINode
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/csinodes",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createCSINode(
@Body CSINode body,
@QueryMap CreateCSINode queryParameters);
final class CreateCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateCSINode dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateCSINode fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSINode(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete a CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSINode(
@Path("name") String name);
/**
* delete a CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSINode(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteCSINode queryParameters);
/**
* delete a CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCSINode(
@Path("name") String name,
@QueryMap DeleteCSINode queryParameters);
final class DeleteCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCSINode dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCSINode gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCSINode orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCSINode propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csinodes/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readCSINode(
@Path("name") String name);
/**
* read the specified CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/csinodes/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readCSINode(
@Path("name") String name,
@QueryMap ReadCSINode queryParameters);
final class ReadCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadCSINode exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadCSINode export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchCSINode(
@Path("name") String name,
@Body CSINode body);
/**
* partially update the specified CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchCSINode(
@Path("name") String name,
@Body CSINode body,
@QueryMap PatchCSINode queryParameters);
final class PatchCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchCSINode dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchCSINode fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchCSINode force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceCSINode(
@Path("name") String name,
@Body CSINode body);
/**
* replace the specified CSINode
*
* @param name name of the CSINode
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/csinodes/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceCSINode(
@Path("name") String name,
@Body CSINode body,
@QueryMap ReplaceCSINode queryParameters);
final class ReplaceCSINode extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceCSINode dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceCSINode fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionStorageClass(
@Body DeleteOptions body);
/**
* delete collection of StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionStorageClass();
/**
* delete collection of StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionStorageClass(
@Body DeleteOptions body,
@QueryMap DeleteCollectionStorageClass queryParameters);
/**
* delete collection of StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionStorageClass(
@QueryMap DeleteCollectionStorageClass queryParameters);
final class DeleteCollectionStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionStorageClass allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionStorageClass continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionStorageClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionStorageClass fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionStorageClass gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionStorageClass labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionStorageClass limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionStorageClass orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionStorageClass propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionStorageClass resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionStorageClass timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionStorageClass watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind StorageClass
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/storageclasses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listStorageClass();
/**
* list or watch objects of kind StorageClass
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/storageclasses"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listStorageClass(
@QueryMap ListStorageClass queryParameters);
final class ListStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListStorageClass allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListStorageClass continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListStorageClass fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListStorageClass labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListStorageClass limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListStorageClass resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListStorageClass timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListStorageClass watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a StorageClass
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/storageclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createStorageClass(
@Body StorageClass body);
/**
* create a StorageClass
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/storageclasses",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createStorageClass(
@Body StorageClass body,
@QueryMap CreateStorageClass queryParameters);
final class CreateStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateStorageClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateStorageClass fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteStorageClass(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete a StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteStorageClass(
@Path("name") String name);
/**
* delete a StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteStorageClass(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteStorageClass queryParameters);
/**
* delete a StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteStorageClass(
@Path("name") String name,
@QueryMap DeleteStorageClass queryParameters);
final class DeleteStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteStorageClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteStorageClass gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteStorageClass orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteStorageClass propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readStorageClass(
@Path("name") String name);
/**
* read the specified StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readStorageClass(
@Path("name") String name,
@QueryMap ReadStorageClass queryParameters);
final class ReadStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadStorageClass exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadStorageClass export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchStorageClass(
@Path("name") String name,
@Body StorageClass body);
/**
* partially update the specified StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchStorageClass(
@Path("name") String name,
@Body StorageClass body,
@QueryMap PatchStorageClass queryParameters);
final class PatchStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchStorageClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchStorageClass fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchStorageClass force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceStorageClass(
@Path("name") String name,
@Body StorageClass body);
/**
* replace the specified StorageClass
*
* @param name name of the StorageClass
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/storageclasses/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceStorageClass(
@Path("name") String name,
@Body StorageClass body,
@QueryMap ReplaceStorageClass queryParameters);
final class ReplaceStorageClass extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceStorageClass dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceStorageClass fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete collection of VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionVolumeAttachment(
@Body DeleteOptions body);
/**
* delete collection of VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionVolumeAttachment();
/**
* delete collection of VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionVolumeAttachment(
@Body DeleteOptions body,
@QueryMap DeleteCollectionVolumeAttachment queryParameters);
/**
* delete collection of VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteCollectionVolumeAttachment(
@QueryMap DeleteCollectionVolumeAttachment queryParameters);
final class DeleteCollectionVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteCollectionVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public DeleteCollectionVolumeAttachment allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public DeleteCollectionVolumeAttachment continues(String continues) {
put("continue", continues);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteCollectionVolumeAttachment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public DeleteCollectionVolumeAttachment fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteCollectionVolumeAttachment gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public DeleteCollectionVolumeAttachment labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public DeleteCollectionVolumeAttachment limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteCollectionVolumeAttachment orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteCollectionVolumeAttachment propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public DeleteCollectionVolumeAttachment resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public DeleteCollectionVolumeAttachment timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public DeleteCollectionVolumeAttachment watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* list or watch objects of kind VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/volumeattachments"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listVolumeAttachment();
/**
* list or watch objects of kind VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/volumeattachments"
)
@Headers({
"Accept: */*"
})
KubernetesListCall listVolumeAttachment(
@QueryMap ListVolumeAttachment queryParameters);
final class ListVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ListVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public ListVolumeAttachment allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public ListVolumeAttachment continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public ListVolumeAttachment fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public ListVolumeAttachment labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public ListVolumeAttachment limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public ListVolumeAttachment resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public ListVolumeAttachment timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public ListVolumeAttachment watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* create a VolumeAttachment
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/volumeattachments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createVolumeAttachment(
@Body VolumeAttachment body);
/**
* create a VolumeAttachment
*/
@HTTP(
method = "POST",
path = "/apis/storage.k8s.io/v1/volumeattachments",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall createVolumeAttachment(
@Body VolumeAttachment body,
@QueryMap CreateVolumeAttachment queryParameters);
final class CreateVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public CreateVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public CreateVolumeAttachment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public CreateVolumeAttachment fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* delete a VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteVolumeAttachment(
@Path("name") String name,
@Body DeleteOptions body);
/**
* delete a VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteVolumeAttachment(
@Path("name") String name);
/**
* delete a VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteVolumeAttachment(
@Path("name") String name,
@Body DeleteOptions body,
@QueryMap DeleteVolumeAttachment queryParameters);
/**
* delete a VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "DELETE",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall deleteVolumeAttachment(
@Path("name") String name,
@QueryMap DeleteVolumeAttachment queryParameters);
final class DeleteVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public DeleteVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public DeleteVolumeAttachment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* The duration in seconds before the object should be deleted. Value must be non-negative integer. The value zero indicates delete immediately. If this value is nil, the default grace period for the specified type will be used. Defaults to a per object value if not specified. zero means delete immediately.
*/
public DeleteVolumeAttachment gracePeriodSeconds(Integer gracePeriodSeconds) {
put("gracePeriodSeconds", gracePeriodSeconds);
return this;
}
/**
* Deprecated: please use the PropagationPolicy, this field will be deprecated in 1.7. Should the dependent objects be orphaned. If true/false, the "orphan" finalizer will be added to/removed from the object's finalizers list. Either this field or PropagationPolicy may be set, but not both.
*/
public DeleteVolumeAttachment orphanDependents(Boolean orphanDependents) {
put("orphanDependents", orphanDependents);
return this;
}
/**
* Whether and how garbage collection will be performed. Either this field or OrphanDependents may be set, but not both. The default policy is decided by the existing finalizer set in the metadata.finalizers and the resource-specific default policy. Acceptable values are: 'Orphan' - orphan the dependents; 'Background' - allow the garbage collector to delete the dependents in the background; 'Foreground' - a cascading policy that deletes all dependents in the foreground.
*/
public DeleteVolumeAttachment propagationPolicy(String propagationPolicy) {
put("propagationPolicy", propagationPolicy);
return this;
}
}
/**
* read the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readVolumeAttachment(
@Path("name") String name);
/**
* read the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall readVolumeAttachment(
@Path("name") String name,
@QueryMap ReadVolumeAttachment queryParameters);
final class ReadVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* Should the export be exact. Exact export maintains cluster-specific fields like 'Namespace'. Deprecated. Planned for removal in 1.18.
*/
public ReadVolumeAttachment exact(Boolean exact) {
put("exact", exact);
return this;
}
/**
* Should this value be exported. Export strips fields that a user can not specify. Deprecated. Planned for removal in 1.18.
*/
public ReadVolumeAttachment export(Boolean export) {
put("export", export);
return this;
}
}
/**
* partially update the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchVolumeAttachment(
@Path("name") String name,
@Body VolumeAttachment body);
/**
* partially update the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchVolumeAttachment(
@Path("name") String name,
@Body VolumeAttachment body,
@QueryMap PatchVolumeAttachment queryParameters);
final class PatchVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchVolumeAttachment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchVolumeAttachment fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchVolumeAttachment force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceVolumeAttachment(
@Path("name") String name,
@Body VolumeAttachment body);
/**
* replace the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceVolumeAttachment(
@Path("name") String name,
@Body VolumeAttachment body,
@QueryMap ReplaceVolumeAttachment queryParameters);
final class ReplaceVolumeAttachment extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceVolumeAttachment dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceVolumeAttachment fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* read status of the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readVolumeAttachmentStatus(
@Path("name") String name);
/**
* read status of the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}/status"
)
@Headers({
"Accept: */*"
})
KubernetesCall readVolumeAttachmentStatus(
@Path("name") String name,
@QueryMap ReadVolumeAttachmentStatus queryParameters);
final class ReadVolumeAttachmentStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReadVolumeAttachmentStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
}
/**
* partially update status of the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchVolumeAttachmentStatus(
@Path("name") String name,
@Body VolumeAttachment body);
/**
* partially update status of the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PATCH",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/merge-patch+json",
"Accept: */*"
})
KubernetesCall patchVolumeAttachmentStatus(
@Path("name") String name,
@Body VolumeAttachment body,
@QueryMap PatchVolumeAttachmentStatus queryParameters);
final class PatchVolumeAttachmentStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public PatchVolumeAttachmentStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public PatchVolumeAttachmentStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint. This field is required for apply requests (application/apply-patch) but optional for non-apply patch types (JsonPatch, MergePatch, StrategicMergePatch).
*/
public PatchVolumeAttachmentStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
/**
* Force is going to "force" Apply requests. It means user will re-acquire conflicting fields owned by other people. Force flag must be unset for non-apply patch requests.
*/
public PatchVolumeAttachmentStatus force(Boolean force) {
put("force", force);
return this;
}
}
/**
* replace status of the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceVolumeAttachmentStatus(
@Path("name") String name,
@Body VolumeAttachment body);
/**
* replace status of the specified VolumeAttachment
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "PUT",
path = "/apis/storage.k8s.io/v1/volumeattachments/{name}/status",
hasBody = true
)
@Headers({
"Content-Type: application/json",
"Accept: */*"
})
KubernetesCall replaceVolumeAttachmentStatus(
@Path("name") String name,
@Body VolumeAttachment body,
@QueryMap ReplaceVolumeAttachmentStatus queryParameters);
final class ReplaceVolumeAttachmentStatus extends HashMap {
/**
* If 'true', then the output is pretty printed.
*/
public ReplaceVolumeAttachmentStatus pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When present, indicates that modifications should not be persisted. An invalid or unrecognized dryRun directive will result in an error response and no further processing of the request. Valid values are: - All: all dry run stages will be processed
*/
public ReplaceVolumeAttachmentStatus dryRun(String dryRun) {
put("dryRun", dryRun);
return this;
}
/**
* fieldManager is a name associated with the actor or entity that is making these changes. The value must be less than or 128 characters long, and only contain printable characters, as defined by https://golang.org/pkg/unicode/#IsPrint.
*/
public ReplaceVolumeAttachmentStatus fieldManager(String fieldManager) {
put("fieldManager", fieldManager);
return this;
}
}
/**
* watch individual changes to a list of CSIDriver. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csidrivers"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSIDriverList();
/**
* watch individual changes to a list of CSIDriver. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csidrivers"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSIDriverList(
@QueryMap WatchCSIDriverList queryParameters);
final class WatchCSIDriverList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchCSIDriverList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchCSIDriverList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchCSIDriverList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchCSIDriverList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchCSIDriverList limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchCSIDriverList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchCSIDriverList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchCSIDriverList timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchCSIDriverList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind CSIDriver. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csidrivers/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSIDriver(
@Path("name") String name);
/**
* watch changes to an object of kind CSIDriver. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the CSIDriver
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csidrivers/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSIDriver(
@Path("name") String name,
@QueryMap WatchCSIDriver queryParameters);
final class WatchCSIDriver extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchCSIDriver allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchCSIDriver continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchCSIDriver fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchCSIDriver labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchCSIDriver limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchCSIDriver pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchCSIDriver resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchCSIDriver timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchCSIDriver watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of CSINode. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csinodes"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSINodeList();
/**
* watch individual changes to a list of CSINode. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csinodes"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSINodeList(
@QueryMap WatchCSINodeList queryParameters);
final class WatchCSINodeList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchCSINodeList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchCSINodeList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchCSINodeList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchCSINodeList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchCSINodeList limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchCSINodeList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchCSINodeList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchCSINodeList timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchCSINodeList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind CSINode. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the CSINode
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csinodes/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSINode(
@Path("name") String name);
/**
* watch changes to an object of kind CSINode. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the CSINode
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/csinodes/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchCSINode(
@Path("name") String name,
@QueryMap WatchCSINode queryParameters);
final class WatchCSINode extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchCSINode allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchCSINode continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchCSINode fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchCSINode labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchCSINode limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchCSINode pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchCSINode resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchCSINode timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchCSINode watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of StorageClass. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/storageclasses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchStorageClassList();
/**
* watch individual changes to a list of StorageClass. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/storageclasses"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchStorageClassList(
@QueryMap WatchStorageClassList queryParameters);
final class WatchStorageClassList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchStorageClassList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchStorageClassList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchStorageClassList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchStorageClassList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchStorageClassList limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchStorageClassList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchStorageClassList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchStorageClassList timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchStorageClassList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind StorageClass. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the StorageClass
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/storageclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchStorageClass(
@Path("name") String name);
/**
* watch changes to an object of kind StorageClass. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the StorageClass
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/storageclasses/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchStorageClass(
@Path("name") String name,
@QueryMap WatchStorageClass queryParameters);
final class WatchStorageClass extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchStorageClass allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchStorageClass continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchStorageClass fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchStorageClass labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchStorageClass limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchStorageClass pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchStorageClass resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchStorageClass timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchStorageClass watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch individual changes to a list of VolumeAttachment. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/volumeattachments"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchVolumeAttachmentList();
/**
* watch individual changes to a list of VolumeAttachment. deprecated: use the 'watch' parameter with a list operation instead.
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/volumeattachments"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchVolumeAttachmentList(
@QueryMap WatchVolumeAttachmentList queryParameters);
final class WatchVolumeAttachmentList extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchVolumeAttachmentList allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchVolumeAttachmentList continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchVolumeAttachmentList fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchVolumeAttachmentList labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchVolumeAttachmentList limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchVolumeAttachmentList pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchVolumeAttachmentList resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchVolumeAttachmentList timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchVolumeAttachmentList watch(Boolean watch) {
put("watch", watch);
return this;
}
}
/**
* watch changes to an object of kind VolumeAttachment. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/volumeattachments/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchVolumeAttachment(
@Path("name") String name);
/**
* watch changes to an object of kind VolumeAttachment. deprecated: use the 'watch' parameter with a list operation instead, filtered to a single item with the 'fieldSelector' parameter.
*
* @param name name of the VolumeAttachment
*/
@HTTP(
method = "GET",
path = "/apis/storage.k8s.io/v1/watch/volumeattachments/{name}"
)
@Headers({
"Accept: */*"
})
KubernetesCall watchVolumeAttachment(
@Path("name") String name,
@QueryMap WatchVolumeAttachment queryParameters);
final class WatchVolumeAttachment extends HashMap {
/**
* allowWatchBookmarks requests watch events with type "BOOKMARK". Servers that do not implement bookmarks may ignore this flag and bookmarks are sent at the server's discretion. Clients should not assume bookmarks are returned at any specific interval, nor may they assume the server will send any BOOKMARK event during a session. If this is not a watch, this field is ignored. If the feature gate WatchBookmarks is not enabled in apiserver, this field is ignored.
*/
public WatchVolumeAttachment allowWatchBookmarks(Boolean allowWatchBookmarks) {
put("allowWatchBookmarks", allowWatchBookmarks);
return this;
}
/**
* The continue option should be set when retrieving more results from the server. Since this value is server defined, clients may only use the continue value from a previous query result with identical query parameters (except for the value of continue) and the server may reject a continue value it does not recognize. If the specified continue value is no longer valid whether due to expiration (generally five to fifteen minutes) or a configuration change on the server, the server will respond with a 410 ResourceExpired error together with a continue token. If the client needs a consistent list, it must restart their list without the continue field. Otherwise, the client may send another list request with the token received with the 410 error, the server will respond with a list starting from the next key, but from the latest snapshot, which is inconsistent from the previous list results - objects that are created, modified, or deleted after the first list request will be included in the response, as long as their keys are after the "next key".
This field is not supported when watch is true. Clients may start a watch from the last resourceVersion value returned by the server and not miss any modifications.
*/
public WatchVolumeAttachment continues(String continues) {
put("continue", continues);
return this;
}
/**
* A selector to restrict the list of returned objects by their fields. Defaults to everything.
*/
public WatchVolumeAttachment fieldSelector(String fieldSelector) {
put("fieldSelector", fieldSelector);
return this;
}
/**
* A selector to restrict the list of returned objects by their labels. Defaults to everything.
*/
public WatchVolumeAttachment labelSelector(String labelSelector) {
put("labelSelector", labelSelector);
return this;
}
/**
* limit is a maximum number of responses to return for a list call. If more items exist, the server will set the `continue` field on the list metadata to a value that can be used with the same initial query to retrieve the next set of results. Setting a limit may return fewer than the requested amount of items (up to zero items) in the event all requested objects are filtered out and clients should only use the presence of the continue field to determine whether more results are available. Servers may choose not to support the limit argument and will return all of the available results. If limit is specified and the continue field is empty, clients may assume that no more results are available. This field is not supported if watch is true.
The server guarantees that the objects returned when using continue will be identical to issuing a single list call without a limit - that is, no objects created, modified, or deleted after the first request is issued will be included in any subsequent continued requests. This is sometimes referred to as a consistent snapshot, and ensures that a client that is using limit to receive smaller chunks of a very large result can ensure they see all possible objects. If objects are updated during a chunked list the version of the object that was present at the time the first list result was calculated is returned.
*/
public WatchVolumeAttachment limit(Integer limit) {
put("limit", limit);
return this;
}
/**
* If 'true', then the output is pretty printed.
*/
public WatchVolumeAttachment pretty(String pretty) {
put("pretty", pretty);
return this;
}
/**
* When specified with a watch call, shows changes that occur after that particular version of a resource. Defaults to changes from the beginning of history. When specified for list: - if unset, then the result is returned from remote storage based on quorum-read flag; - if it's 0, then we simply return what we currently have in cache, no guarantee; - if set to non zero, then the result is at least as fresh as given rv.
*/
public WatchVolumeAttachment resourceVersion(String resourceVersion) {
put("resourceVersion", resourceVersion);
return this;
}
/**
* Timeout for the list/watch call. This limits the duration of the call, regardless of any activity or inactivity.
*/
public WatchVolumeAttachment timeoutSeconds(Integer timeoutSeconds) {
put("timeoutSeconds", timeoutSeconds);
return this;
}
/**
* Watch for changes to the described resources and return them as a stream of add, update, and remove notifications. Specify resourceVersion.
*/
public WatchVolumeAttachment watch(Boolean watch) {
put("watch", watch);
return this;
}
}
}